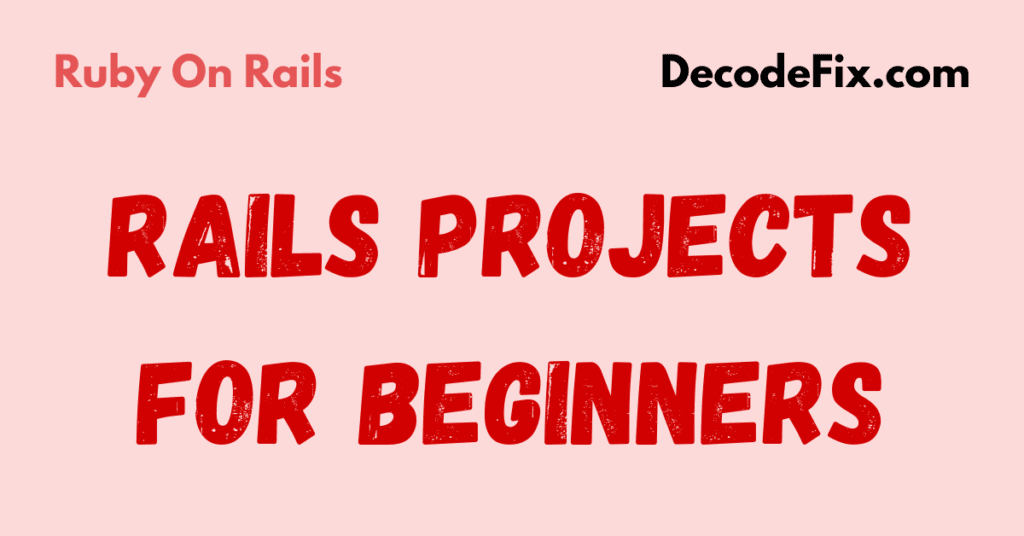
Ruby on Rails, often called Rails, is a web development framework that simplifies coding. It’s designed for developers of all levels but shines especially for beginners. Why? Rails is opinionated. It guides you toward best practices without overwhelming you. For instance, the “Convention over Configuration” principle minimizes decisions by providing defaults. This approach makes starting with Ruby on Rails projects for beginners both efficient and enjoyable, allowing you to focus more on building and less on setup.
Rails also come with powerful tools that are out of the box. Features like scaffolding, built-in testing, and a structured MVC (Model-View-Controller) architecture help you organize your code. For beginners, Rails bridges the gap between “just learning” and “actually creating.” With a few commands, you can have a functioning app. It’s like assembling a Lego set with instructions—rewarding and educational.
Getting Started: Setting Up Your Rails Development Environment
Before starting, set up your Rails workspace. Here’s what you need:
- Ruby: Rails runs on Ruby. Install the latest version of Ruby or use a version manager like RVM or rbenv.
- Rails: Install Rails via RubyGems. Use the command:
gem install rails
- Database: Start with SQLite, included by default. Later, you can explore PostgreSQL or MySQL for advanced projects.
- Editor: Choose a text editor or IDE like VS Code, Sublime Text, or RubyMine.
- Terminal Skills: Learn basic shell commands. Rails uses the terminal extensively.
Once everything is set up, create a new app with:
rails new my_project
This generates a ready-to-go project with folders for models, views, controllers, and more. Test your setup by running:
rails server
Visit localhost:3000
in your browser. If you see the Rails welcome page, you’re all set!
15 Ruby on Rails Projects for Beginners
Rails is versatile, so let’s explore projects that teach key concepts while building functional applications.
Project #1: Build a Simple To-Do List App
A to-do list app is one of the most classic beginner projects. It helps you understand the basics of Ruby on Rails, such as working with databases, creating models, and managing simple CRUD (Create, Read, Update, Delete) operations. You’ll build an app where users can add tasks, mark them as complete, and delete them. This project teaches essential skills, like setting up routes, controllers, and views, and helps you become comfortable with managing user input. Start by creating a Task
model:
rails generate model Task title:string completed:boolean
rails db:migrate
Next, create a controller and views for adding, displaying, and managing tasks. Use Rails form helpers to make task creation user-friendly.
Project #2: Create a Personal Blog Platform
Building a blog is a great way to dive into more advanced concepts, such as user authentication, text formatting, and managing blog posts with rich content. With this project, you will learn how to handle user sessions (logins and logouts), how to create a database schema for posts, and how to integrate features like text editors for formatting blog content. A blog app will also give you a deeper understanding of how to manage user-generated content securely. Generate a Post
scaffold for quick setup:
rails generate scaffold Post title:string content:text
rails db:migrate
Add styling with CSS or a framework like Bootstrap. To make your blog dynamic, implement pagination with the will_paginate gem.
Project #3: Develop an Inventory Management System
This project is ideal for beginners who want to learn about handling larger sets of data. You’ll build an app that allows users to track products, stock levels, suppliers, and more. Features might include adding new products, updating inventory levels, and generating reports. This project introduces you to more advanced database relationships, like has_many and belongs_to associations and allows you to practice working with data models in a real-world context. Create an Item
model to track stock levels:
class Item < ApplicationRecord
validates :name, presence: true
validates :quantity, numericality: { greater_than_or_equal_to: 0 }
end
Design views to list, add, and update inventory items. Include error messages for invalid entries.
Project #4: Design a Contact Management Application
A contact management app allows users to store, update, and delete contact information. This app introduces you to more complex form handling, validations, and data relationships. You will learn how to manage user inputs and build features like search functionality, grouping contacts by categories (work, personal, etc.), and importing/exporting contact data. This project gives you valuable practice in handling more complex user interfaces. Create a Contact
model with fields for name, email, and phone. Add features like search and sorting. Use Rails’ flash
messages to notify users of actions:
<% if flash[:notice] %>
<p><%= flash[:notice] %></p>
<% end %>
Project #5: Create a Recipe Sharing Platform
A recipe sharing platform lets users post, browse, and search for recipes. In addition to handling CRUD operations for recipes, this project involves managing user-uploaded images and perhaps even integrating third-party APIs for features like ingredient suggestions. You will get hands-on experience with file uploads, nested forms, and integrating various types of user inputs. It’s a fun way to practice Rails and develop a user-friendly app.
Project #6: Build a Basic Note-Taking App
In this project, you’ll create a simple note-taking app that allows users to save, edit, and delete notes. This project focuses on teaching you to work with databases, handling text data, and using validations to ensure notes are properly formatted. You can enhance the app by adding features like organizing notes into categories or providing users with the ability to search through their notes, making it a perfect project for beginners.
Project #7: Develop a Weather Dashboard Using an API
Integrating external APIs is an excellent way to extend your Rails skills. In this project, you’ll create a weather dashboard where users can input a city and view the weather conditions. By utilizing a weather API, you’ll learn how to make HTTP requests, process external data, and display it in a user-friendly format. This project also involves working with JavaScript, CSS, and HTML to make the interface more dynamic. Fetch data from OpenWeatherMap:
require 'net/http' require 'json' url = URI("https://api.openweathermap.org/data/2.5/weather?q=city&appid=your_api_key") response = Net::HTTP.get(url) weather_data = JSON.parse(response) puts weather_data['weather'][0]['description']
Project #8: Create a Budget Tracker for Personal Finance
A personal finance app allows users to track their income, expenses, and savings. You’ll learn how to create a system for inputting transactions, calculating balances, and organizing data by categories like food, entertainment, and bills. This project provides a great opportunity to practice Rails’ built-in support for working with forms, validations, and calculations while gaining real-world experience in developing financial tools.
Project #9: Build a Book Collection Organizer
This project is perfect for learning how to organize and categorize data. In the book collection organizer, users can add books, categorize them by genre, and track whether they’ve read them. You will learn how to set up relationships between models, create search and filter functionality, and work with different types of data, such as text, numbers, and dates. Additionally, you can explore ways to present the book collection in an engaging interface with various sorting and filtering options. Catalog your books by title, author, and genre. Add a model for authors and set up associations:
class Book < ApplicationRecord belongs_to :author end
Project #10: Develop a Basic Task Reminder Application
A task reminder app allows users to create tasks with deadlines and reminders. You’ll create features to notify users of upcoming tasks and send reminders via email or notifications. This project will help you practice working with background jobs, scheduling tasks, and integrating email services like SendGrid or Mailgun for notifications. It’s a great way to learn about working with time-based data and building an app that interacts with users in real-time. Use Rails’ Active Job
and Action Mailer
to send email notifications:
class ReminderMailer < ApplicationMailer def task_reminder(task) @task = task mail(to: @task.user.email, subject: 'Task Reminder') end end
Project #11: Create a Photo Gallery App with Upload Functionality
A photo gallery app lets users upload, view, and manage their images. You will learn how to integrate image upload functionality using Rails and popular gems like Paperclip or ActiveStorage. Additionally, you can implement image resizing, cropping, and categorization features. This project also teaches you how to handle file validation and securely store images, making it a perfect project to deepen your understanding of Rails and multimedia content management.
Project #12: Build a URL Shortener Tool
A URL shortener is a simple but highly functional app that takes a long URL and converts it into a shorter, easier-to-share link. This project introduces you to working with databases for generating unique short URLs and redirecting them to the original destination. You’ll also explore how to create custom URL structures and ensure that links are tracked or expire after a certain period. This project teaches essential skills related to data manipulation and web redirects. Use Rails routes to redirect users:
get '/:short_url', to: 'short_urls#redirect'
Project #13: Develop a Quiz Application with Score Tracking
A quiz app allows users to take quizzes, track their answers, and view their scores. This project involves creating forms for taking quizzes, managing questions, and scoring answers. You will also explore features like randomizing questions and offering immediate feedback based on users’ selections. You can enhance this project by adding an admin panel to create and manage quizzes, making it a practical, engaging project for beginners.
Project #14: Create a Portfolio Website with Rails
A personal portfolio website is a perfect project for showcasing your skills and projects. This Rails project will help you build a dynamic, interactive portfolio where you can display your work, list your skills, and include links to your projects. You’ll practice working with Rails to manage content, design layouts, and optimize the site for performance. You can also include a contact form for potential clients or employers to reach you.
Project #15: Design a Student Grade Tracker
In this project, you’ll create an app where students can track their grades for various subjects. Users will input their scores, and the app will calculate averages and display grades in an easy-to-read format. This project helps you practice working with numerical data, calculations, and creating dynamic charts or graphs. It’s also a great opportunity to explore user authentication, as you can design different interfaces for students, teachers, and admins. Use nested resources to organize data:
resources :students do resources :grades end
Tips for Customizing and Expanding These Projects
Once you’ve built a basic app, expand it. Add user authentication with Devise. Improve performance with caching. Integrate APIs or third-party services.
Tools, Gems, and Resources to Support Your Learning Journey
Explore these tools to enhance your Rails projects:
- Devise: Authentication
- Active Storage: File uploads
- Sidekiq: Background jobs
- RSpec: Testing
Conclusion: Your First Steps in Ruby on Rails Development
Starting Ruby on Rails projects for beginners is a great way to kick off your web development journey. With its beginner-friendly design, Rails lets you focus on creating functional, dynamic applications while learning core development principles like routing, database integration, and user interactions. Each project you tackle not only strengthens your technical skills but also builds your confidence. From simple to-do lists to interactive portfolio sites, these projects are stepping stones to mastering Rails and creating real-world applications. Keep experimenting, learning, and sharing your work—every project is a step toward becoming a skilled Rails developer.