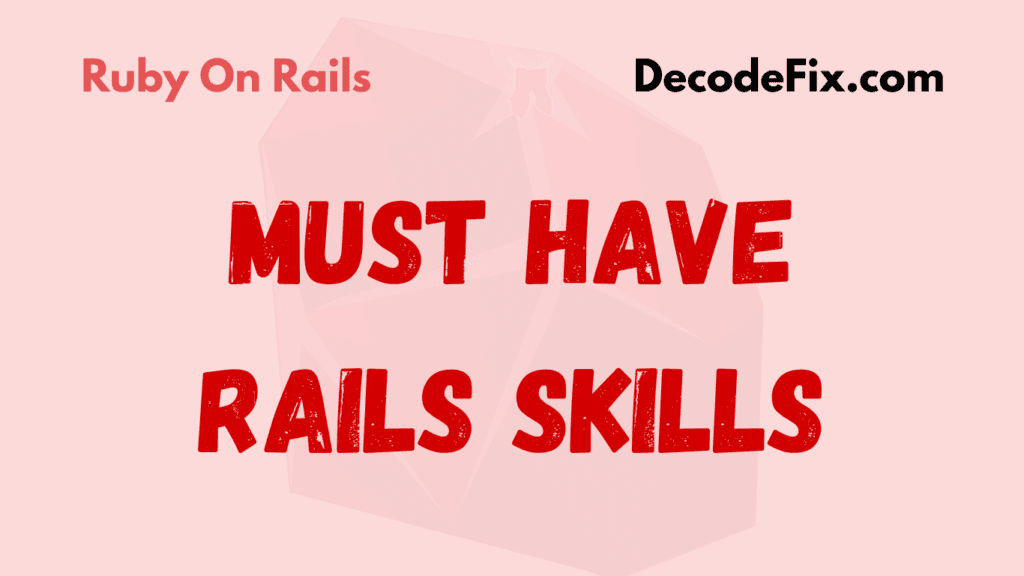
Introduction
Ruby on Rails, often referred to as Rails, is one of the most popular frameworks for building modern, scalable web applications. It powers high-traffic platforms like GitHub, Shopify, and Airbnb. Its developer-friendly nature—thanks to its conventions over configuration and integrated ecosystem—has made it a favorite choice for startups and enterprises alike.
Excelling in Rails isn’t just about knowing its syntax or methods. It requires mastering a unique set of skills that allow developers to build robust, maintainable, and scalable applications. Without these skills, developers may struggle to meet user demands, optimize performance, or deliver secure software.
This guide covers the core skills every Ruby on Rails software developer should have. It focuses on Ruby fundamentals, Rails framework expertise, and practical tips to help you enhance your abilities.
Core Ruby Knowledge
What is Ruby, and why should you master it?
Ruby is the backbone of Rails. To succeed with Rails, you must first become comfortable with Ruby’s syntax and philosophy. Known for being expressive and concise, Ruby allows developers to write code that is both fun and productive.
Key Areas to Master
1. Ruby Syntax
Understand variables, loops, conditionals, methods, and blocks.
For example, instead of writing verbose loops, Ruby allows you to use:[1, 2, 3].each { |n| puts n }
2. Idiomatic Ruby
Learn practices like using symbols over strings where possible:user = { name: :john, age: 30 }
3. Object-Oriented Programming (OOP)
Create classes and modules that encapsulate logic, such as:
class User
attr_accessor :name
def initialize(name)
@name = name
end
end
This is foundational for Rails models and controllers.
What are Ruby Gems, and why are they important?
Gems are reusable libraries that extend Ruby’s functionality. Popular gems like Devise (for authentication) and Pundit (for authorization) make Rails development faster and easier.
Pro Tip: Learn how to build your own gem. It boosts your understanding of Ruby and makes your tools reusable across projects.
What does it mean to write efficient Ruby code?
Efficient Ruby code reduces computational overhead and improves application performance. For example, use map
instead of each
to avoid manually building arrays:names = users.map(&:name)
This approach keeps your code clean and maintainable.
Rails Framework Proficiency
Why is Rails so effective for building web applications?
Rails follows the Model-View-Controller (MVC) architecture, which separates application logic, user interfaces, and data handling.
For example:
- Models define database structure and logic.
- Views handle HTML generation.
- Controllers manage interactions and user actions.
What is Active Record, and why does it matter?
Active Record is Rails’ Object-Relational Mapping (ORM) system. It enables developers to interact with the database using Ruby objects instead of SQL queries.
Examples:
- Retrieving all users:
User.all
- Finding a user by ID:
User.find(1)
Pro Tip: Learn advanced Active Record techniques like eager loading to optimize queries:users = User.includes(:posts).where(active: true)
What are Rails conventions, and how do they help?
Rails emphasizes “convention over configuration” to minimize the time spent on boilerplate code.
Example: Naming a model User
automatically links it to a database table called users
.
Key Best Practices:
- Keep controllers skinny and models fat by delegating most logic to models.
- Use partials to organize repetitive view code.
- Follow Rails’ naming conventions to improve readability and maintainability.
Front-End Development Skills
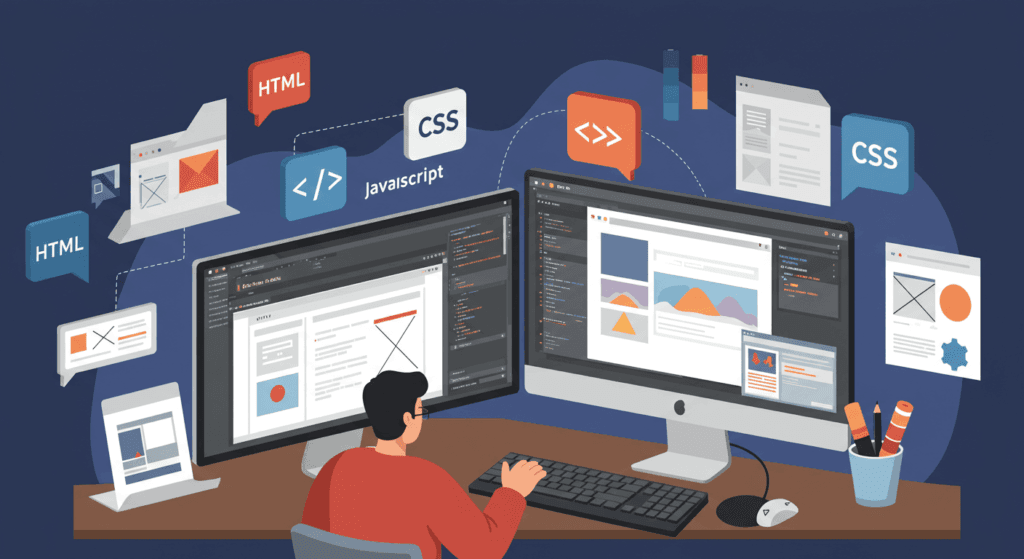
Why are front-end development skills crucial for a Rails developer?
As a Rails developer, it’s not enough to focus only on the back end. A complete understanding of front-end technologies ensures that you can seamlessly integrate user-facing components into your Rails applications, creating an effective and fluid user experience.
What front-end technologies should a Rails developer be familiar with?
Being well-versed in HTML, CSS, and JavaScript is essential. These technologies are foundational for a webpage’s structure, layout, and interactivity.
HTML is the backbone of any web page. Knowing how to structure content using semantic tags ensures accessibility and SEO optimization.
CSS defines the appearance of HTML elements. Knowledge of responsive design and CSS frameworks like Bootstrap helps developers create mobile-friendly, attractive interfaces.
JavaScript is necessary for creating dynamic user experiences. Rails developers should know JavaScript for tasks like form validation, AJAX requests, and animations.
Pro Tip: While HTML and CSS are relatively straightforward, mastering JavaScript’s asynchronous nature can set you apart. Understanding Promises, async/await, and fetch API makes your interactions with the back end much smoother.
Should Rails developers learn modern front-end libraries or frameworks?
Yes. Rails developers often work with front-end frameworks like React, Vue.js, or StimulusJS to build interactive components. These tools enhance user engagement and streamline application behavior.
React is one of the most widely used JavaScript libraries for building dynamic UIs. React allows you to break down complex user interfaces into smaller, reusable components.
Vue.js offers a flexible alternative to React and is known for its simplicity and learning curve. It integrates easily with Rails, and its progressive nature lets you add it incrementally to existing applications.
StimulusJS is designed to work seamlessly with Rails. It’s a modest JavaScript framework that complements Rails’ conventions, allowing for lightweight, powerful behavior in your HTML elements without over-complicating things.
How do you integrate front-end components with Rails applications?
You’ll likely need to pass data from the Rails back-end to the front-end or vice versa. There are several ways to do this:
- Using Rails Views and JavaScript: This is the most straightforward approach, where you embed JavaScript directly into Rails views using
javascript_include_tag
orrails-ujs
for handling asynchronous requests like form submissions. - API Integration: With a growing trend toward decoupled front-end and back-end architectures, many Rails developers create APIs that handle data exchange. Front-end frameworks like React or Vue.js can consume these APIs to manage the display and interaction with data.
Real-world Example: If you were building a blog with Rails and React, you could have Rails serve as the back-end API that sends and receives blog post data (via JSON), while React would be responsible for rendering the blog posts on the front end.
Database Management and Query Optimization
Why is database management a critical skill for Rails developers?
Rails developers must understand how to interact with databases effectively. Whether you’re designing schema, optimizing queries, or maintaining the integrity of data, efficient database management is crucial to the performance of your application.
What should a Rails developer know about SQL and database schema design?
A strong understanding of SQL is foundational. You should be able to write efficient queries to retrieve and manipulate data. This includes JOINs, subqueries, and aggregations.
Pro Tip: While Active Record abstracts many of the SQL details, knowing how SQL works under the hood will make you a more efficient developer. For instance, using the includes
method to load associations in Active Record is a common pattern to prevent N+1 queries. You can also take SQL Quiz to know your skill level.
When designing a database schema, consider normalization (to reduce redundancy) and indexing (to speed up queries). It’s important to strike a balance between normalization and performance.
Real-world Example: If you’re building a Rails e-commerce application, you need to design tables for products, categories, orders, and users. You should know how to relate these tables using foreign keys and how to index frequently queried columns to improve performance.
What databases are essential for Rails developers?
Rails supports multiple database systems, but the most commonly used are PostgreSQL and MySQL. You should have a working knowledge of both.
PostgreSQL is known for its rich set of features, including full-text search, JSON support, and advanced indexing options. It’s a popular choice for Rails applications, particularly when handling complex queries.
MySQL is another widely used option, particularly in legacy systems. It has a simpler structure but is fast and efficient for read-heavy applications.
How can Rails developers optimize queries and handle large datasets efficiently?
When it comes to large datasets, query optimization is key to improving performance. You should focus on:
- Indexing: Make sure frequently searched fields are indexed to speed up query performance.
- Pagination: Use pagination techniques to limit the amount of data returned by a query and avoid performance bottlenecks.
- Eager Loading: Use eager loading in Active Record to preload associations and avoid N+1 query problems.
- Batch Processing: For tasks like importing data or sending emails, avoid loading all data at once. Instead, use batch processing to handle large operations in smaller chunks.
Real-world Example: In a Rails application with a large number of users and posts, if you need to fetch all posts and their associated comments, eager load associations like this:
Post.includes(:comments).where(user_id: current_user.id)
This will reduce the number of database queries, improving speed and efficiency.
Version Control and Collaboration
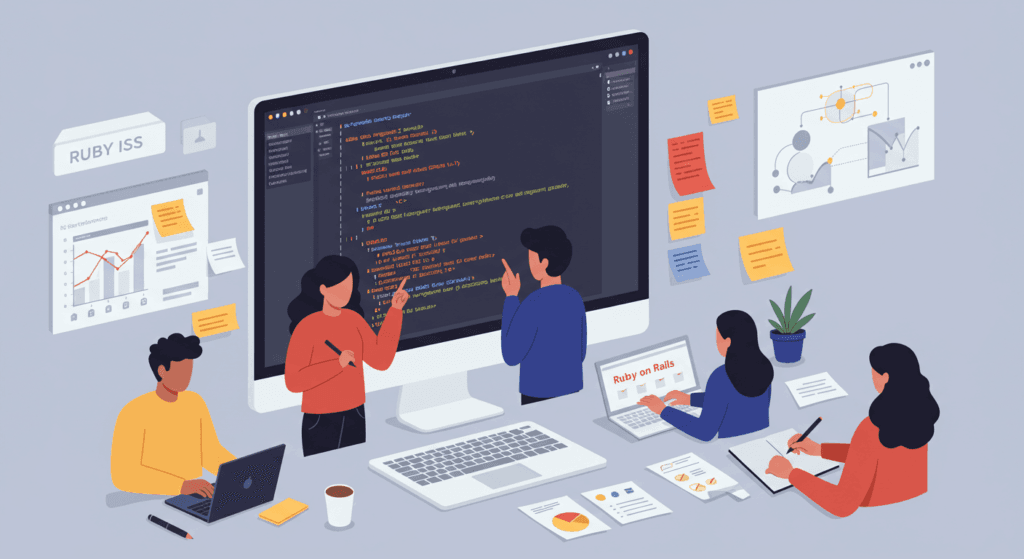
Why is version control important for a Rails developer?
Version control is a critical part of a developer’s workflow. It allows you to track changes, collaborate with others, and maintain the integrity of your codebase. For a Ruby on Rails developer, Git is the most widely used tool for managing code versions and collaborating with other developers. Platforms like GitHub or GitLab provide a place to store your code and offer additional features like pull requests, issue tracking, and code reviews.
What should Rails developers know about Git?
Rails developers must be comfortable using Git for daily tasks. This includes:
- Committing changes: Knowing how to create commits that clearly describe the changes made to the codebase.
- Branching: Working with branches to isolate new features or bug fixes from the main production branch. This ensures the main branch always holds a stable version of the application.
- Merging: Merging branches will incorporate the changes made in feature branches back into the main branch.
- Resolving conflicts: Conflicts are a common issue when multiple developers make changes to the same lines of code. You must know how to resolve conflicts efficiently to avoid delays in your workflow.
Real-world Example: If you’re working on a new feature for an e-commerce application and your colleague is working on a bug fix, you’ll create separate branches (feature/add-to-cart
and bugfix/login-error
). After both branches are ready, you’ll merge them into the main branch. If there are any conflicts in the files, you’ll resolve them before merging to avoid breaking the code.
How does collaboration work in a team environment?
In a team setting, collaboration is crucial to ensure smooth development. Familiarity with Agile methodologies like Scrum or Kanban can improve team coordination. Rails developers work with Product Owners, designers, and other developers to plan sprints and prioritize tasks.
Tools like GitHub or GitLab offer features that facilitate collaboration:
- Pull requests allow team members to review and discuss changes before they are merged into the main codebase.
- Code reviews ensure that best practices are followed and bugs are caught early.
- Issue tracking helps teams keep track of bugs, features, and improvements.
Pro Tip: Make sure to write clear, concise commit messages. They serve as documentation for your team and can save time when you need to understand the history of changes made to the codebase.
Testing and Debugging
Why is testing essential for Rails development?
Testing ensures that your code behaves as expected and helps you catch bugs early. It’s a cornerstone of maintaining high-quality, maintainable applications. Rails developers should have a strong understanding of both automated testing and debugging practices.
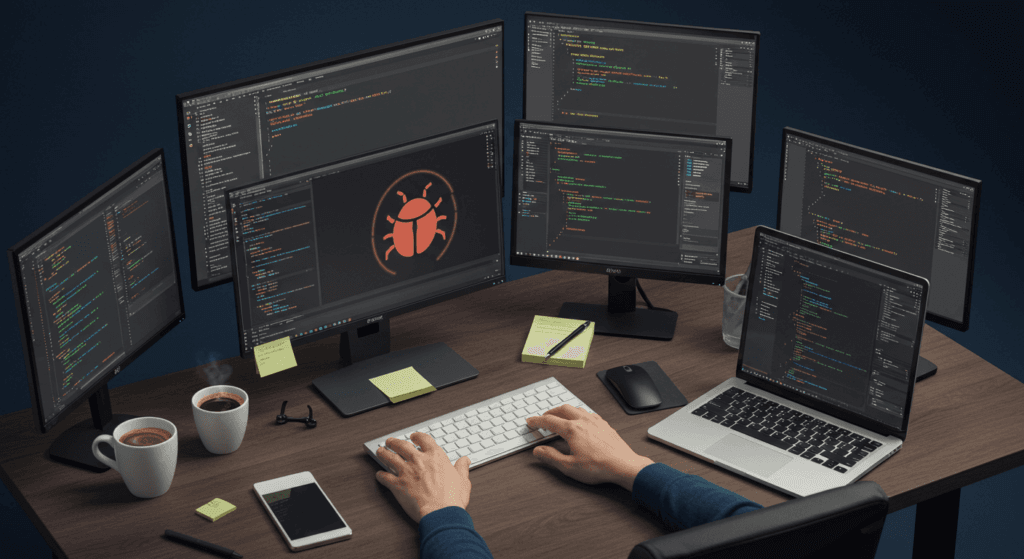
What testing frameworks should Rails developers use?
Rails supports several testing frameworks, but RSpec and Minitest are the most widely used.
- RSpec: This is a popular testing framework for Ruby on Rails, known for its readable syntax. It allows you to write unit tests, integration tests, performance tests, and feature tests with ease.
- Minitest: Minitest is the default testing framework for Rails and provides a lightweight, fast alternative to RSpec. It supports unit testing, mocking, and assertions.
What is test-driven development (TDD), and should Rails developers use it?
Test-driven development (TDD) is a development approach where you write tests before writing the actual code. TDD ensures that your code is thoroughly tested and meets the requirements set by your team. It encourages writing modular, maintainable code by focusing on small, manageable chunks.
Rails developers should consider practicing TDD as it leads to cleaner code, better test coverage, and fewer bugs in the long run.
Real-world Example: Let’s say you’re building a feature that allows users to rate products in your Rails application. In TDD, you’d first write a test that checks if a user can submit a rating. Then, you’d write the minimum amount of code to pass the test. After that, you’d refactor and improve the code, all while ensuring your tests pass.
How do you debug Rails applications?
Even with automated tests in place, debugging remains an essential skill for Rails developers. Common debugging tools and techniques include:
- Byebug: A powerful debugging tool that allows you to pause the execution of your code at any point and inspect variables, step through code, and understand what’s going wrong.
- Rails Logger: Rails has built-in logging functionality that outputs helpful information to the console, such as the SQL queries generated, request parameters, and error messages.
- Stack Traces: When errors occur, Rails provides a stack trace that can help you identify where the error originated in your application. Understanding how to read and use stack traces will save time when fixing bugs.
Pro Tip: If you’re unsure about the state of a variable or object, don’t hesitate to use byebug
it to inspect its value in real-time. It’s a quick and effective way to pinpoint issues.
Deployment and DevOps Basics
Why is deployment knowledge important for a Rails developer?
As a Rails developer, you need to ensure your application is deployed smoothly and reliably. Deployment involves making your application available for users, and understanding DevOps principles can streamline this process.
What deployment tools should Rails developers know?
There are several tools available for deploying Rails applications, with some of the most common being Capistrano and Heroku CLI.
- Capistrano: This tool automates the process of deploying Rails applications to servers. Capistrano helps manage tasks like code updates, asset precompilation, and server restarts.
- Heroku CLI: Heroku is a popular Platform-as-a-Service (PaaS) that simplifies Rails deployment. The Heroku CLI lets developers deploy, manage, and scale applications directly from the command line.
Real-world Example: If you’re using Heroku, deployment could be as simple as pushing your code to a Git repository with git push heroku main
. Heroku handles all of the infrastructure management for you. On the other hand, if you’re using Capistrano, you might need to configure your server and set up tasks manually.
What DevOps skills should Rails developers have?
DevOps involves automating and streamlining development, testing, and deployment processes. Rails developers should have some knowledge of tools and practices like:
- Docker: Containerization allows you to package your application and its dependencies into a single, portable container that can be deployed anywhere.
- Kubernetes: A container orchestration tool that automates the deployment, scaling, and management of containerized applications.
- AWS (Amazon Web Services): Many Rails applications are hosted on AWS. Familiarity with EC2, RDS, and S3 allows developers to set up scalable infrastructure for their applications.
What is a CI/CD pipeline, and why is it important?
A CI/CD pipeline (Continuous Integration/Continuous Deployment) automates the process of testing, building, and deploying code. Setting up a CI/CD pipeline ensures that code is automatically tested and deployed without manual intervention, reducing human error and speeding up the deployment process. Popular CI/CD tools include CircleCI, Travis CI, and GitHub Actions.
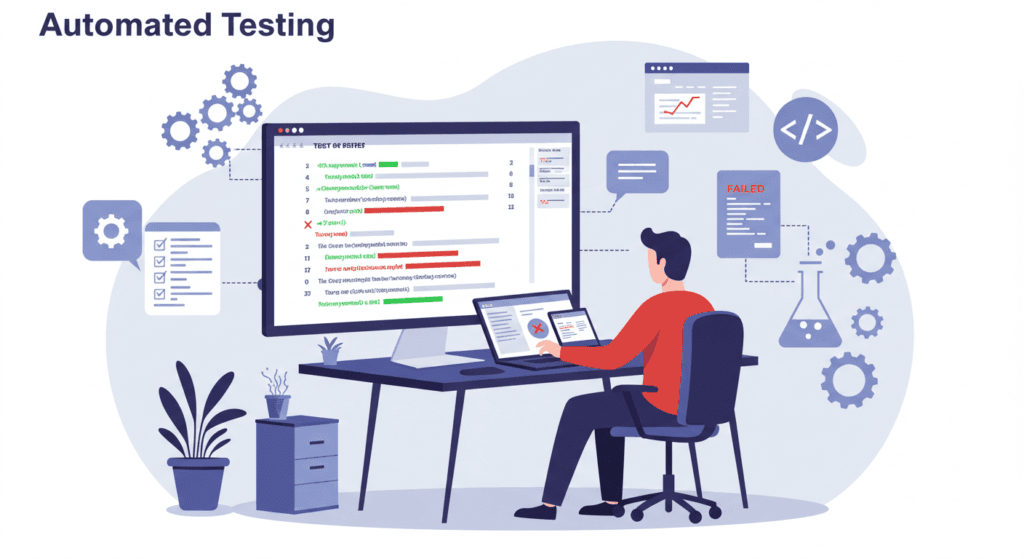
Real-world Example: In a CI/CD pipeline, when you push code to a GitHub repository, it automatically triggers tests. If the tests pass, the code is deployed to a staging environment for further testing. If everything checks out, the code is pushed to production. This process saves time and ensures consistency across deployments.
API Development
What is API development, and why is it important for Rails developers?
APIs (Application Programming Interfaces) are essential for connecting different applications and systems. API development is crucial for Rails developers, especially as applications increasingly rely on external data and services. Rails offers great support for building RESTful APIs and integrating them with other systems.
How do Rails developers build RESTful APIs?
REST (Representational State Transfer) is an architectural style used to design networked applications. Rails developers can create RESTful APIs by defining resources (e.g., users, products) and using routes to specify HTTP actions (GET, POST, PUT, DELETE) for each resource.
For instance, if you’re building an API for a blog application, you’d have a posts
resource with routes like:
GET /posts
to list all posts.POST /posts
to create a new post.GET /posts/:id
to retrieve a single post.PUT /posts/:id
to update a post.PATCH /posts/:id
: to update specific fields of a post.DELETE /posts/:id
to delete a post.
Real-world Example: If you’re creating a RESTful API for a task management app, the tasks
resource might include actions for creating, reading, updating, and deleting tasks. You could use Rails controllers like TasksController
and define actions like index
, show
, create
, and update
.
How do Rails developers handle JSON serialization?
When developing APIs, JSON serialization is a key part of the process. Rails provides tools like ActiveModel::Serializer or Fast JSONAPI to transform Ruby objects into JSON data, which is easier for clients to consume.
For example, in the task management app, the Task
model would be serialized into a JSON format with attributes like id
, title
, and completed
. Serialization ensures that only the necessary data is returned to the client, improving efficiency.
What about GraphQL?
GraphQL is a powerful alternative to RESTful APIs, allowing clients to request only the data they need. Unlike REST, where multiple endpoints might be needed for different resources, GraphQL uses a single endpoint and flexible queries to fetch related data.
Rails developers can use the graphql-ruby gem to implement GraphQL APIs. GraphQL offers more efficient data retrieval and provides a more tailored response to clients, which is especially useful in mobile applications where bandwidth may be limited.
How do you secure APIs?
Security is a top priority when developing APIs. Rails developers can use authentication methods like OAuth and JWT (JSON Web Tokens) to ensure that only authorized users can access the API.
- OAuth is commonly used for third-party integrations, such as logging in with Google or Facebook.
- JWT is a popular choice for securing API endpoints by issuing tokens that the client uses to authenticate subsequent requests.
Pro Tip: Always use HTTPS to secure communication between clients and your API to prevent data interception.
Security Best Practices
Why is security so critical for Rails developers?
Security is an ongoing concern for developers, especially when working with web applications. With sensitive user data like passwords and financial details often stored in databases, it’s essential to protect against common vulnerabilities and implement secure practices from the start.
How can Rails developers prevent common vulnerabilities?
Rails developers must be aware of common vulnerabilities like SQL injection, XSS (Cross-Site Scripting), and CSRF (Cross-Site Request Forgery). These can be avoided by following secure coding practices.
- SQL Injection: Rails uses ActiveRecord and parameterized queries, which help protect against SQL injection attacks. Never concatenate raw SQL queries with user input.
- XSS: Always escape user-generated content to prevent malicious scripts from being executed in the user’s browser. Rails automatically escape HTML by default in views, but be cautious when using raw HTML.
- CSRF: Rails includes CSRF protection by default with tokens in forms to prevent unauthorized users from submitting requests on behalf of others.
How can Rails developers secure user data?
Encryption is a vital practice for protecting sensitive data like passwords and credit card numbers. Rails provides the bcrypt gem for securely hashing passwords. Always store passwords as hashes, never in plain text.
For additional layers of security, consider encrypting data before storing it in the database, especially for personally identifiable information (PII). For instance, you can use Rails’ ActiveRecord Encryption to encrypt sensitive columns in the database.
How can Rails developers stay updated with security best practices?
Rails releases regular security patches and advisories to address new vulnerabilities. It’s important for Rails developers to stay updated by subscribing to mailing lists or using tools like Dependabot to monitor for vulnerable gems in your application.
Pro Tip: Make sure you regularly update Rails and other dependencies in your application to benefit from the latest security fixes.
Soft Skills and Continuous Learning
How do soft skills impact a Rails developer’s work?
In addition to technical expertise, soft skills like communication and collaboration are essential for a successful Rails developer. Being able to communicate complex technical concepts clearly and work well with teammates can significantly enhance the quality of a project.
- Communication: Effective communication ensures that everyone on the team understands project goals, progress, and obstacles. This is especially important in an Agile development environment where regular updates and feedback loops are key to success.
- Collaboration: Rails developers often work in teams, so being able to collaborate efficiently and resolve conflicts when they arise is critical to maintaining productivity and morale.
Why is adaptability important for Rails developers?
Rails developers need to be flexible and adaptable as technologies, tools, and frameworks evolve. The world of software development is constantly changing, and the ability to learn new skills and adjust to new tools or methodologies is key to long-term success.
How can Rails developers continue learning?
Continuous learning is an essential part of being a great developer. Rails developers should stay updated with new releases, community contributions, and trends in the Rails ecosystem. Participating in forums like Stack Overflow, attending meetups, and reading blogs or official Rails documentation are great ways to stay engaged and improve your skills.
Pro Tip: Follow prominent Ruby on Rails developers and contributors on Twitter or GitHub to stay informed about the latest industry trends and best practices.
Conclusion
Recap of Essential Skills
To be an effective Ruby on Rails developer, you need a combination of technical skills and soft skills. Mastering version control, API development, testing, debugging, security best practices, and deployment tools is essential for delivering high-quality applications. Additionally, strong communication, collaboration, and adaptability are equally important to thrive in a team environment.
Encouragement for Developers
The Rails ecosystem is constantly evolving, and so should you. Keep learning, stay curious, and embrace new challenges. Whether you’re just starting or have years of experience, there’s always room to grow and improve as a developer.
Call-to-action
We’d love to hear from you! Drop your thoughts, additional skills, or experiences in the comments on our LinkedIn and other social media platforms. Let’s keep the conversation going and learn together