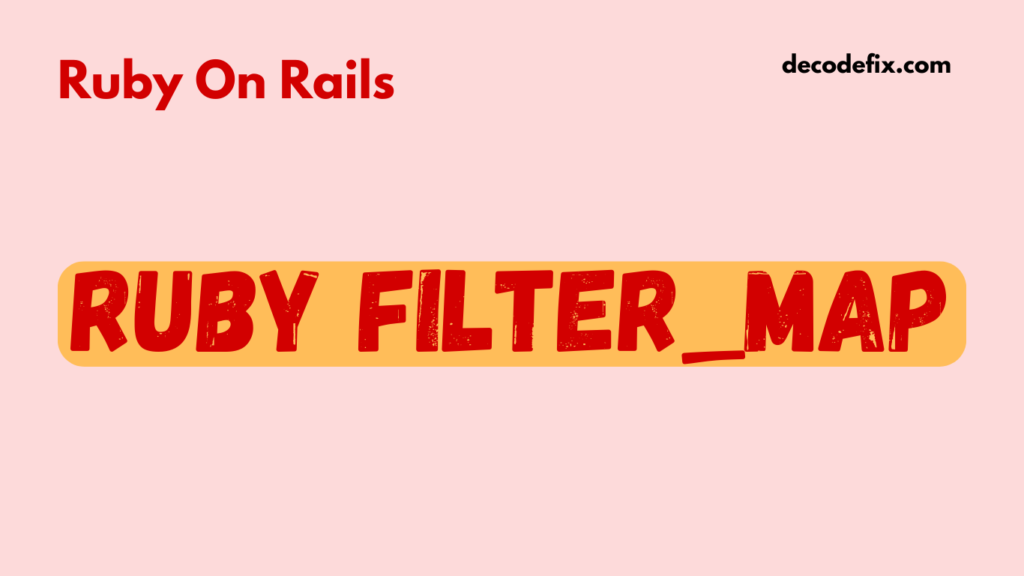
Ruby, as an elegant programming language, offers developers a rich collection of tools to work with arrays and hashes, making data transformation and filtering operations a breeze. Among these tools is the filter_map method, introduced in Ruby 2.7, which quickly gained popularity for its simplicity and effectiveness in combining filtering and mapping in a single step. If you’re a Ruby developer or just curious about optimizing your code, understanding filter_map
is essential.
Let’s dive into the what, why, and how of filter_map
, its difference from similar Ruby methods, and how it can elevate your Ruby coding skills.
What is filter_map in Ruby?
Imagine you’re working with an array in Ruby and need to do two things: filter out specific elements based on a condition and then transform (or “map”) each remaining element to something new. Previously, you’d have to use both select
and map
, running two loops and potentially slowing down your code. Enter filter_map
—Ruby’s answer to doing both operations in one clean, efficient pass.
Simply put, filter_map
combines select
and map
in one step. This means it filters out unwanted elements based on a condition you specify and then applies a transformation to each element that meets the condition. The result? A filtered and mapped array with minimal effort.
How filter_map Works: An Example
Let’s say we have an array of numbers, and we want to get the square of all even numbers:
numbers = [1, 2, 3, 4, 5, 6]
# Using filter_map
even_squares = numbers.filter_map { |n| n**2 if n.even? }
# => [4, 16, 36]
In this example, filter_map
filters for even numbers (by checking n.even?
) and maps each of them to their square (n**2
). It’s concise, efficient, and easy to read.
Why Use filter_map?
Using filter_map
makes your code cleaner and more efficient. By combining filtering and mapping in a single method, it:
- Reduces complexity in your code.
- Improves readability by minimizing nested methods.
- Enhances performance, as the array is only looped through once.
This streamlined approach not only makes your code look better but also makes it faster and easier to understand.
How to Use filter Method in Ruby on Rails?
Before filter_map
, many developers relied on the filter
(or select
) method for filtering operations. filter
simply removes elements that don’t meet a specified condition but does not transform them.
Using filter in Action
Let’s revisit the example above, but this time only filtering out even numbers:
even_numbers = numbers.filter { |n| n.even? }
# => [2, 4, 6]
The filter
method returns all even numbers but doesn’t perform any transformation. If you want to transform each element after filtering, you’d need to chain map
, which means two passes through the array:
even_squares = numbers.filter { |n| n.even? }.map { |n| n**2 }
# => [4, 16, 36]
filter_map
combines these two steps into one, saving time and increasing efficiency. Not only is this cleaner, but it’s also faster—especially with larger data sets.
Difference Between map method and select in Ruby
Before diving into filter_map
, it’s essential to understand how map
and select
(or filter
) work independently in Ruby.
map
: This method goes through each element in an array and applies a transformation. It doesn’t remove or filter any element; it simply returns a new array based on the results of the block.rubyCopy codesquared_numbers = numbers.map { |n| n**2 } # => [1, 4, 9, 16, 25, 36]
select
(orfilter
): This method is used to filter elements that match a specific condition but doesn’t transform them.
even_numbers = numbers.select { |n| n.even? } # => [2, 4, 6]
To get the behavior of filter_map
without actually using it, you would need to chain select
and map
:
even_squares = numbers.select { |n| n.even? }.map { |n| n**2 }
# => [4, 16, 36]
But this requires two passes through the array. filter_map
performs both actions in a single pass, making it more efficient for scenarios where you want to both filter and transform.
filter_map vs map method: What’s the Difference?
While map
and filter_map
might look similar because they both involve transformations, they serve different purposes:
- With
map
, every element is transformed. You’re not discarding any values. - With
filter_map
, only elements that meet a certain condition are transformed. Those that don’t meet the condition are excluded.
Here’s a quick example to illustrate:
names = ["Alice", "Bob", "Charlie", nil, "David", nil]
# Using map
name_lengths = names.map { |name| name&.length }
# => [5, 3, 7, nil, 5, nil]
# Using filter_map
valid_name_lengths = names.filter_map { |name| name&.length if name }
# => [5, 3, 7, 5]
The filter_map
version excludes nil
values, producing a cleaner, more focused result. This can be especially useful when working with data that might contain nil
values or other “falsy” entries.
Handling undefined method ‘filter_map’ in Ruby
If you encounter an error like undefined method 'filter_map'
, it likely means your Ruby version is older than 2.7. Since filter_map
was introduced in Ruby 2.7, you’ll need to update your Ruby installation to use it. Alternatively, you can achieve similar results by chaining select
and map
as shown above, though it may be less efficient.
To update Ruby, you might use a version manager like rbenv
or rvm
. Once you have Ruby 2.7 or higher, filter_map
should work seamlessly.
filter_map vs reduce: When to Use Each in Ruby
Another common Ruby method is reduce
(or inject
), which is used for accumulating or aggregating values across an array. While filter_map
focuses on filtering and transforming, reduce
is more about summarizing or combining elements.
Example: Using reduce to Sum Values
If you wanted to sum all the numbers in an array, reduce
would be your go-to method:
sum = numbers.reduce(0) { |acc, n| acc + n }
# => 21
Combining filter_map with reduce
You can use filter_map
to first filter and transform data, and then use reduce
to aggregate the results. Let’s say we want to sum the squares of all even numbers:
even_square_sum = numbers.filter_map { |n| n**2 if n.even? }.reduce(:+)
# => 56
This approach is powerful because it allows for a highly customized chain of operations, combining filtering, transforming, and aggregating all in a single, readable line.
When to Choose filter_map over Other Methods
Knowing when to use filter_map
can make your code more efficient and readable. Here are some situations where it shines:
- When you need to filter and transform in one pass.
filter_map
saves you from chainingselect
andmap
. - When working with large datasets. With big data, efficiency counts. Since
filter_map
avoids an extra loop, it’s more performant. - When avoiding
nil
values is important. Sincefilter_map
inherently skipsnil
values, it’s a natural choice for “clean” data transformations.
Real-World Example: Using filter_map in a Web Application
Let’s say you’re building a simple blog and want to display only published articles with a specific keyword in the title. Here’s how you might use filter_map
to achieve this efficiently:
articles = [
{ title: "Ruby on Rails Basics", published: true },
{ title: "Advanced Ruby", published: false },
{ title: "Ruby filter_map Tutorial", published: true }
]
ruby_articles = articles.filter_map do |article|
article[:title] if article[:published] && article[:title].include?("Ruby")
end
# => ["Ruby on Rails Basics", "Ruby filter_map Tutorial"]
This single line of code filters for published articles with “Ruby” in the title and returns only the titles, which can then be displayed on your site.
Example: Using filter_map with Enums in Ruby
Suppose we have a Task
model with a status
enum. The status
can be either pending
, completed
, or archived
. We want to get a list of titles for all completed tasks.
Step 1: Define the Enum in the Model
class Task < ApplicationRecord
enum status: { pending: 0, completed: 1, archived: 2 }
end
Step 2: Use filter_map to Get Titles of Completed Tasks
tasks = Task.all
completed_titles = tasks.filter_map { |task| task.title if task.completed? }
puts completed_titles
# Example Output: ["Finish report", "Review code", "Write documentation"]
Explanation:
tasks.filter_map
iterates through each task.task.completed?
checks if the task’s status iscompleted
.- If the task is completed,
filter_map
addstask.title
to the new array; otherwise, it skips it.
This simple example shows how filter_map
can efficiently filter and transform collections in a single step.
Performance: Is filter_map Really Faster?
When you use select
and map
separately, Ruby iterates over the array twice. With filter_map
, the iteration happens only once. This can improve performance, especially with large datasets.
Here’s a quick benchmark comparison:
require 'benchmark'
numbers = (1..10_000).to_a
Benchmark.bm do |x|
x.report("select + map:") { numbers.select { |n| n.even? }.map { |n| n**2 } }
x.report("filter_map:") { numbers.filter_map { |n| n**2 if n.even? } }
end
For small arrays, the difference may not be noticeable. But with larger arrays, filter_map
usually proves to be faster.
When Shouldn’t You Use filter_map?
While filter_map
is convenient, it’s not always the right choice. Here are a few cases where it might be better to stick with select
and map
:
- Complex Filtering Logic: If your filtering condition is very complex or has multiple steps, splitting the logic between
select
andmap
can make the code more readable. - Separate Transformation Requirements: If the filtering and transformation steps need to be distinct for readability or debugging, using two methods might be clearer.
- Readability Concerns: If your team is not familiar with
filter_map
, it might be beneficial to use separate methods for clarity.
Conclusion
The filter_map
method in Ruby is a versatile tool that combines the functionality of select
and map
in a single, powerful pass. It’s especially useful when working with datasets where you need to filter and transform elements efficiently. Whether you’re building a small application or handling large amounts of data, filter_map
is a method that can enhance both the readability and performance of your Ruby code.
With this guide, you should have a solid understanding of how filter_map
works, when to use it, and how it stacks up against similar methods like map
, select
, and reduce
. Embrace filter_map
to streamline your code and make your code more concise and responsive.