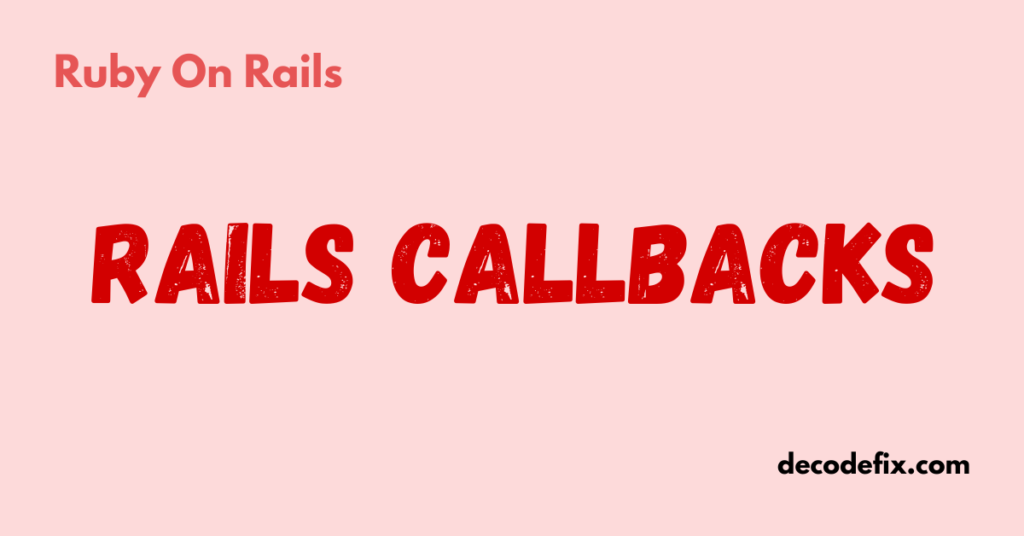
Ruby on Rails, commonly called Rails, is a powerful web development framework. One of the most essential tools it offers developers is callbacks. This blog will explore Rails callbacks in a straightforward, structured way, helping you understand when and why to use them. We’ll break down the different types of callbacks, provide real-world examples, and clarify common issues.
What Are Callbacks in Ruby on Rails?
In Rails, callbacks are methods that get triggered at specific points in an object’s lifecycle. Think of a callback as a trigger that allows you to run specific code when an action occurs, such as before saving a record or after it’s destroyed. Callbacks automate processes, reduce repetitive code, and help manage complex workflows in your application.
Callbacks work with Active Record, the Rails layer that manages database interactions, making it easier to manage these common actions.
Why Use Callbacks?
Callbacks can be helpful when you want to:
- Ensure data integrity
- Automate routine tasks
- Clean up or set up data
- Apply specific conditions based on record states
- Log or monitor actions automatically
For example, a before_save
callback can validate data and prevent a save if certain criteria aren’t met, adding a layer of security to data handling.
Types of Callbacks
Rails offers a range of callbacks, each suited to different stages in an object’s lifecycle. Here’s a list of the most common callback types:
- Before Callbacks
before_validation
before_save
before_create
- After Callbacks
after_validation
after_save
after_create
after_update
after_destroy
- Around Callbacks
around_save
around_create
Before Callbacks
Before callbacks trigger before an action. They’re helpful when you want to prepare or verify data before saving it to the database.
before_validation
Use this callback to modify data right before validation checks. For example:
class User < ApplicationRecord
before_validation :normalize_name
private
def normalize_name
self.name = name.strip.downcase.titleize
end
end
Here, the before_validation
callback ensures the user’s name is stored in a consistent format before the actual validation process begins.
before_save
This callback runs just before saving a record, whether it’s a new or updated one. It’s useful for setting default values or preparing data.
class Post < ApplicationRecord
before_save :set_default_views
private
def set_default_views
self.views ||= 0
end
end
If views
isn’t set when creating a new post, the callback assigns it a default value of 0.
before_create
This callback only runs before creating a new record. Unlikebefore_save
, it doesn’t trigger during updates, making it ideal for actions needed only once.
class Order < ApplicationRecord
before_create :generate_order_number
private
def generate_order_number
self.order_number = SecureRandom.hex(10)
end
end
This ensures that every order has a unique number before it’s saved for the first time.
After Callbacks
After callbacks execute after an action has completed, making them useful for actions that rely on data being saved.
after_save
Useafter_save
when you want something to happen every time a record is saved.
class Profile < ApplicationRecord
after_save :send_update_notification
private
def send_update_notification
NotificationService.send_profile_updated(self)
end
end
Here, the callback sends an email notification whenever a profile is saved.
after_create
Unlikeafter_save
,after_create
only runs when a new record is saved.
class Subscription < ApplicationRecord
after_create :send_welcome_email
private
def send_welcome_email
WelcomeMailer.send_to(user)
end
end
This example triggers a welcome email only when a new subscription is created.
after_update
This callback fires only when an existing record is updated. It’s ideal for tracking changes over time.
class Product < ApplicationRecord
after_update :log_price_change
private
def log_price_change
PriceChangeLogger.log(self)
end
end
after_destroy
Theafter_destroy
callback triggers after a record is deleted, which is useful for cleanup tasks.
class Comment < ApplicationRecord
after_destroy :remove_comment_count
private
def remove_comment_count
post.update(comments_count: post.comments.count)
end
end
Around Callbacks
Around callbacks wrap around an action, running both before and after it. They allow for wrapping code execution, giving you more control over the action flow.
class User < ApplicationRecord
around_save :log_save_time
private
def log_save_time
start_time = Time.now
yield
end_time = Time.now
puts "Save took #{end_time - start_time} seconds"
end
end
In this example, the callback measures and logs the time it takes to save a record.
When to Avoid Rails Callbacks
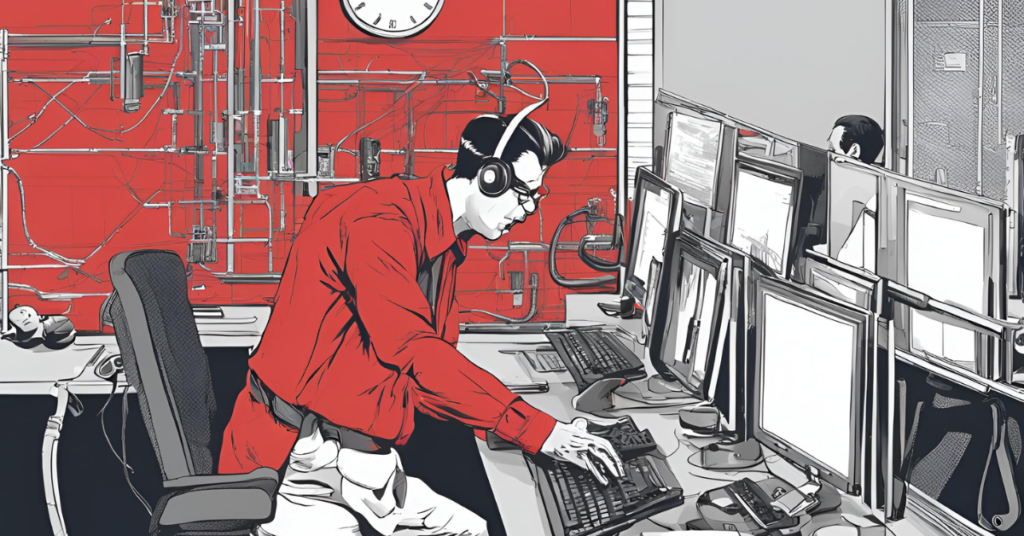
While callbacks offer useful functionality, overusing them can lead to hard-to-debug code. Here are situations where callbacks may not be ideal:
- Complex Logic
If your callback performs complex logic, consider moving it to a service object or background job. - Cross-Table Dependencies
Modifying data across tables with callbacks can create unexpected dependencies, especially if not all tables are kept in sync. - Testing Challenges
Callbacks can make testing more difficult, as they introduce implicit behavior. Whenever possible, simplify your callback logic or isolate it for easier testing.
Using Callbacks with ActiveRecord Transactions
When using callbacks, keep in mind that some will trigger even if an ActiveRecord transaction is rolled back. For instance, after_save
will still run even if the transaction fails later. To avoid this, consider after_commit
or after_rollback
callbacks for actions that should depend on a successful transaction.
class Purchase < ApplicationRecord
after_commit :send_receipt
after_rollback :handle_failed_purchase
private
def send_receipt
ReceiptMailer.send_receipt(self)
end
def handle_failed_purchase
Logger.log("Purchase failed for #{self.id}")
end
end
Rails Callback for Attribute Changes
In some cases, you may want to trigger a callback only when a specific attribute changes. Rails provides an easy way to do this using Active Model Callbacks with conditions.
For example, let’s say you only want to send a notification email when a user updates their email address. You can use the :if
or :unless
options to specify a condition:
class User < ApplicationRecord
after_update :send_email_notification, if: :email_changed?
private
def send_email_notification
UserMailer.email_updated(self).deliver_later
end
end
In this example, the send_email_notification
method is called after the User
object is updated, but only if the email address has changed. The email_changed?
method is a built-in Rails helper that checks whether the email
attribute has changed.
Practical Example: A User Registration Workflow with Callbacks
Imagine you have a user registration system with requirements such as:
- Standardizing usernames
- Sending a welcome email
- Logging the user creation time
Here’s how you could accomplish this with callbacks:
class User < ApplicationRecord
before_validation :standardize_username
after_create :send_welcome_email
after_commit :log_creation_time
private
def standardize_username
self.username = username.strip.downcase
end
def send_welcome_email
WelcomeMailer.welcome(self).deliver_later
end
def log_creation_time
Logger.log("User created at #{created_at}")
end
end
This setup ensures each user’s registration process runs smoothly, with minimal extra coding.
Use Cases for Rails Callbacks
Let’s look at some practical examples where callbacks come in handy:
- Sending Email Notifications
- You might want to send a welcome email after a user has been created. You can define an
after_create
callback to automatically trigger the email once the user record has been saved.
- You might want to send a welcome email after a user has been created. You can define an
- Generating Slugs
- When a user creates a blog post, you might want to generate a URL-friendly slug based on the title. You can use a
before_save
callback to generate the slug just before saving the record.
- When a user creates a blog post, you might want to generate a URL-friendly slug based on the title. You can use a
- Data Normalization
- If you need to format phone numbers or addresses before they are saved to the database, a
before_save
callback is a good place to handle that.
- If you need to format phone numbers or addresses before they are saved to the database, a
- Cascading Deletes
- When deleting a record, you may want to delete associated records as well. A
before_destroy
orafter_destroy
callback can handle this cascade effect.
- When deleting a record, you may want to delete associated records as well. A
Troubleshooting Rails Callbacks
While callbacks are powerful, they can sometimes cause issues. Here are some common problems you might encounter and how to fix them:
1. Callbacks Not Being Triggered
- Problem: A callback is not being executed when expected.
- Solution: Double-check that you’ve defined the callback correctly in the right place (inside the model or controller). Ensure the conditions (e.g.,
if
orunless
) are being met. If usingbefore_validation
orbefore_save
, check that validations and saves aren’t being skipped elsewhere in the code.
2. Callbacks Running Out of Order
- Problem: Callbacks appear to run in the wrong order.
- Solution: Rails executes
before_*
callbacks first andafter_*
callbacks last. If you need more control over the order, you can specify:prepend
to ensure a callback runs before others or use:skip_callback
to skip certain callbacks under specific conditions.
class Post < ApplicationRecord
# First, define a callback to prepend a title change before saving
before_save :prepend_title_change, prepend: true
# Second, define another callback to check content length before saving
before_save :check_content_length
# Third, define a callback to log the update of content before saving
before_save :log_content_update
# Callback to prepend a "Modified:" to the title
def prepend_title_change
self.title = "Modified: #{self.title}"
Rails.logger.info "Title was modified."
end
# Callback to check if content length is too short
def check_content_length
if content.length < 10
errors.add(:content, "Content is too short.")
throw(:abort) # Prevent the save from happening
end
end
# Callback to log content update
def log_content_update
Rails.logger.info "Content was updated."
end
# Method to skip the content length check
def skip_content_length_check
self.class.skip_callback(:save, :before, :check_content_length)
end
end
post = Post.new(title: "Sample Post", content: "Short")
post.skip_content_length_check
post.save
# Output:
# "Title was modified."
# "Content was updated."
# No content length error (since we skipped the callback)
3. Callbacks Causing Performance Issues
- Problem: Callbacks slow down your application.
- Solution: Evaluate if you’re using callbacks too frequently, especially for complex logic. In some cases, moving logic into background jobs (using ActiveJob) can help reduce the load on your application.
4. Callback Interactions with Validations
- Problem: A callback is modifying data, but validations are failing.
- Solution: Remember that callbacks happen before or after validations. Ensure that any modifications made in the callback don’t interfere with validations that happen afterward. Sometimes, re-ordering callbacks or validations can solve this.
Conclusion
Rails callbacks are powerful tools for managing lifecycle events in your models. By automating common processes like data validation, logging, and notifications, callbacks can make your Rails applications more efficient. However, with this power comes responsibility—use callbacks judiciously to avoid complexity and potential debugging headaches. For more complicated workflows, consider service objects or background jobs instead.
Key Takeaway: Use callbacks for essential actions directly tied to model changes, keep them simple, and avoid making them too dependent on other parts of your application. With the right balance, callbacks in Rails can be the key to writing cleaner, more efficient, and automated code.