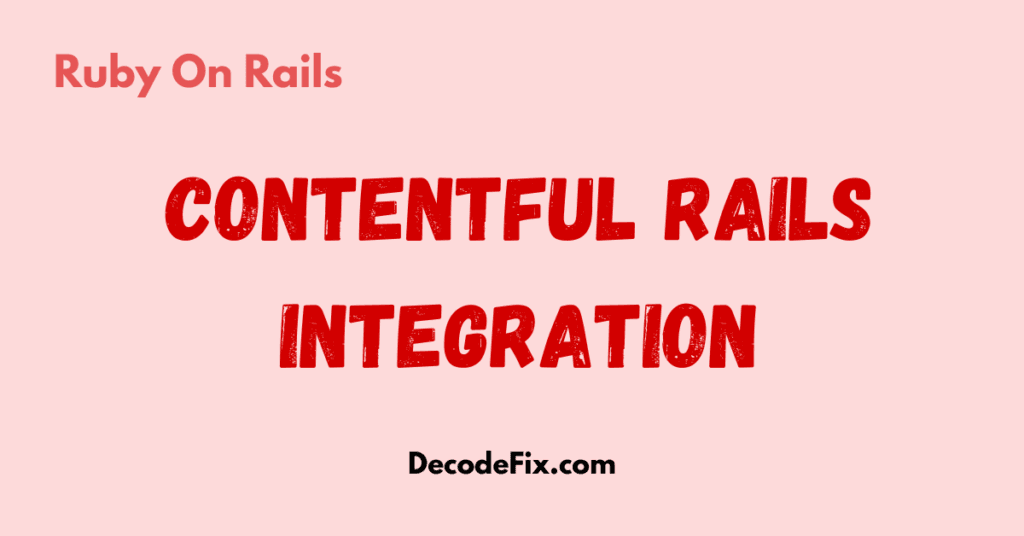
What is Contentful, and Why Use it With Rails?
Contentful is a powerful headless CMS that acts as a backend for managing content across multiple platforms. With Rails, you can fetch, display, and manage content efficiently. This integration provides a seamless way to structure your content dynamically while maintaining Rails’ simplicity.
In today’s fast-paced development, using a headless CMS like Contentful gives you flexibility. You can create, manage, and update your content without deploying your Rails application repeatedly. Content editors love it because it makes their job smoother, and developers appreciate its API-driven structure.
Setting Up Contentful in Your Rails App
To get started, you’ll need an API key from Contentful and the contentful
gem installed in your Rails application. Follow these steps to connect Rails with Contentful:
# Add this to your Gemfile
gem 'contentful'
# Run the bundle command to install
bundle install
Once the gem is installed, configure it by creating a new initializer file:
# config/initializers/contentful.rb
require 'contentful'
CONTENTFUL_CLIENT = Contentful::Client.new(
access_token: ENV['CONTENTFUL_ACCESS_TOKEN'], # Your Contentful API key
space: ENV['CONTENTFUL_SPACE_ID'], # Your Contentful Space ID
environment: ENV['CONTENTFUL_ENVIRONMENT'] # Optional, defaults to 'master'
)
Now, Rails can communicate with Contentful. Let’s query some data!
Fetching Content With the Contentful API
Contentful provides a rich API to fetch content. Here’s how you can retrieve entries from your space:
# app/controllers/articles_controller.rb
class ArticlesController < ApplicationController
def index
@articles = CONTENTFUL_CLIENT.entries(content_type: 'article')
end
end
This will fetch all entries of the article
content type. To display the content in your view:
<!-- app/views/articles/index.html.erb -->
<% @articles.each do |article| %>
<h2><%= article.fields[:title] %></h2>
<p><%= article.fields[:description] %></p>
<% end %>
Why Choose Contentful for Content Management?
Rails developers often rely on ActiveAdmin or similar tools for content management. However, Contentful eliminates the need for a heavy CMS tied directly to your app. With Contentful, content is decoupled, scalable, and future-proof. You can even use the same content for a Rails website, a React front end, and a mobile app.
Imagine this: you’re building an e-commerce site. Contentful allows your marketing team to update product descriptions without touching your Rails code. This independence improves collaboration between teams.
Real-World Use Cases of Contentful + Rails
- Blog Platforms: Contentful powers blogs with dynamic categories, tags, and multi-language support.
- E-commerce: Rails handles the storefront, while Contentful manages the catalog.
- Marketing Sites: With Contentful, marketers can update landing pages without bothering developers.
Netflix and Spotify use headless CMS solutions similar to Contentful. Their platforms rely on structured, API-first content systems to deliver seamless experiences.
Handling Webhooks in Rails
Want real-time updates? Contentful supports webhooks to notify your Rails app when content changes. Set up a webhook in Contentful, then add this endpoint in your Rails app:
# config/routes.rb
post 'contentful/webhook', to: 'contentful#webhook'
# app/controllers/contentful_controller.rb
class ContentfulController < ApplicationController
skip_before_action :verify_authenticity_token
def webhook
# Handle webhook events
Rails.logger.info("Received webhook: #{params}")
head :ok
end
end
This ensures your app always has the latest content. Combine it with background jobs like Sidekiq for efficient processing.
Integrating Contentful With Active Storage
If you’re using Active Storage for image uploads, Contentful’s asset management can complement it. Fetch image URLs from Contentful like this:
@articles.each do |article|
image_url = article.fields[:image]&.url
end
Use these URLs in your views for on-the-fly asset rendering.
Improving SEO With Contentful
SEO is crucial for Rails apps. Contentful’s structured content makes it easy to add meta tags, Open Graph data, and more. A typical implementation:
# app/helpers/meta_tags_helper.rb
module MetaTagsHelper
def meta_tags(entry)
content_tag(:meta, nil, name: 'description', content: entry.fields[:meta_description]) +
content_tag(:meta, property: 'og:title', content: entry.fields[:title]) +
content_tag(:meta, property: 'og:image', content: entry.fields[:image]&.url)
end
end
This dynamic approach ensures your app stays SEO-friendly.
Deploying Contentful-Integrated Rails Apps
When deploying, ensure your ENV variables (API key, space ID, etc.) are securely configured. Services like Heroku and AWS support this. Use gems like dotenv-rails
for local development:
# .env file
CONTENTFUL_ACCESS_TOKEN=your_api_key
CONTENTFUL_SPACE_ID=your_space_id
CONTENTFUL_ENVIRONMENT=master
Deployments become hassle-free with this setup.
Final Thoughts
Integrating Contentful with Rails offers unmatched flexibility. It lets developers focus on building apps while empowering content creators to manage data independently. The combination of Rails’ speed and Contentful’s structure creates scalable, future-ready applications.
Try it out, and you’ll see the difference. If your team is debating switching to a headless CMS, Contentful might just be the missing piece in your stack!