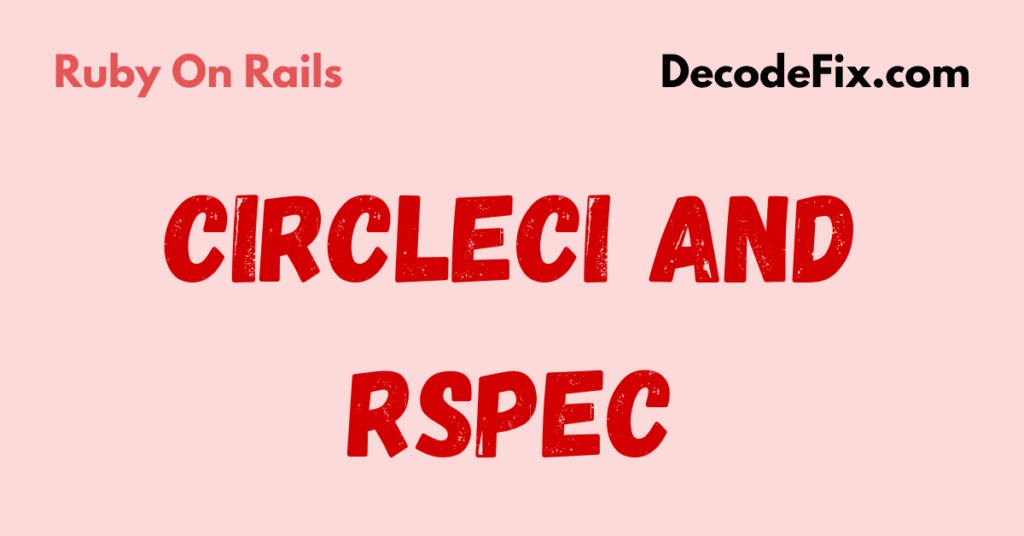
Testing is like flossing for your code—nobody likes to do it, but skipping it leads to serious issues. With CircleCI and RSpec, you can automate the testing process and avoid biting into bugs later. Let’s get down to brass tacks and see how to use CircleCI with RSpec to keep your Ruby projects shiny and bug-free.
What Is CircleCI?
CircleCI is a continuous integration tool. Think of it as a robot that watches your code, checks for mistakes, and helps you deliver faster. It automates testing, deployment, and other repetitive tasks, so developers can focus on what matters: building great software.
For Ruby and Rails projects, CircleCI stands out because it supports Docker, caching, parallelism, and custom configurations. And since we’re talking about RSpec, CircleCI’s integration can run tests in no time, saving you the hassle of doing it manually.
RSpec: Your Testing Companion
RSpec is the bread and butter of testing in the Ruby ecosystem. It’s known for its human-readable syntax and ability to create comprehensive test suites.
Here’s a quick example:
RSpec.describe User, type: :model do
it "validates the presence of a name" do
user = User.new(name: nil)
expect(user.valid?).to be false
end
end
Readable, isn’t it? This simplicity is why RSpec is loved by developers. It doesn’t just test your code; it documents your application’s behavior.
Setting Up RSpec in Your Rails Project
First things first—let’s install RSpec in your Rails app.
Edit your Gemfile:
group :test do
gem 'rspec-rails', '~> 6.0'
end
Then run the setup commands:
bundle install
rails generate rspec:install
You’ll get a folder structure under spec/
for organizing tests. Write your first test in spec/models/user_spec.rb
, as shown above.
With RSpec, tests become a safety net for your app. Run them before deploying changes to catch issues early.
CircleCI and RSpec: A Match Made in Heaven
Now it’s time to link RSpec to CircleCI. First, you’ll need a .circleci/config.yml
file. This is where CircleCI reads instructions for running tests.
Create the file in the root of your project:
version: 2.1
jobs:
test:
docker:
- image: circleci/ruby:3.1-node
steps:
- checkout
- run: bundle install
- run: bundle exec rspec
This basic setup uses a prebuilt Ruby Docker image. When you push code to GitHub, CircleCI runs these steps automatically.
Making It Faster: Optimizing CircleCI for RSpec
Nobody likes waiting for tests to run. Thankfully, CircleCI has tricks up its sleeve.
Caching Dependencies
Reuse gems between builds by adding caching to your config:
steps:
- restore_cache:
keys:
- gem-cache-{{ checksum "Gemfile.lock" }}
- run: bundle install
- save_cache:
paths:
- vendor/bundle
key: gem-cache-{{ checksum "Gemfile.lock" }}
Now, CircleCI will skip downloading gems unless your Gemfile.lock
changes.
Parallelizing Test Runs
Got a huge test suite? Split it across multiple containers to save time:
test:
parallelism: 2
steps:
- run: bundle exec rspec --format RspecJunitFormatter --out test-results/rspec.xml
CircleCI divides tests automatically, so they finish in half the time.
Handling Failures
When a test fails on CircleCI, don’t panic. Check the build logs to see what went wrong. For deeper debugging, CircleCI lets you SSH into the environment where the test ran. Use this to reproduce issues locally.
Real-World Example of CircleCI in Action
Let’s say you’re working on a Rails e-commerce app. You write RSpec tests to check product pricing, inventory updates, and checkout flows. Without automation, you’d run these tests manually after every change—not fun.
With CircleCI, it’s automatic. Push code to GitHub, and CircleCI runs the tests. If a test fails, you’ll get a notification before anyone notices the bug in production.
For example, when a developer accidentally changes the tax calculation:
# Tax calculation should be price * 0.07, but someone wrote:
tax = price * 0.6
RSpec catches it immediately, thanks to your test:
it "calculates tax correctly" do
product = Product.new(price: 100)
expect(product.tax).to eq(7)
end
CircleCI ensures the error never makes it to production.
Alternatives to CircleCI
CircleCI is great, but it’s not the only tool in town. For simpler needs, GitHub Actions can be a good choice. Travis CI is another option, but its popularity has waned recently. If you’re using GitLab, their built-in CI/CD works seamlessly.
Choose CircleCI if you value flexibility and powerful integrations for Ruby projects.
Diving Deeper: Advanced CircleCI and RSpec Techniques
Once you’ve got CircleCI and RSpec set up, you’re ready to level up. Let’s explore some advanced techniques to get the most out of this combo.
Running RSpec in Different Environments
Testing only in development or staging? That’s good, but real-life bugs often creep into production. With CircleCI, you can test across environments easily.
For instance, create different build pipelines for staging and production:
workflows:
version: 2.1
pipeline:
jobs:
- test:
filters:
branches:
only:
- staging
- deploy:
requires:
- test
filters:
branches:
only:
- main
This setup ensures you only deploy after passing tests in staging.
Automating Database Migrations
A Rails app without database migrations is like a car without wheels. It won’t go far. CircleCI can handle migrations for you before running tests.
Update your config file to include migration steps:
steps:
- run: rails db:create
- run: rails db:migrate
CircleCI ensures your test environment matches the latest schema.
Generating Reports with RSpec
RSpec has built-in support for detailed reports, and CircleCI can capture these for you. Use the JUnit formatter to output test results in XML format:
steps:
- run: bundle exec rspec --format RspecJunitFormatter --out test-results/rspec.xml
- store_test_results:
path: test-results
View the test reports directly in CircleCI’s dashboard. This makes it easy to identify failing tests and track performance trends over time.
Testing with Multiple Ruby Versions
Have you ever updated Ruby and realized half your app breaks? Avoid this nightmare by testing with multiple Ruby versions on CircleCI.
Here’s how you do it:
jobs:
test:
docker:
- image: circleci/ruby:2.7-node
- image: circleci/ruby:3.1-node
steps:
- checkout
- run: bundle install
- run: bundle exec rspec
CircleCI will test your app against both Ruby 2.7 and 3.1, so you’ll know if upgrading is safe.
Secrets Management with CircleCI
Storing sensitive data like API keys or database credentials in your code is a bad idea. With CircleCI, you can securely manage secrets using environment variables.
Head to your CircleCI project settings and add environment variables like DATABASE_URL
or AWS_ACCESS_KEY_ID
. Reference them in your .circleci/config.yml
file:
steps:
- run: DATABASE_URL=$DATABASE_URL rails db:migrate
CircleCI keeps your secrets safe while allowing your builds to run smoothly.
Debugging CircleCI Builds
Even with automation, things go wrong. The good news? Debugging in CircleCI is straightforward.
Enable SSH access for a failing build. You’ll get a command to connect to the test environment and inspect what’s causing the issue. For example:
ssh -p 22 user@<circleci-ip-address>
Run failing commands manually to identify the problem, fix it locally, and push the changes to your repo.
Speeding Up Feedback Loops
Waiting for tests can be frustrating. CircleCI’s split tests feature minimizes delays by analyzing past runs and distributing tests more efficiently.
Add this snippet to your config:
steps:
- run: |
circleci tests split --split-by=timings "bundle exec rspec"
The more you run tests, the smarter CircleCI gets at optimizing runtime.
Real-Life Success Stories with CircleCI and RSpec
Companies like Shopify and Zendesk rely on CircleCI and RSpec for testing their Ruby apps. Shopify, for example, processes thousands of transactions per second, and their CI/CD pipeline ensures their app stays rock-solid under heavy traffic.
Let’s imagine a smaller scenario. Say you’re building a Rails API for a ride-hailing app. Your API handles user authentication, ride requests, and payments. Bugs here aren’t just annoying—they’re expensive.
By combining RSpec and CircleCI:
- You write specs to test endpoints like
POST /rides
. - CircleCI runs these tests automatically before deployment.
- If any endpoint fails, the build stops, and you get an alert.
The result? A smooth, error-free app your users trust.
Tips for Writing Better RSpec Tests
Great CI/CD pipelines depend on great tests. Here are some tips to write better RSpec tests:
- Keep tests atomic: Each test should verify one thing.
- Mock external services: Use gems like
WebMock
to simulate APIs. - Avoid hardcoding data: Use factories (e.g., FactoryBot) for test data.
- Focus on edge cases: Bugs often hide where you least expect them.
Example of mocking an external service:
require 'webmock/rspec'
WebMock.stub_request(:get, "https://example.com/api").to_return(status: 200, body: "success")
RSpec.describe "External API" do
it "returns success" do
response = Net::HTTP.get(URI("https://example.com/api"))
expect(response).to eq("success")
end
end
Why CircleCI + RSpec Is a No-Brainer
Let’s face it—manual testing is like riding a bicycle uphill in a thunderstorm. Slow, exhausting, and prone to failure. CircleCI and RSpec automate the grind, giving you confidence and speed.
Whether you’re a solo developer or part of a big team, this duo saves time and prevents headaches. And in today’s fast-paced development world, that’s priceless.
So what are you waiting for? Set up CircleCI and RSpec today, and let automation do the heavy lifting. You’ll thank yourself later!
Conclusion
CircleCI and RSpec are a power duo. Together, they ensure your Ruby apps remain stable, secure, and fast. By automating your tests, you save time, reduce stress, and catch issues before they snowball.
The setup may seem like a chore at first, but trust me—it’s worth every second. Start small, optimize as you go, and watch your productivity soar.
Now, go ahead and set up CircleCI for your next Ruby project. You’ll wonder how you ever lived without it!