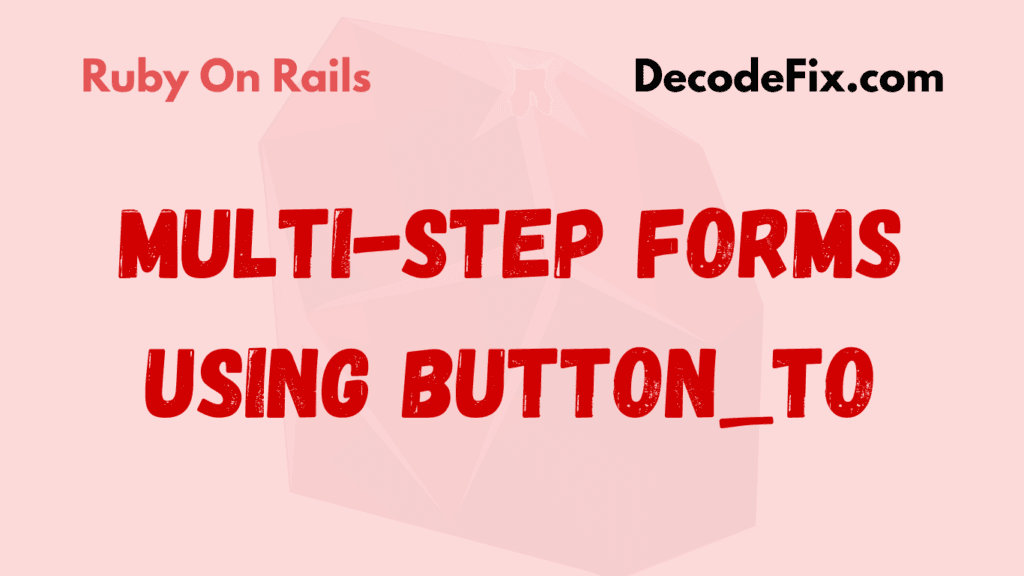
In Rails, button_to is a versatile helper method used to create form buttons that trigger HTTP requests. Unlike link_to
, which generates links, button_to
creates actual form buttons encapsulated within a form tag. This makes it suitable for actions that require submitting data or performing server-side operations.
Here’s a simple example of how button_to
works:
<%= button_to 'Submit', some_path, method: :post %>
This generates:
<form method="post" action="/some_path">
<button type="submit">Submit</button>
</form>
Multi-step forms break complex forms into smaller steps, enhancing usability. Using button_to
for navigation within multi-step forms simplifies form submissions and enhances user experience by:
- Ensuring forms align with RESTful design principles.
- Supporting seamless navigation with appropriate HTTP methods (e.g.,
:get
,:post
). - Allowing integration of form states and data preservation across steps.
Understanding Multi-step Forms in Rails
Benefits of Multi-step Forms in Web Applications
Multi-step forms improve user interaction by:
- Reducing cognitive load by presenting smaller chunks of information.
- Enabling progressive data collection for complex forms (e.g., registration processes).
- Improving conversion rates as users are less likely to abandon short forms.
How Multi-step Forms Work in Rails
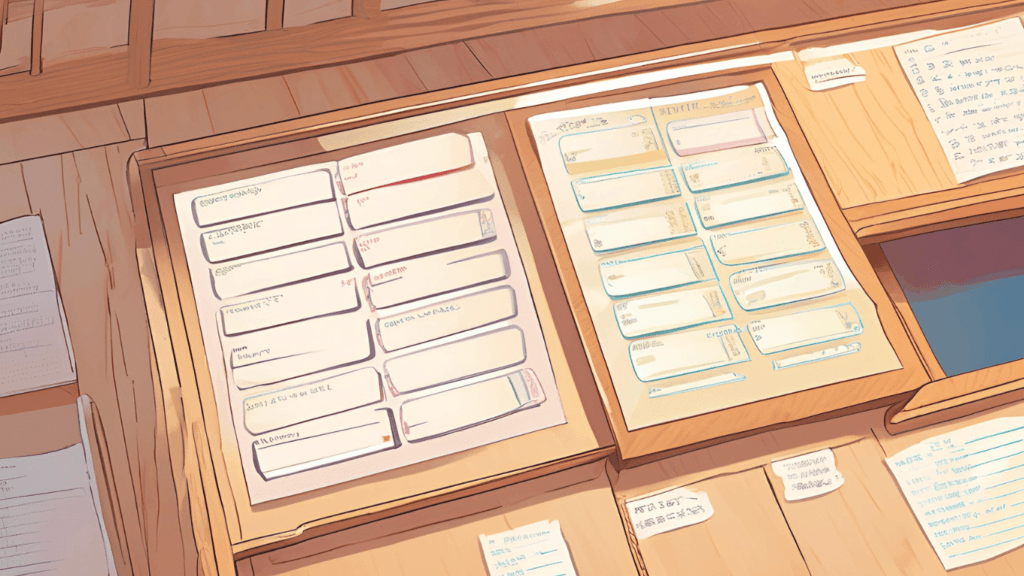
Multi-step forms in Rails generally involve:
- Splitting Forms into Steps: Each step corresponds to a specific part of the form.
- Managing State: Preserving data as users navigate between steps.
- Validating Inputs: Ensuring each step validates the data before proceeding.
Rails’ RESTful routes and helpers like button_to
make it easy to implement these steps effectively.
Setting Up the Rails Project
Basic Setup for Multi-step Forms
- Create a Rails Application:
rails new multi_step_form_app
cd multi_step_form_app
- Generate a Model:
rails generate model User name:string email:string age:integer
rake db:migrate
- Create a Controller:
rails generate controller Users new step1 step2 step3 create
Installing Required Gems and Dependencies
To streamline multi-step forms, install these gems:
- Simple Form: For simplified form creation.
bundle add simple_form
rails generate simple_form:install
- Wicked Gem: For managing step navigation (optional).
bundle add wicked
Feature | button_to | link_to |
---|---|---|
Purpose | Generates form buttons | Generates anchor tags |
HTTP Methods | Supports :post , :put , :delete | Typically used for :get |
Form Encapsulation | Encapsulates button within a form tag | No form tag generated |
Use Case | Submitting data, triggering server-side actions | Navigation links or actions |
For multi-step forms, you can use button_to
to navigate between steps:
<%= button_to 'Next Step', step2_users_path, method: :get, params: { name: @user.name } %>
<%= button_to 'Previous Step', step1_users_path, method: :get %>
These buttons allow users to move forward and backward while preserving form data.
Creating a Multi-step Form Workflow
Breaking Your Form into Multiple Steps
Step 1: Define Routes
In config/routes.rb
, define routes for each step:
resources :users do
collection do
get :step1
get :step2
get :step3
post :create
end
end
Step 2: Create Views for Each Step
1. View:
<%= simple_form_for @user, url: step2_users_path, method: :get do |f| %>
<%= f.input :name, label: 'Enter Your Name' %>
<%= button_to 'Next Step', step2_users_path, method: :get, params: { name: @user.name } %>
<% end %>
2. View:
<%= simple_form_for @user, url: step3_users_path, method: :get do |f| %>
<%= f.input :email, label: 'Enter Your Email' %>
<%= button_to 'Next Step', step3_users_path, method: :get, params: { email: @user.email } %>
<%= button_to 'Previous Step', step1_users_path, method: :get %>
<% end %>
3. View:
<%= simple_form_for @user, url: users_path, method: :post do |f| %>
<%= f.input :age, label: 'Enter Your Age' %>
<%= button_to 'Submit', users_path, method: :post %>
<%= button_to 'Previous Step', step2_users_path, method: :get %>
<% end %>
Use session
to manage state between steps. Example:
Controller:
class UsersController < ApplicationController
def step1
@user = User.new(session[:user_params] || {})
end
def step2
session[:user_params] ||= {}
session[:user_params].merge!(params[:user]) if params[:user]
@user = User.new(session[:user_params])
end
def step3
session[:user_params].merge!(params[:user]) if params[:user]
@user = User.new(session[:user_params])
end
def create
@user = User.new(session[:user_params])
if @user.save
session[:user_params] = nil
redirect_to users_path, notice: 'User created successfully!'
else
render :step3
end
end
end
This workflow ensures smooth navigation between steps while preserving data. With button_to
, you can create robust and user-friendly multi-step forms in Rails.
In multi-step forms, navigation is key. Rails’ button_to
makes it easy to create Next and Previous buttons with clean syntax. Here’s how you can implement navigation:
# Example: Next Button in a Form
<%= button_to 'Next', next_step_path, method: :post, params: { current_step: @current_step } %>
# Example: Previous Button
<%= button_to 'Previous', previous_step_path, method: :post, params: { current_step: @current_step } %>
Managing Data Retention Between Steps
To retain user data between steps, leverage Rails’ session or hidden fields:
Using Hidden Fields
Hidden fields pass data between steps seamlessly:
<%= form_with url: next_step_path, method: :post do %>
<%= hidden_field_tag :user_data, @user_data.to_json %>
<%= button_to 'Next', next_step_path, method: :post %>
<% end %>
Using Session
Store form data in the session for retrieval in later steps:
# Controller
session[:user_data] ||= {}
session[:user_data].merge!(params[:user_data])
# Retrieving Data
@user_data = session[:user_data]
Styling Buttons with CSS Classes
Customize the appearance of your buttons using CSS classes:
<%= button_to 'Submit', submit_path, class: 'btn btn-primary' %>
Define your styles in CSS:
.btn {
padding: 10px 20px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 5px;
}
.btn:hover {
background-color: #0056b3;
}
Adding Icons and Conditional Logic
Enhance buttons with icons or conditional rendering:
Adding Icons
<%= button_to raw("<i class='icon-next'></i> Next"), next_step_path, class: 'btn btn-next' %>
Conditional Logic
Display buttons conditionally based on the current step:
<% if @current_step < @total_steps %>
<%= button_to 'Next', next_step_path, method: :post %>
<% end %>
Handling Validation and Error Messages
Validating Each Step of the Multi-step Form
Validate data step-by-step to ensure a smooth user experience. Use Rails’ ActiveModel validations:
class UserStepValidator
include ActiveModel::Model
attr_accessor :name, :email
validates :name, presence: true, if: -> { current_step == 1 }
validates :email, presence: true, format: { with: /@/ }, if: -> { current_step == 2 }
end
Displaying Errors Using Rails Flash Messages
Show validation errors using flash messages:
# Controller
if @user_step.invalid?
flash[:error] = @user_step.errors.full_messages.join(", ")
redirect_to current_step_path
end
# View
<% if flash[:error] %>
<div class="alert alert-danger">
<%= flash[:error] %>
</div>
<% end %>
Saving Data After the Final Step
Submitting Multi-step Form Data
Gather all the data at the last step and save it to the database:
# Controller
if @user_form.complete?
User.create(@user_form.data)
session.delete(:user_data)
redirect_to success_path, notice: 'Form submitted successfully!'
end
Using Rails Controllers to Handle Form Submission
Handle submission logic in a dedicated controller:
class MultiStepFormsController < ApplicationController
def submit
@user = User.new(form_data)
if @user.save
redirect_to success_path
else
flash[:error] = @user.errors.full_messages.join(", ")
redirect_to last_step_path
end
end
private
def form_data
session[:user_data]
end
end
Add AJAX functionality to button_to
for dynamic updates:
<%= button_to 'Next', next_step_path, remote: true, method: :post %>
Handle AJAX responses in JavaScript:
document.addEventListener("ajax:success", function(event) {
const [data, status, xhr] = event.detail;
document.getElementById("step-container").innerHTML = xhr.responseText;
});
Enhancing Accessibility and User Experience
Ensure buttons are accessible:
- Use ARIA labels:
<%= button_to 'Next', next_step_path, aria: { label: 'Go to the next step' } %>
- Provide keyboard navigation.
Common Challenges and How to Overcome Them
Debugging Issues in Multi-step Forms
- Use
Rails.logger.debug
to track session data and parameters. - Check browser dev tools for AJAX issues.
- Always specify the HTTP method (e.g.,
method: :post
). - Handle edge cases, such as users skipping steps by manipulating URLs.
Conclusion and Best Practices
- Flexibility:
button_to
can handle various multi-step form scenarios. - Customization: Tailor buttons with CSS and conditional logic.
- Error Handling: Validate and show errors dynamically.
Best Practices for Building Multi-step Forms
- Keep It Simple: Minimize the number of steps.
- Retain Data: Use sessions or hidden fields to retain data.
- Accessibility: Ensure buttons are usable by all users.
By following these tips, you can leverage Rails’ button_to
helper to create efficient, user-friendly multi-step forms that enhance the user experience and streamline data collection.