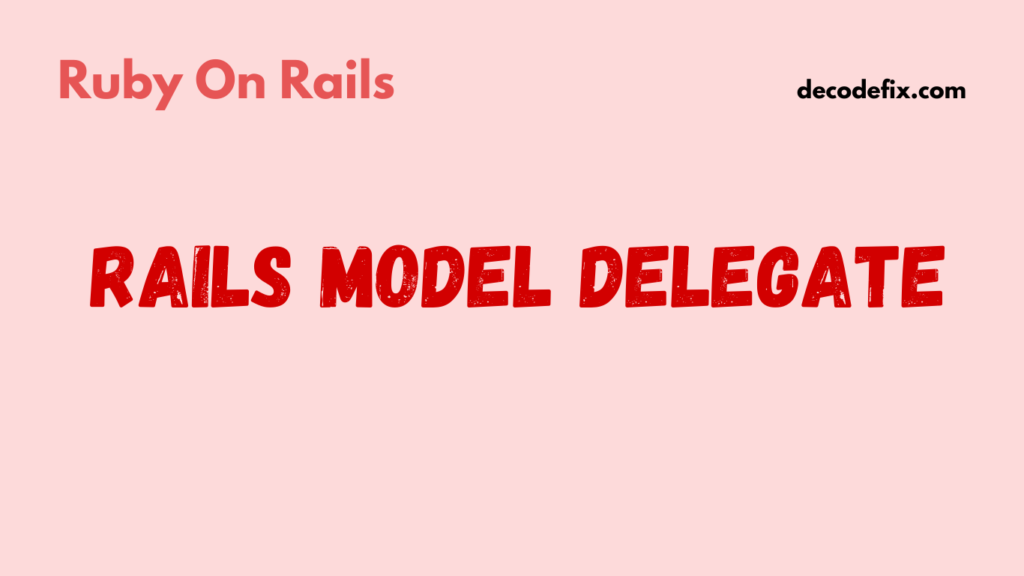
Rails developers know that organizing code efficiently and keeping methods slim is key. Rails model delegate can make your code cleaner, more readable, and easier to maintain. When used correctly, it lets you call methods on associated models directly, improving clarity and reducing method clutter. In this post, we’ll explore how delegate
works, why it’s so useful, and how to implement it effectively.
What is delegate in Rails?
In Rails, delegate
is a method that allows a model to use methods from another related model without explicitly calling that model. Essentially, it forwards a call to another object. Think of it as giving one model access to another model’s methods without creating duplicate methods.
Why Use Delegation?
Delegation saves time and keeps your codebase organized. Here’s why it’s worth using:
- Reduces code repetition: It allows you to avoid writing repetitive methods just to pass data from one model to another.
- Improves readability: Delegation makes it easier for others (and you) to understand your code at a glance.
- Encapsulates logic: It simplifies the calling code, ensuring that your logic is in the model where it belongs.
The Basics of Rails Model Delegation
Using delegate
is straightforward. You specify the methods you want to delegate, the model to which they belong, and add them in the form of one line in your main model. Let’s look at an example.
Basic Example of delegate
Suppose we have two models, Order
and Customer
. The Customer
model contains the name
attribute, but we want to access the customer’s name directly from an Order
object.
class Order < ApplicationRecord
belongs_to :customer
delegate :name, to: :customer
end
Now, instead of writing @order.customer.name
, we can simply write @order.name
. This keeps our code more readable and saves us from calling through two levels of objects.
Delegating Multiple Methods
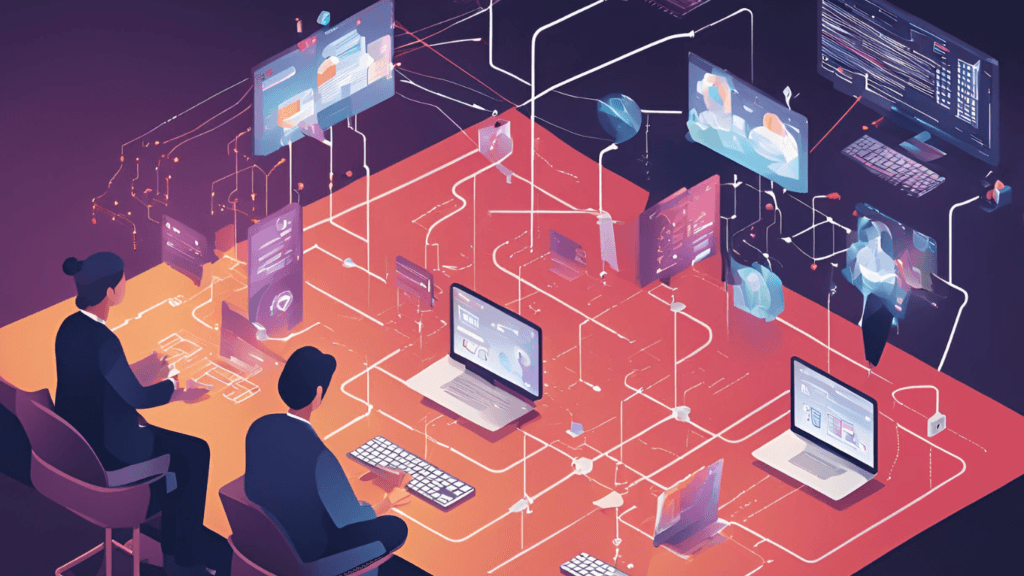
If you want to delegate multiple methods, Rails makes it easy:
class Order < ApplicationRecord
belongs_to :customer
delegate :name, :email, :phone_number, to: :customer
end
With the above code, you can call @order.name
, @order.email
, and @order.phone_number
without directly referencing the customer
association each time.
Customizing Your Delegate
Rails provides additional options with delegate
, giving you control over error handling and naming.
Using prefix in Rails Delegate
The prefix
option is helpful when you have similar method names across associated models. If both Order
and Customer
have a name
attribute, you can use the prefix
option to avoid confusion:
class Order < ApplicationRecord
belongs_to :customer
delegate :name, to: :customer, prefix: true
end
Now, instead of @order.name
, you’ll use @order.customer_name
. This is especially useful when dealing with models with similar fields.
Handling nil Values with allow_nil in Rails Delegate
Sometimes, you may want to delegate a method even if the association is nil
. By default, Rails will throw an error if you try to delegate to a nil
object. Using the allow_nil
option, you can return nil
gracefully instead of raising an error.
class Order < ApplicationRecord
belongs_to :customer
delegate :name, to: :customer, allow_nil: true
end
With allow_nil: true
, if @order.customer
is nil
, @order.name
will return nil
instead of raising an error. This can make your app more resilient and avoid potential crashes.
Rails Delegate to Instance Variable
Sometimes you might need to delegate methods to an instance variable or a specific object in your model that isn’t an ActiveRecord association. Rails allows you to delegate to instance variables as well. You can simply pass an instance variable to delegate
.
Let’s say you have an instance variable @profile
in your model and want to delegate full_name
to that instance variable:
class User < ApplicationRecord
delegate :full_name, to: :profile
end
Here, @profile
would be another object (possibly another model or a service), and calling user.full_name
would return the full_name
from the @profile
object.
Ruby Delegate Pattern in Rails
In software design, the delegate pattern is a structural pattern that allows an object to pass on a task to another object. In Rails, the delegate pattern is implemented using the delegate
method to forward method calls from one object to another. This simplifies your models and removes the need to write redundant code.
For example, if you have a User
model that needs to access a Profile
model, instead of writing a custom method in User
to fetch the full_name
from Profile
, you can delegate the method call directly:
class User < ApplicationRecord
has_one :profile
delegate :full_name, to: :profile
end
By using delegation, you avoid writing custom getter methods and keep your code DRY (Don’t Repeat Yourself).
Forwardable Module in Ruby
Ruby provides the Forwardable
module, which can be included in a class to forward specific methods to another object. This module is a core part of Ruby’s delegation pattern and offers an alternative to the delegate
method in Rails.
Here’s an example using Forwardable
:
require 'forwardable'
class Car
extend Forwardable
def_delegators :@engine, :start, :stop
def initialize
@engine = Engine.new
end
end
In this example, we use def_delegators
to forward the start
and stop
methods to the @engine
object. This allows Car
to delegate those actions directly to the engine without exposing its implementation.
Explicit Delegation in Rails
While delegate
and Forwardable
are convenient, sometimes you may need to manually delegate method calls. Explicit delegation involves writing the code to forward method calls manually. Though it’s not as elegant as using delegate
, it gives you total control.
For example:
class User
def full_name
profile.full_name
end
end
This approach can be useful when you need more fine-grained control over the delegation process, but it’s often more verbose and error-prone compared to Rails delegate
method.
Real-World Example of E-Commerce Platform
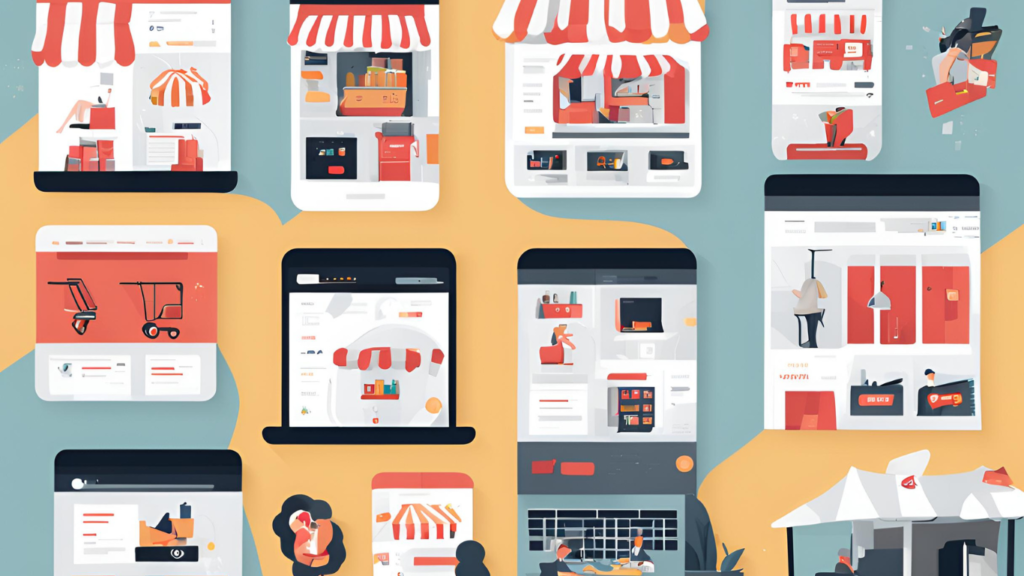
Let’s consider a real-world example involving an e-commerce platform. Imagine you have three models: Order
, Customer
, and Address
. Each Customer
has an associated Address
, and each Order
is linked to a Customer
.
If you need to display the customer’s address for an order, delegation can simplify things. Instead of writing:
@order.customer.address.street
@order.customer.address.city
@order.customer.address.zip_code
You can set up delegation in Order
to pull in those details:
class Order < ApplicationRecord
belongs_to :customer
delegate :street, :city, :zip_code, to: :address, prefix: true
end
This code makes it possible to call @order.address_street
, @order.address_city
, and @order.address_zip_code
without dealing with nested associations every time.
Potential Pitfalls of Delegation in Rails
Overusing Delegation
While delegation is powerful, overusing it can lead to confusion. Delegate methods should logically belong to the associated model. If Order
starts delegating dozens of methods from Customer
, it can blur the line between the two models and reduce code readability.
Performance Concerns
Delegating methods increase the number of calls between objects, which could have performance implications. For example, if you’re delegating methods that are frequently called in large collections, this could lead to unnecessary database queries. Consider caching or eager loading in cases where performance is critical.
Code Duplication
Sometimes, delegating too many methods may indicate a need for model restructuring. If two models share a lot of methods, you might want to consider combining them or extracting the shared methods into a concern or module.
Best Practices for Using Delegate in Rails Models
- Use delegation selectively: Only delegate methods when it enhances readability or reduces code duplication.
- Limit delegation to a few key methods: Over-delegation can make the model bloated and confusing.
- Use
prefix
to avoid naming conflicts: Adding a prefix keeps things clear and prevents method name collisions. - Handle
nil
values: Useallow_nil
when necessary to prevent errors and make your code more robust. - Consider performance: Avoid delegating frequently called methods that may lead to N+1 query issues. Use caching or eager loading as needed.
FAQs on Rails Model Delegation
Q1: Can I delegate methods to multiple associations?
Yes, you can set up delegation to multiple associations within the same model, but each needs to be set up separately:
class Order < ApplicationRecord
belongs_to :customer
belongs_to :product
delegate :name, to: :customer, prefix: true
delegate :title, to: :product, prefix: true
end
Q2: Is delegate available in all versions of Rails?
Yes, Rails has supported delegate since version 2.3. However, the allow_nil
option was added in Rails 3, so if you’re working on an older Rails project, check the documentation for compatibility.
Q3: How do I debug delegated methods?
If you run into issues, remember that the delegated method is still just a regular method in Rails. You can use puts
, byebug
gem, or Rails console to inspect the delegated call and ensure it’s functioning as expected.
ActiveSupport::Delegate in Rails Applications
Rails extends Ruby’s delegation capabilities with ActiveSupport::Delegate, a module that provides utility methods for delegating method calls to other objects. ActiveSupport’s delegate
method integrates tightly with ActiveRecord and is the go-to choice for most Rails applications.
By using ActiveSupport::Delegate
, you get a number of benefits, such as improved code readability, fewer lines of code, and less manual intervention. It is specifically optimized for Rails applications and works seamlessly with ActiveRecord models, reducing the complexity of managing associations.
Conclusion
Delegation is an essential technique for Rails developers. It allows you to create cleaner, more manageable models by reducing method clutter, making your codebase easier to understand and maintain. While it’s easy to implement, using it effectively requires careful consideration of readability, performance, and code organization.
By following best practices and understanding the options available, you can leverage Rails model delegation to simplify your app’s structure and make your models more intuitive to work with. With these tips in mind, explore delegation in your Rails applications to see how it can improve your code.