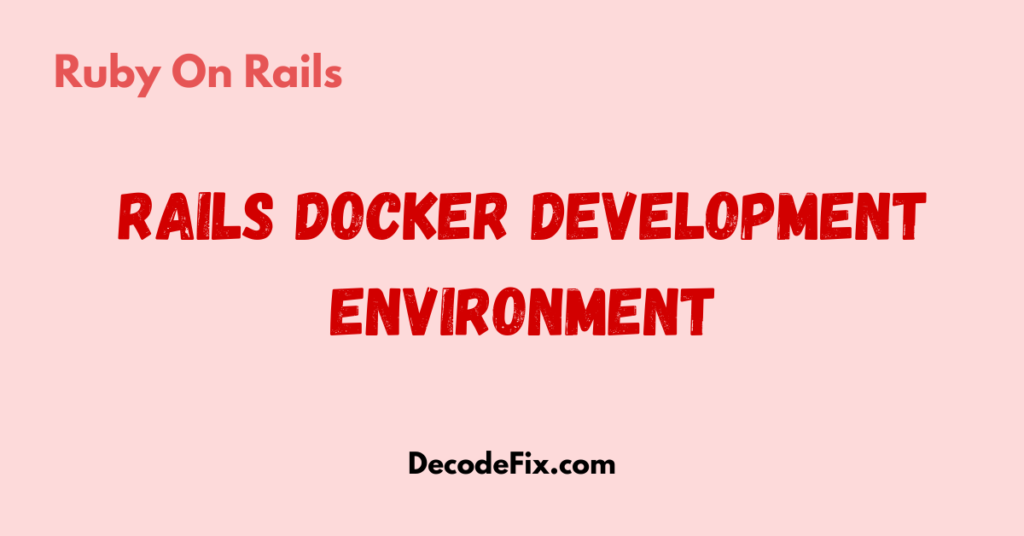
Setting up a Ruby on Rails Docker development environment is simpler than you think. With Docker, you can eliminate system-specific issues and ensure your app works the same everywhere. Whether you’re a Rails newbie or a seasoned developer, Docker offers a flexible way to containerize your app and streamline development. Let’s walk through creating a seamless Dockerized Rails setup.
What is Docker, and Why Should Rails Developers Care?
Docker is a tool that packages applications and their dependencies into lightweight containers. Think of a container as a virtual box that holds everything your Rails app needs to run—Ruby, gems, PostgreSQL, and more. By using Docker, you avoid the infamous “It works on my machine!” problem.
Imagine collaborating with a team where everyone has different operating systems or Ruby versions. With Docker, you can sidestep those compatibility headaches. Plus, deploying Rails apps becomes smoother since production mirrors your local environment.
Step 1: Prepare Your Rails App for Docker
First things first, create or open your Rails application. If you don’t have one, generate a new app.
rails new my_dockerized_app -d postgresql
cd my_dockerized_app
We’re using PostgreSQL as the database here. You can substitute this with MySQL or SQLite if you prefer.
Step 2: Add a Dockerfile
The Dockerfile tells Docker how to build your app’s image. In the root of your app, create a file named Dockerfile
. Here’s an example:
# Base image
FROM ruby:3.1
# Set the working directory
WORKDIR /app
# Install dependencies
RUN apt-get update -qq && apt-get install -y nodejs postgresql-client yarn
# Copy the Gemfile and install gems
COPY Gemfile Gemfile.lock ./
RUN bundle install
# Copy the rest of the app
COPY . .
# Expose the default Rails port
EXPOSE 3000
# Start the Rails server
CMD ["rails", "server", "-b", "0.0.0.0"]
This Dockerfile does several things:
- It uses Ruby 3.1 as the base image.
- Sets up Node.js, PostgreSQL client, and Yarn.
- Installs your gems and copies the app’s code.
Step 3: Add a docker-compose.yml File
The docker-compose.yml
file simplifies running multi-container applications. You’ll need a database container alongside your Rails app. Create a docker-compose.yml
file in your app’s root directory:
version: '3.8'
services:
web:
build: .
command: bundle exec rails server -b 0.0.0.0 -p 3000
volumes:
- .:/app
ports:
- "3000:3000"
depends_on:
- db
db:
image: postgres:14
environment:
POSTGRES_USER: postgres
POSTGRES_PASSWORD: password
POSTGRES_DB: my_dockerized_app_development
ports:
- "5432:5432"
Here’s what’s happening:
- The web service builds your Rails app.
- The db service runs a PostgreSQL container.
Step 4: Update database.yml for Docker
Your Rails app needs to connect to the database running inside a container. Update your config/database.yml
:
default: &default
adapter: postgresql
encoding: unicode
host: db
username: postgres
password: password
pool: 5
development:
<<: *default
database: my_dockerized_app_development
test:
<<: *default
database: my_dockerized_app_test
Notice the host: db
. This matches the service name in docker-compose.yml
.
Step 5: Build and Run Your Containers
You’re ready to fire up Docker! Run these commands:
docker-compose build
docker-compose up
The first command builds your containers, and the second one starts them. Open your browser and visit http://localhost:3000. You should see the Rails welcome page.
Common Errors and How to Fix Them
1. Precompiling Assets in Production Mode
If you plan to deploy using Docker, add this line to your Dockerfile to precompile assets:
RUN bundle exec rake assets:precompile
2. Permission Errors on Linux
Linux users may encounter file permission errors. Fix this by adding the --user
flag when running commands:
docker-compose run --user "$(id -u):$(id -g)" web rails db:create
3. Database Connection Issues
Ensure your POSTGRES_USER
and POSTGRES_PASSWORD
in docker-compose.yml
match those in database.yml
.
Optimizing Your Dockerized Rails Setup
Consider adding a docker-compose.override.yml
file for development. This keeps your development workflow fast and efficient:
version: '3.8'
services:
web:
volumes:
- .:/app
- bundle_cache:/usr/local/bundle
volumes:
bundle_cache:
This setup caches your gems, speeding up builds.
Why Docker Is a Game-Changer for Rails Development
Docker simplifies dependency management and boosts productivity. No more struggling with mismatched Ruby or Node.js versions. It also enables seamless collaboration across teams.
For instance, a 2023 survey showed 65% of developers prefer Docker for local development. Its popularity continues to grow as teams embrace containerization for its consistency and portability.
Final Thoughts
Docker transforms how you develop Rails applications. From eliminating environment issues to making deployments predictable, the benefits are undeniable. Give this setup a spin, and you’ll wonder how you ever worked without it.
Feel stuck? Drop a comment below! Let’s solve it together.