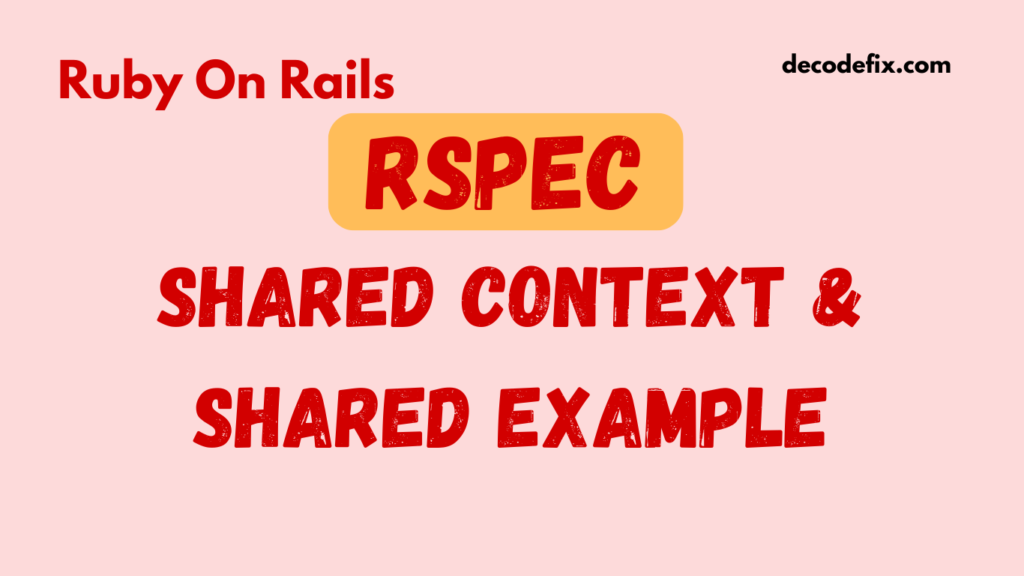
Writing maintainable, DRY (Don’t Repeat Yourself) test code is essential for scalable and robust test suites. In Ruby, RSpec is a popular framework that helps developers write clean, organized, and effective tests. Within RSpec, tools like shared context and shared example are fundamental for achieving DRY tests, especially when you need to reuse configurations, behaviors, or expectations across multiple examples.
In RSpec, shared contexts let you set up conditions, data, or configuration blocks that you can reuse in multiple test groups. They are perfect when you need to establish a shared let
statements, before
hooks, or other setup details that apply to a group of tests.
A shared context is defined using shared_context
and can be included in examples or describe blocks by calling include_context
. Here’s a basic example:
RSpec.shared_context 'common setup' do
let(:user) { User.new(name: 'Jane Doe') }
before { allow_any_instance_of(User).to receive(:admin?).and_return(true) }
end
RSpec.describe 'User behavior' do
include_context 'common setup'
it 'should have a name' do
expect(user.name).to eq('Jane Doe')
end
it 'should be an admin' do
expect(user.admin?).to be true
end
end
In this example, the shared_context
defines a user and a setup block. By calling include_context
, we make this shared setup available to all examples within the User behavior
test suite.
- Code Reusability: You avoid duplicating setup code across multiple test files.
- Maintenance: Updating a shared context automatically updates all tests that use it.
- Readability: Test setup is kept DRY and clean, improving readability.
Shared examples allow you to define behaviors that should apply to different objects or scenarios. With shared examples, you specify how an object should behave, and you can reuse these behaviors across different tests. Shared examples are ideal when testing multiple objects or models that share common behavior.
Here’s how to define and use shared examples:
RSpec.shared_examples 'a valid user' do
it 'has a name' do
expect(user.name).to be_present
end
it 'has an email' do
expect(user.email).to match(/\A[^@\s]+@[^@\s]+\z/)
end
end
RSpec.describe 'AdminUser' do
let(:user) { AdminUser.new(name: 'Admin', email: 'admin@example.com') }
it_behaves_like 'a valid user'
end
RSpec.describe 'RegularUser' do
let(:user) { RegularUser.new(name: 'User', email: 'user@example.com') }
it_behaves_like 'a valid user'
end
- Consistency: Ensures that multiple classes or objects adhere to the same behavior.
- Efficiency: Eliminates repeated test code by creating a single, reusable behavior set.
- Modularity: Isolates and organizes common behaviors for easy reference and use.
Although shared contexts and shared examples might seem similar, they serve different purposes and are used in distinct scenarios.
Feature | Shared Context | Shared Example |
---|---|---|
Purpose | Setting up data or configurations. | Defining reusable behaviors for objects. |
Use Case | Useful for setting let blocks, before hooks. | Used for sharing common behavior or expectations. |
Definition | shared_context 'name' do ... end | shared_examples 'name' do ... end |
Invocation | include_context 'name' | it_behaves_like 'name' |
In short:
- Use shared contexts when you need to reuse test setup configurations.
- Use shared examples when you need to reuse behaviors or expectations across multiple examples.
Using Context in RSpec
In RSpec, context
is a way to define a specific scenario or condition under which certain tests should run. Unlike shared contexts or shared examples, which are reusable across multiple examples, context
is generally used to organize related examples within a single spec.
Here’s an example where context
helps organize tests for different scenarios:
RSpec.describe 'User login' do
context 'when the user is an admin' do
let(:user) { User.new(admin: true) }
it 'allows access to admin dashboard' do
expect(user.can_access_admin_dashboard?).to be true
end
end
context 'when the user is a regular user' do
let(:user) { User.new(admin: false) }
it 'denies access to admin dashboard' do
expect(user.can_access_admin_dashboard?).to be false
end
end
end
In this example, context
helps differentiate between an admin and a regular user scenario within the same spec, improving test readability.
One powerful feature of shared contexts in RSpec is the ability to parameterize them. This allows you to set different values or configurations each time you include the context, depending on the specific test case.
To use shared contexts with parameters, you can pass arguments to include_context
like this:
RSpec.shared_context 'with custom user' do |name, role|
let(:user) { User.new(name: name, role: role) }
end
RSpec.describe 'User roles' do
include_context 'with custom user', 'Alice', 'Admin'
it 'should have the name Alice' do
expect(user.name).to eq('Alice')
end
it 'should have the role Admin' do
expect(user.role).to eq('Admin')
end
context 'with a different user' do
include_context 'with custom user', 'Bob', 'Editor'
it 'should have the name Bob' do
expect(user.name).to eq('Bob')
end
it 'should have the role Editor' do
expect(user.role).to eq('Editor')
end
end
end
In this example:
- We define a shared context
with custom user
that takes parametersname
androle
. - By passing values to
include_context
, we can customize theuser
setup for each test scenario.
- Flexibility: Allows you to reuse contexts with specific setups, reducing the need for separate contexts for minor variations.
- Customization: You can adjust shared data or configurations dynamically, making your tests more adaptable to different scenarios.
1. Authentication Testing
Shared contexts are especially helpful in authentication tests where you may need to simulate logged-in and logged-out states.
RSpec.shared_context "logged in user" do
before do
@user = User.create(name: "Test User", email: "test@example.com")
login_as(@user)
end
end
RSpec.describe "Profile page" do
include_context "logged in user"
it "shows user information" do
visit "/profile"
expect(page).to have_content("Test User")
end
end
In this scenario, the shared context handles the login setup, reducing the setup needed for each test that requires a logged-in user.
2. Testing Different User Roles
For applications with different user roles, shared contexts help simulate role-specific setups.
RSpec.shared_context "admin setup" do
let(:user) { User.new(role: "admin") }
end
RSpec.shared_context "regular user setup" do
let(:user) { User.new(role: "user") }
end
RSpec.describe "Access control" do
context "as admin" do
include_context "admin setup"
it "has access to admin panel" do
expect(user.role).to eq("admin")
end
end
context "as regular user" do
include_context "regular user setup"
it "does not have access to admin panel" do
expect(user.role).to eq("user")
end
end
end
With these shared contexts, you create test cases specific to different roles without duplicating the role setup.
- Shared Context: Use when the primary need is shared setup, including
let
statements andbefore
hooks. - Shared Example: Use when you need to verify that different classes or objects adhere to the same behavior or set of expectations.
Structuring Tests for Readability
Using shared contexts and shared examples should make your tests more readable, not more complex. Avoid overusing them when simple tests will suffice. Each shared piece should be relevant and specific to the examples that use it.
Avoid Naming Conflicts
When defining let
variables in shared contexts, be mindful of potential naming conflicts with variables in the main example group. Consider prefixing variable names to avoid accidental overwriting.
When using shared contexts in RSpec, follow these practices:
- Keep Contexts Focused: Avoid overloading shared contexts with unrelated setups. Each shared context should serve a clear, specific purpose.
- Name Contexts Meaningfully: Use descriptive names for shared contexts to make your test suite understandable.
- Limit Deep Nesting: Avoid excessive nesting with
include_context
and metadata. Deep nesting can make tests challenging to debug. - Combine with Hooks When Necessary: Use hooks like
before
orafter
within shared contexts to set up and tear down dependencies efficiently. - Document Shared Contexts: Commenting or documenting shared contexts can help team members understand the context’s purpose quickly.
Conclusion
RSpec’s shared contexts and shared examples are invaluable tools for creating maintainable and DRY test suites. While they serve different purposes, both contribute to cleaner, more readable code by allowing developers to reuse setup and expectations across tests.
- Shared Context is ideal for setting up test data and configurations that multiple examples can use.
- Shared Example is perfect for defining behaviors that various classes or objects should share.
By leveraging these tools effectively, you can streamline your tests, reduce redundancy, and make your code more maintainable. Whether you’re dealing with complex applications or simple models, RSpec gem’s shared features will help keep your test code organized and efficient.