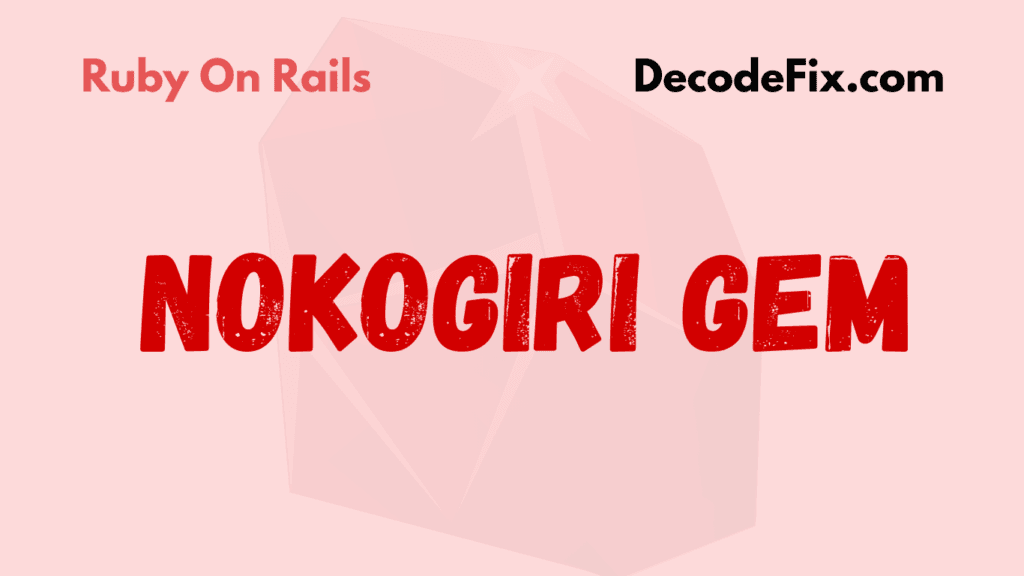
When working with Ruby, one gem frequently mentioned is Nokogiri. Whether you’re building a web scraper, processing XML, or handling complex HTML documents, Nokogiri simplifies these tasks with ease. But what makes it so popular among Ruby developers? Let’s dive in.
What is Nokogiri?
Nokogiri is a Ruby gem that provides an intuitive API for parsing, searching, and manipulating HTML and XML documents. Its name comes from the Japanese word for “saw,” hinting at its precision and sharpness when working with structured documents.
Why is Nokogiri Popular Among Ruby Developers?
Ruby developers love Nokogiri because it simplifies the otherwise tedious tasks of handling XML and HTML. With Nokogiri, you can parse and search documents effortlessly, making it invaluable for web scraping, API integrations, and content processing. Its robust feature set, combined with excellent documentation, ensures that developers of all experience levels can leverage its power.
Key Features of Nokogiri
HTML and XML Parsing
Nokogiri excels at parsing both HTML and XML. It can handle malformed HTML, ensuring your application gracefully processes even poorly formatted documents. Its support for encoding detection ensures compatibility with documents in various character sets.
XPath and CSS Selector Support
Want to extract specific elements from a webpage or XML document? Nokogiri’s support for XPath and CSS selectors makes querying documents both precise and simple. Whether you’re retrieving a single node or a collection of elements, Nokogiri’s tools deliver accurate results.
Schema Validation
Nokogiri supports XML Schema and Document Type Definition (DTD) validation, ensuring your documents comply with specific structural rules. This feature is particularly useful when working with strict XML formats or validating input data.
Performance and Compatibility
Thanks to its C and Java implementations, Nokogiri is fast and efficient. It integrates seamlessly with both CRuby (MRI) and JRuby, making it a versatile choice for various Ruby environments.
Installing Nokogiri in Your Ruby Project
Prerequisites for Installation
Before installing Nokogiri, ensure your environment meets these requirements:
- Ruby (version 2.5 or higher recommended).
- A compatible C compiler (for native extensions).
- Bundler installed for managing gems.
Step-by-Step Installation Guide
- Add Nokogiri to your Gemfile:
gem 'nokogiri'
- Run the following command to install the gem:
bundle install
- Test your installation:
require 'nokogiri'
puts Nokogiri::VERSION
If you see the version number, you’re good to go!
Parsing HTML and XML with Nokogiri
Basic HTML Parsing
Parsing HTML with Nokogiri is straightforward. Here’s a basic example:
require 'nokogiri'
html = '<html><body><h1>Hello, World!</h1></body></html>'
doc = Nokogiri::HTML(html)
puts doc.at_css('h1').text # Output: Hello, World!
This snippet demonstrates how to parse an HTML string and extract the content of an <h1>
tag.
XML Document Handling
Handling XML is just as simple:
xml = '<books><book><title>Ruby Programming</title></book></books>'
doc = Nokogiri::XML(xml)
puts doc.at_xpath('//title').text # Output: Ruby Programming
Nokogiri’s XML parser ensures you can navigate and manipulate structured data easily.
Examples of Parsing Real-World Documents
Consider extracting titles from a blog:
require 'nokogiri'
require 'open-uri'
doc = Nokogiri::HTML(URI.open('https://example.com'))
titles = doc.css('h2.post-title').map(&:text)
puts titles
This example demonstrates how you can extract all post titles using CSS selectors.
Using XPath and CSS Selectors with Nokogiri
When to Use XPath vs. CSS
- XPath is ideal for complex queries or when working with XML namespaces.
- CSS selectors are intuitive and sufficient for most HTML documents.
Examples of Querying Nodes
Using CSS Selectors:
elements = doc.css('.class-name')
elements.each { |el| puts el.text }
Using XPath:
nodes = doc.xpath('//div[@class="class-name"]')
nodes.each { |node| puts node.text }
Both methods provide powerful tools for querying your documents.
Manipulating HTML and XML with Nokogiri
Adding, Removing, or Modifying Elements
Nokogiri makes document manipulation simple:
require 'nokogiri'
doc = Nokogiri::HTML('<div><p>Hello</p></div>')
# Add a new element
doc.at('div').add_child('<p>World</p>')
puts doc.to_html
This example adds a new paragraph to the <div>
element.
Working with Attributes
link = doc.at_css('a')
link['href'] = 'https://new-url.com'
puts doc.to_html
You can easily modify attributes of any element.
Common Use Cases for Nokogiri
Web Scraping
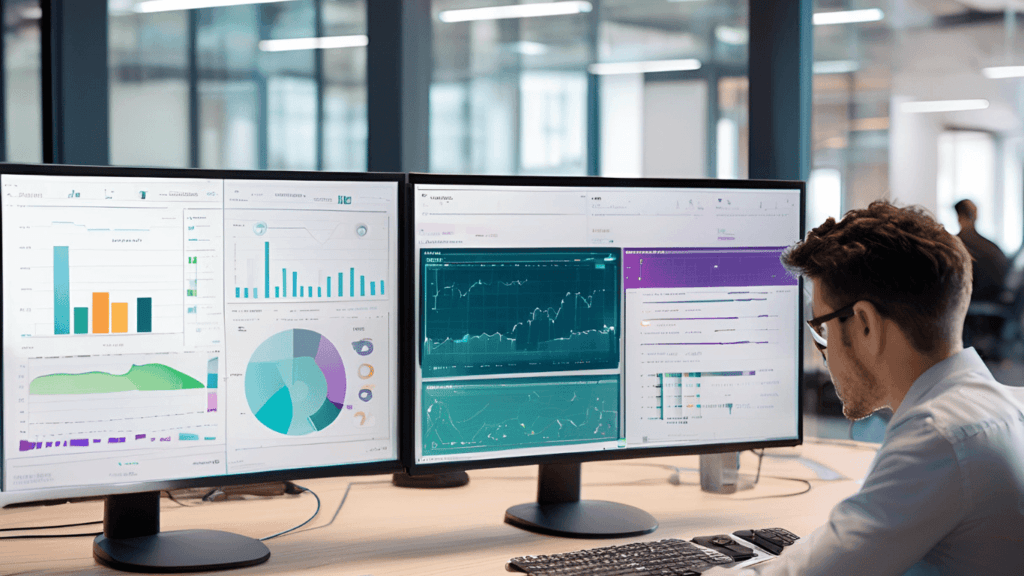
Nokogiri is a popular choice for web scraping. It’s often used to extract data from pages for analysis or automation.
Example:
require 'nokogiri'
require 'open-uri'
doc = Nokogiri::HTML(URI.open('https://news.ycombinator.com'))
links = doc.css('a.storylink').map { |link| { text: link.text, url: link['href'] } }
puts links
This script extracts article titles and URLs from Hacker News.
Data Extraction from XML APIs
Many APIs return XML responses. Nokogiri helps process and extract useful information.
response = '<response><user><name>John Doe</name></user></response>'
doc = Nokogiri::XML(response)
puts doc.at_xpath('//name').text # Output: John Doe
Transforming HTML or XML Content
Nokogiri simplifies transforming structured content:
doc = Nokogiri::HTML('<ul><li>Item 1</li></ul>')
new_li = Nokogiri::XML::Node.new('li', doc)
new_li.content = 'Item 2'
doc.at('ul').add_child(new_li)
puts doc.to_html
This snippet appends a new list item to an unordered list.
Best Practices and Tips for Using Nokogiri
Avoiding Common Pitfalls
Nokogiri is a powerful Ruby gem for parsing HTML and XML, but there are some common mistakes to avoid:
- Not Sanitizing Input: Always sanitize the input before parsing to avoid injecting malicious code. For instance, use libraries like
sanitize
orloofah
when dealing with user-generated content. - Improper XPath or CSS Selectors: Ensure your XPath or CSS queries match the document’s structure. For example:
# Incorrect
doc.xpath('//div[@id="example"]')
# Correct
doc.css('div#example')
- Assuming Document Encoding: Specify the encoding explicitly when parsing non-UTF-8 documents to avoid encoding errors.
html = File.read('example.html', encoding: 'ISO-8859-1')
Nokogiri::HTML(html)
Handling Invalid or Malformed Documents
Web scraping often involves handling poorly structured HTML or XML. Nokogiri has features to manage these issues:
- Use
Nokogiri::HTML5
for Modern HTML: This ensures better handling of malformed HTML. - Recover Mode: For XML, enable the recovery mode to handle errors gracefully:
doc = Nokogiri::XML(xml_string) { |config| config.recover }
- Error Handling: Always wrap parsing in a
begin-rescue
block:
begin
doc = Nokogiri::XML(xml_string)
rescue Nokogiri::XML::SyntaxError => e
puts "Parsing failed: #{e.message}"
end
Integrating Nokogiri with Other Gems and Libraries
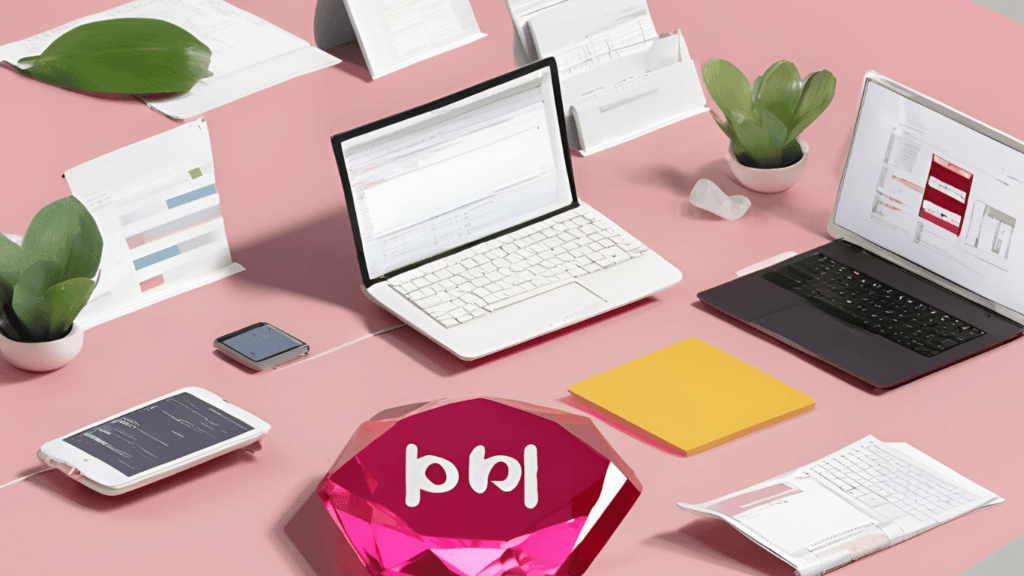
Combining Nokogiri with HTTP Clients
To fetch and parse documents, Nokogiri pairs seamlessly with HTTP libraries:
- HTTParty Example:
require 'httparty'
require 'nokogiri'
response = HTTParty.get('https://example.com')
doc = Nokogiri::HTML(response.body)
puts doc.css('h1').text
- Net::HTTP Example:
require 'net/http'
require 'nokogiri'
uri = URI('https://example.com')
response = Net::HTTP.get(uri)
doc = Nokogiri::HTML(response)
puts doc.title
Nokogiri and Rails
Integrating Nokogiri into Rails applications can simplify tasks like web scraping and data import:
- Extracting Data for Models:
class ScraperService
def self.import_data
url = 'https://example.com/data'
doc = Nokogiri::HTML(HTTParty.get(url).body)
doc.css('.item').each do |item|
Model.create!(name: item.text.strip)
end
end
end
- Using Nokogiri with Active Storage:
html = Rails.application.routes.url_helpers.rails_blob_url(document)
doc = Nokogiri::HTML(html)
For more, Check out the article on must-know 6 libraries of Rails.
Advanced Features of Nokogiri
Working with Namespaces in XML
Namespaces can complicate parsing, but Nokogiri makes them manageable:
- Define Namespace Mapping:
doc = Nokogiri::XML(xml_string)
namespaces = { 'ns' => 'http://example.com/ns' }
doc.xpath('//ns:element', namespaces)
Schema and DTD Validation
Nokogiri supports validating XML against schemas and DTDs:
- Schema Validation:
schema = Nokogiri::XML::Schema(File.read('schema.xsd'))
doc = Nokogiri::XML(File.read('document.xml'))
schema.validate(doc).each do |error|
puts error.message
end
- DTD Validation:
dtd = Nokogiri::XML::DTD(File.read('document.dtd'))
puts dtd.valid?(doc) ? 'Valid' : 'Invalid'
Performance Optimization with Nokogiri
Efficient Parsing Techniques
- Use Specific Selectors: Avoid parsing the entire document if only specific elements are needed.
doc = Nokogiri::HTML(html)
titles = doc.css('h2.title')
- Stream Parsing: For large XML documents, consider using
Nokogiri::XML::Reader
:
reader = Nokogiri::XML::Reader(File.open('large.xml'))
reader.each do |node|
puts node.name if node.node_type == Nokogiri::XML::Reader::TYPE_ELEMENT
end
Managing Large Documents
- Batch Processing: Split large documents into smaller chunks for processing.
- Memory Management: Use tools like
GC.start
to manage memory during heavy processing.
Alternatives to Nokogiri
Other Parsing Libraries for Ruby
- Oga: Lightweight and fast, good for simple HTML/XML parsing.
- REXML: Bundled with Ruby, suitable for basic XML parsing.
When to Use Nokogiri vs. Alternatives
- Use Nokogiri for its rich feature set and performance.
- Opt for lighter libraries when only basic parsing is required.
Troubleshooting and Debugging Nokogiri
Common Errors and Their Fixes
- Encoding Errors: Specify the correct encoding or preprocess the input.
- XPath Errors: Double-check your query syntax and document structure.
Debugging Tools and Techniques
- Enable Logging:
require 'logger'
Nokogiri::XML::Document.logger = Logger.new(STDOUT)
- Visualize Structure:
puts doc.to_xml(indent: 2)
Conclusion and Next Steps
Nokogiri remains a must-have gem for Ruby developers tackling HTML and XML parsing. Its robustness, flexibility, and performance make it indispensable. By adhering to best practices and exploring its advanced features, developers can unlock its full potential.