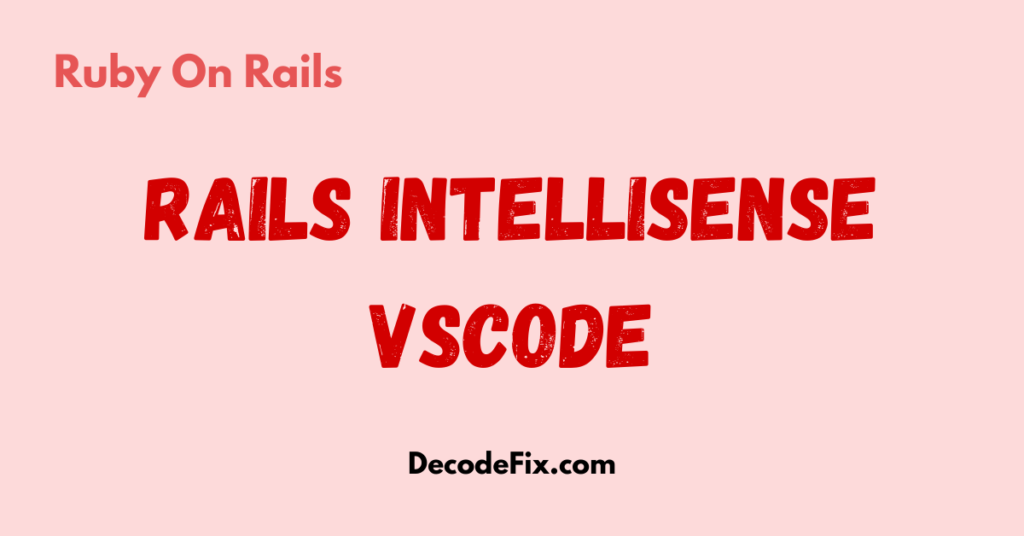
Ruby on Rails is a favorite for building web applications quickly. Combine it with Visual Studio Code (VS Code), and you get a powerful environment. But coding efficiency often hinges on tools like IntelliSense, which makes coding faster, easier, and less error-prone.
VS Code, with Ruby extensions and IntelliSense, simplifies everything from navigating your project to writing clean, bug-free code. If you’re wondering how to integrate IntelliSense for Ruby on Rails in VS Code, you’ve landed in the right place.
What Is IntelliSense?
IntelliSense provides code suggestions, auto-completions, and inline documentation. It acts like a helpful assistant, offering:
- Method suggestions as you type.
- Quick information about classes and methods.
- Real-time error detection.
Think of IntelliSense as a GPS for your code. Instead of guessing, it guides you directly to your destination.
Setting Up Ruby on Rails IntelliSense in VS Code
Before diving into setup, ensure you have Ruby, Rails, and VS Code installed on your machine.
Install Required Extensions
- Ruby Extension Pack:
Install the “Ruby” extension by Peng Lv. It includes debugging, syntax highlighting, and IntelliSense features.
Pro Tip: If you’re unsure which to pick, start with this one. - Solargraph:
Solargraph powers IntelliSense. It’s like having a dictionary and thesaurus for your Ruby project.
Command to Install Solargraph
Open your terminal and run:
gem install solargraph
Solargraph enhances autocomplete and provides method documentation.
Configuring VS Code for Ruby on Rails IntelliSense
Add Solargraph to Your Workspace
Ensure Solargraph recognizes your project. Run this command in your Rails project folder:
bundle exec yard gems
This generates documentation for your gems. Next, create or update the .solargraph.yml
file:
include:
- "**/*.rb"
exclude:
- "node_modules/**/*"
- "log/**/*"
- "tmp/**/*"
Restart VS Code for changes to take effect.
Enable IntelliSense in Settings
To optimize IntelliSense, tweak VS Code’s settings. Go to Settings and add:
"solargraph.autoformat": true,
"solargraph.diagnostics": true,
"solargraph.completion": true
These settings ensure smooth auto-completion and inline error messages.
IntelliSense in Action
Let’s look at an example. Imagine you’re writing a controller in Rails:
class PostsController < ApplicationController
def index
@posts = Post.all
end
end
As you type Post.
, IntelliSense suggests methods like find
, all
, or where
. No need to jump between files or Google method names—it’s all there.
Troubleshooting Common Issues in Rails IntelliSense
Even with setup complete, you may encounter hiccups. Here’s how to handle them:
IntelliSense Not Working?
- Check Dependencies: Ensure
solargraph
is installed and updated:
gem update solargraph
- Restart VS Code: Sometimes a simple restart fixes the issue.
Performance Slowdowns?
Disable unnecessary extensions to speed things up. Go to the Extensions tab and disable those you’re not using.
Boosting Productivity with Advanced Tips
Debugging Made Easy
With IntelliSense, debugging becomes less painful. Add breakpoints in your code by clicking in the margin. Run:
rails s
And watch your app halt execution at critical points. IntelliSense lets you inspect variable states with ease.
Explore Your Gems
Solargraph reads your Gemfile. Type a gem name, and IntelliSense suggests methods included in that gem. For instance, if you’re using devise
, it might suggest authenticate_user!
or current_user
.
Why IntelliSense Matters
Time is money, and IntelliSense saves both. It eliminates mundane tasks like manually searching documentation, allowing you to focus on solving problems. Whether you’re a junior developer or a seasoned pro, IntelliSense reduces frustration and boosts confidence.
Code Example of Rails and IntelliSense Magic
Here’s a more advanced Rails example to see IntelliSense shine:
class UsersController < ApplicationController
before_action :set_user, only: [:show, :edit, :update, :destroy]
def index
@users = User.order(created_at: :desc)
end
def show
end
def edit
end
private
def set_user
@user = User.find(params[:id])
end
end
As you type methods like order
or find
, IntelliSense lists options, helping you avoid typos and understand method parameters.
Final Thoughts
Setting up Ruby on Rails IntelliSense in VS Code might feel like assembling IKEA furniture—tedious at first, but incredibly rewarding once complete. The payoff? Faster development, fewer bugs, and more time for what matters: building great apps.
So, what are you waiting for? Install IntelliSense today and supercharge your Ruby on Rails coding experience!