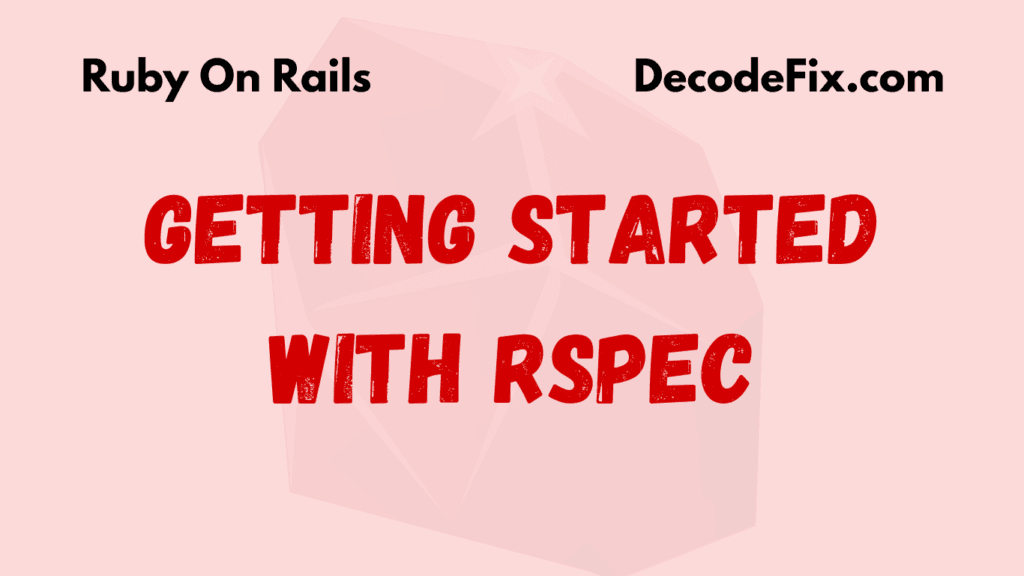
Introduction
RSpec Rails is a widely used testing framework designed to ensure the quality and functionality of Ruby on Rails applications. Unlike traditional manual testing, RSpec provides an automated, systematic approach to testing, helping developers save time and avoid potential bugs.
Why Is RSpec Important in Rails Applications?
- Ensures Code Reliability: Tests written with RSpec act as a safety net, catching bugs early in the development process.
- Enhances Collaboration: RSpec’s human-readable syntax makes it easy for team members to understand test cases.
- Promotes Better Code Design: Writing tests encourage developers to structure their code logically and maintain readability.
Key Benefits of Using RSpec for Rails Apps
- Comprehensive Testing Support: Supports unit, integration, and functional tests.
- Clear Syntax: Intuitive DSL (Domain-Specific Language) makes tests easy to write and read.
- Active Community: The active RSpec community frequently updates the tool and provides extensive resources.
- Flexibility: Works seamlessly with other gems like FactoryBot and Capybara for mocking and integration testing.
For example, e-commerce platforms can use RSpec to verify cart calculations and ensure accurate payment processing.
What is RSpec in Rails?
RSpec is a behavior-driven development (BDD) framework tailored for Ruby applications. It helps developers define application behavior through structured examples, making code validation seamless.
How Does RSpec Differ from Other Testing Tools in Rails?
Feature | RSpec | Minitest |
---|---|---|
Syntax | Human-readable DSL | Standard Ruby code |
Community Support | Extensive | Moderate |
Focus | BDD | TDD |
Flexibility | Highly customizable | Limited plugins |
Why Is RSpec Popular in the Rails Community?
- Readable Syntax: Test cases resemble plain English, improving accessibility for non-technical stakeholders.
- Seamless Rails Integration: RSpec works effortlessly with Rails-specific features like models, views, and controllers.
- Vibrant Ecosystem: Gems like
rspec-rails
extend its capabilities to Rails-specific needs.
💡 Pro Tip: If you’re working with advanced Rails features like callbacks, RSpec provides options to test them efficiently. Learn more about testing callbacks in RSpec.
Setting Up RSpec in a Rails Application
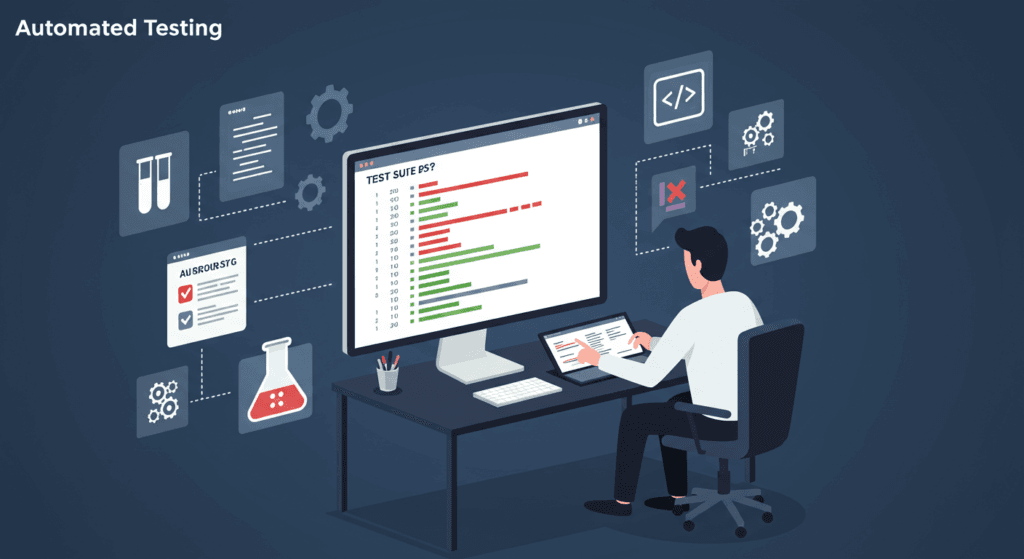
How to Install RSpec Rails
Getting started with RSpec Rails installation is straightforward. Here’s how:
Add the rspec-rails
Gem
Open your Gemfile
and include the following:
group :development, :test do
gem 'rspec-rails', '~> 6.0'
end
Run bundle install
to install the gem.
Run the Installation Generator
Generate the RSpec configuration files using:
rails generate rspec:install
This creates the following files:
.rspec
spec/spec_helper.rb
spec/rails_helper.rb
Verify the Setup
Ensure everything works by running:
rspec --help
Configuring RSpec for Your Application
- Use FactoryBot to mock and seed test data.
- Integrate Capybara for browser-based testing if you have user-facing features.
Common Issues During Setup
- Gem Conflicts: Ensure you’re using compatible versions of Rails and RSpec.
- File Structure Problems: RSpec requires a
spec
directory instead oftest
.
💡 Real-World Example: Many developers use RSpec to test model validations and associations efficiently. Explore how to handle these scenarios in Ruby on Rails Fixtures and Associations.
Understanding the RSpec Structure
The RSpec Rails structure provides an organized way to manage test files and ensure seamless integration with your application.
Overview of the RSpec Directory and File Structure
When you install RSpec in a Rails application, it creates a spec
directory. This folder contains subdirectories and files to organize test cases:
spec/models
: Tests for Rails models.spec/controllers
: Tests for controllers.spec/requests
: Integration tests for HTTP requests.spec/features
: End-to-end tests for user interactions.spec/helpers
: Tests for view helpers.
💡 Pro Tip: Structure your test files similarly to your app files. For instance, if you have a User
model, create spec/models/user_spec.rb
.
Key Components of RSpec Files
Spec Files (*_spec.rb
)
Every test file should follow the naming convention *_spec.rb
. For example: spec/models/user_spec.rb
This naming ensures RSpec can automatically detect and run the file.
Describe Blocks
The describe
block defines the object or method being tested.
describe User do
# test cases here
end
Contexts
Use context
blocks to group-related test scenarios.
context 'when email is invalid' do
# test cases here
end
Examples
Individual test cases are written using it
or specify
.
it 'is valid with valid attributes' do
expect(user.valid?).to be_truthy
end
💡 Real-World Use: Imagine testing a User
model with validations for email and password. Using describe
, context
, and it
, you can write clean, modular tests for each scenario.
Writing Your First Test Case with RSpec
Testing your Rails models with RSpec Rails test examples is straightforward. Here’s how to write and run a basic test for model validations.
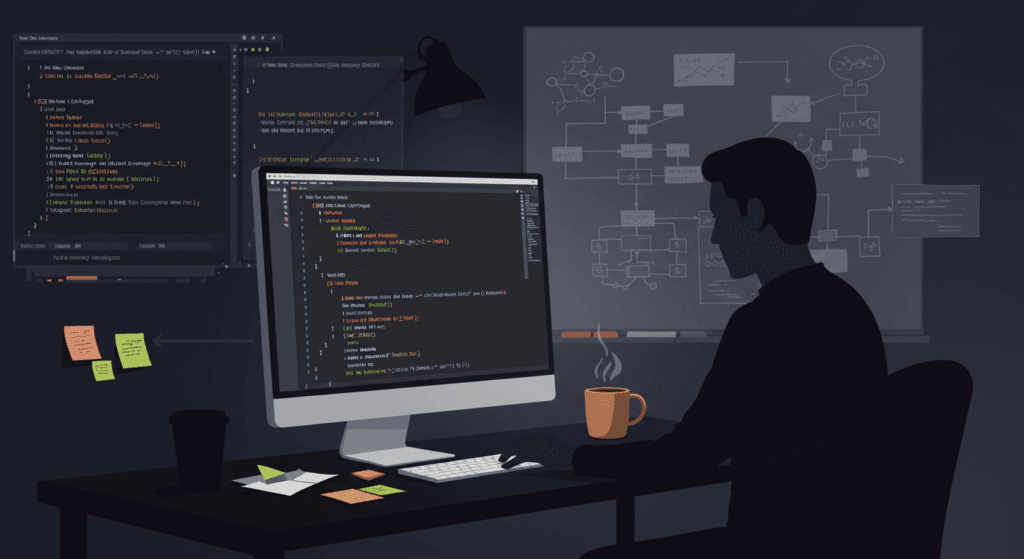
Example: Testing a User Model
Suppose you have a User
model with validations for email
and password
.
Generate the Model
rails generate model User email:string password:string
rake db:migrate
Create the Test File
Create a new file under spec/models
: spec/models/user_spec.rb
Write the Test
require 'rails_helper'
describe User, type: :model do
context 'validations' do
it 'is valid with a valid email and password' do
user = User.new(email: 'test@example.com', password: 'password123')
expect(user).to be_valid
end
it 'is invalid without an email' do
user = User.new(email: nil, password: 'password123')
expect(user).to_not be_valid
end
end
end
Run the Test
Execute the test using:
rspec spec/models/user_spec.rb
Common Matchers in RSpec
Matchers are powerful tools in RSpec for validating test outcomes.
Frequently Used Matchers
- Equality Matchers
eq
: Compares values.expect(5).to eq(5)
eql
: Checks object value and type.
- Inclusion Matchers
include
: Verifies that an element exists in a collection.expect([1, 2, 3]).to include(2)
- Boolean Matchers
be_truthy
andbe_falsey
: Checks for truthy or falsy values.expect(user.valid?).to be_truthy
- Comparison Matchers
be >
: Checks if a value is greater than another.expect(10).to be > 5
Using Matchers in Test Cases
Here’s an example combining multiple matchers:
describe 'Array matchers' do
it 'includes specific elements' do
array = [1, 2, 3]
expect(array).to include(1)
expect(array).to_not include(5)
end
it 'has a specific length' do
array = [1, 2, 3]
expect(array.length).to eq(3)
end
end
💡 Pro Tip: Use custom matchers
for repetitive checks in your tests.
By understanding the RSpec Rails structure, learning to write your first test, and mastering matchers, you’ll streamline your testing process and build robust Rails applications.
For more resources, check out Advanced RSpec Rails Testing Techniques.
Running and Debugging RSpec Tests
Running and debugging RSpec tests in Rails is straightforward but can become a powerful skill with the right approach.
How to Run RSpec Rails Tests
To execute your tests, use the following commands:
- Run all tests:
rspec
- Run a specific directory:
rspec spec/models
- Run a specific file:
rspec spec/models/user_spec.rb
- Run a specific test case:
rspec spec/models/user_spec.rb:12
(The number12
refers to the line where the test case begins.)
💡 Pro Tip: Use the --format documentation
flag for detailed output:
rspec --format documentation
RSpec Debugging Tips
When a test fails, debugging becomes critical. Here are some practical tips:
Use puts
or p
Statements
Insert puts
or p
statements in your code to inspect variables.
it 'checks user email' do
user = User.new(email: 'test@example.com')
puts user.email
expect(user.email).to eq('test@example.com')
end
Focus on Failure Messages
RSpec’s output shows:
- Expected behavior.
- Actual outcome.
Use the binding.pry Gem
Pause execution and interactively debug by adding:
require 'pry'; binding.pry
Run Tests in Isolation
Narrow down failures by running specific test files or lines.
Leverage RSpec’s --backtrace
Option
View the full stack trace for failures:
rspec --backtrace
💡 Pro Tip: Clean up your test environment using rails db:test:prepare
before rerunning tests to ensure consistent results.
Best Practices for Writing RSpec Tests in Rails
Maintaining clean, readable, and reliable test cases ensures long-term success with RSpec Rails.
Tips for Writing Better RSpec Tests
Follow Arrange-Act-Assert (AAA) Pattern
Structure your tests for clarity:
- Arrange: Set up the test.
- Act: Perform the operation.
- Assert: Verify the result.
it 'creates a valid user' do
# Arrange
user = User.new(email: 'test@example.com', password: 'password')
# Act
valid = user.valid?
# Assert
expect(valid).to be_truthy
end
Use Descriptive Test Names
Replace vague names like it "works"
with specific ones:
it 'is valid with a valid email and password'
Avoid Hardcoding Values
Use factories (e.g., FactoryBot) for reusable data:
user = FactoryBot.create(:user)
Write Independent Tests
Ensure tests don’t rely on the state left by other tests.
Test What Matters
Focus on critical functionality rather than over-testing trivial details.
Common Pitfalls to Avoid
- Over-mocking: Mock only external dependencies, not your own app logic.
- Skipping Edge Cases: Always consider negative scenarios.
- Testing Database State: Reset the database between tests to avoid conflicts.
💡 Pro Tip: Use let
and before
blocks wisely to DRY (Don’t Repeat Yourself) your test setup.
Conclusion
RSpec Rails is a cornerstone of reliable Rails applications. Its flexibility, readability, and powerful DSL make it an indispensable tool for developers.
Recap of RSpec’s Benefits
- Ensures code quality through robust testing.
- Provides clear test outputs for debugging.
- Simplifies testing through descriptive syntax and matchers.
What’s Next?
To master RSpec Rails, explore topics like:
- Mocking and stubbing with
RSpec Mocks
. - Advanced matchers and custom matchers.
- Integration testing with
Capybara
.
📩 Invite Readers: Have questions or insights about RSpec? Drop them in the comments on LinkedIn or share your experience to help others!
FAQs About RSpec in Rails
RSpec is a popular testing framework for Ruby and Rails applications. It simplifies writing and running test cases, enabling developers to validate their code efficiently.
RSpec has a more descriptive syntax and extensive community support.
Minitest is faster and part of the Rails core but less feature-rich.
describe
, context
, and it
in RSpec?describe
defines the main subject or functionality being tested.context
specifies conditions or scenarios under which the tests are run.it
contains individual test cases that verify specific behaviors.
You can verify relationships like “belongs to” or “has many” between models by using libraries that simplify association testing, ensuring your database relationships are correctly set up.
Yes, RSpec is well-suited for API testing. It enables you to check endpoints, response statuses, and data structures returned by your API.
Yes, RSpec is versatile and works well with any Ruby project, allowing you to write tests for standalone scripts or libraries.