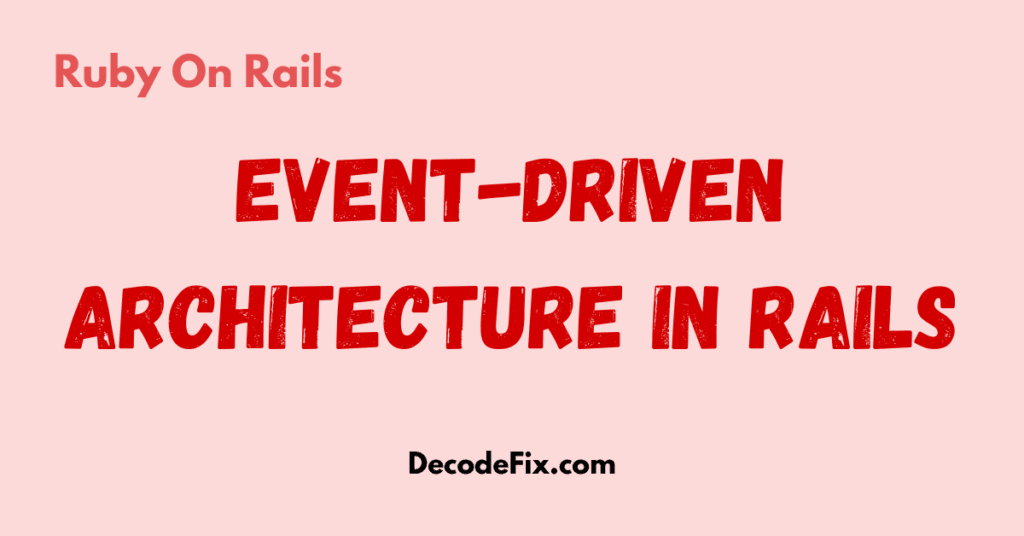
Modern software systems face increasing demands for scalability, flexibility, and real-time responsiveness. Event-driven architecture (EDA) is a design paradigm that meets these needs by organizing application logic around events—actions or state changes that trigger subsequent workflows. For Ruby on Rails developers, EDA offers an opportunity to build systems that are modular, asynchronous, and highly scalable.
In this blog, we’ll delve into the principles of EDA, why it’s beneficial in Ruby on Rails applications, and a step-by-step guide to implementing it effectively. Along the way, we’ll explore tools, real-world use cases, and best practices to help you get the most out of this powerful architecture.
What is Event-Driven Architecture?
At its core, event-driven architecture structures application workflows around events. These events, generated by actions like user input or system state changes, serve as the trigger for subsequent processes. EDA decouples producers (event generators) and consumers (event handlers), ensuring systems remain flexible and scalable.
Key Components of Event-Driven Architecture:
- Events: Indicators of state changes, such as “user_signed_up” or “order_placed.”
- Producers: Emit events when significant actions occur.
- Consumers: Listen for specific events and act on them.
- Event Bus: The mediator that facilitates communication between producers and consumers.
Benefits of Event-Driven Architecture in Rails
Ruby on Rails applications traditionally follow a monolithic structure. While this simplifies development for smaller projects, it becomes a bottleneck as complexity grows. Adopting EDA in Rails can address these challenges:
- Decoupling Components: Producers and consumers work independently, reducing interdependencies.
- Improved Scalability: Asynchronous processing enables the system to handle higher traffic loads without slowing down.
- Flexibility in Development: Adding new features or modifying existing ones is less risky and more straightforward.
- Real-Time Responsiveness: EDA is ideal for handling real-time notifications, updates, and alerts.
- Resilience: Failures in one component don’t directly affect others, improving system robustness.
When to Use Event-Driven Architecture
EDA isn’t suitable for every application. It shines in scenarios where:
- Asynchronous Processing is Critical: For example, sending notifications, processing payments, or updating inventory.
- Real-Time Data Handling is Needed: Applications like chat systems, live feeds, or IoT devices.
- High Scalability is a Requirement: Systems handling millions of users or transactions daily.
Step-by-Step Guide to Implementing EDA in Ruby on Rails
Let’s break down how to implement EDA in your Rails application:
Step 1: Identify Events
Begin by pinpointing the actions in your application that could generate events. These might include:
- User registration
- Order placement
- Inventory updates
- Payment processing
Each of these represents a point where decoupling logic can enhance performance.
Step 2: Create an Event Class
Define a lightweight class to represent events. This ensures a standard structure for event data.
class Event
attr_reader :name, :payload
def initialize(name, payload = {})
@name = name
@payload = payload
end
end
Here, name
identifies the event type, while payload
contains any relevant data.
Step 3: Implement an Event Bus
The event bus routes events from producers to consumers. You can implement a simple event bus in Rails or use an existing gem like Wisper.
class EventBus
@subscribers = {}
def self.subscribe(event_name, handler)
@subscribers[event_name] ||= []
@subscribers[event_name] << handler
end
def self.publish(event_name, payload)
(@subscribers[event_name] || []).each do |handler|
handler.call(payload)
end
end
end
This allows multiple consumers to handle the same event without interfering with each other.
Step 4: Publish Events
Publish events from producers when key actions occur. For example, after a user signs up:
class User < ApplicationRecord
after_create :emit_signup_event
private
def emit_signup_event
EventBus.publish('user.signup', { user_id: id, email: email })
end
end
Step 5: Create Event Consumers
Consumers subscribe to events and execute tasks. For instance, sending a welcome email:
class SendWelcomeEmail
def self.call(payload)
UserMailer.welcome_email(payload[:email]).deliver_later
end
end
EventBus.subscribe('user.signup', SendWelcomeEmail)
Step 6: Add Background Jobs
To handle long-running tasks asynchronously, integrate a background job framework like Sidekiq.
class NotifyAdminJob < ApplicationJob
queue_as :default
def perform(payload)
AdminNotifier.notify(payload[:user_id])
end
end
EventBus.subscribe('user.signup', ->(payload) { NotifyAdminJob.perform_later(payload) })
Step 7: Scale with External Tools
For distributed systems, you can use message brokers like RabbitMQ, Kafka, or Redis Streams for reliable and scalable event handling.
Real-World Applications of Event-Driven Architecture
- E-commerce:
- Producers: User actions like adding items to cart or placing orders.
- Consumers: Updating inventory, sending notifications, or generating invoices.
- Social Media:
- Producers: Posting, liking, or commenting on content.
- Consumers: Updating activity feeds or notifying users.
- Finance:
- Producers: Transactions or account updates.
- Consumers: Fraud detection, sending alerts, or generating reports.
Challenges and How to Overcome Them
Common Challenges
- Event Duplication: Consumers might process the same event multiple times.
- Solution: Ensure consumers are idempotent.
- Debugging: Tracing asynchronous workflows can be difficult.
- Solution: Use monitoring tools like Honeybadger or Sentry.
- Complexity: EDA can add overhead for simple systems.
- Solution: Start with small, manageable use cases.
Best Practices of Event-Driven Architecture
- Use Clear Event Names: Stick to a convention like
resource.action
(e.g.,user.signup
). - Document Events: Maintain an event registry for easy reference.
- Monitor Performance: Use tools like New Relic to track event handling.
- Write Tests: Ensure producers and consumers work seamlessly.
Conclusion
Event-driven architecture brings a wealth of benefits to Ruby on Rails applications, including scalability, flexibility, and resilience. By structuring your app around events, you can build systems that are prepared to handle the demands of modern, high-traffic environments.
Start with small implementations and expand as your understanding grows. Tools like Sidekiq, RabbitMQ, and Redis can help you scale while maintaining efficiency.
Adopting EDA isn’t just a technical upgrade—it’s a strategic investment in your application’s future.