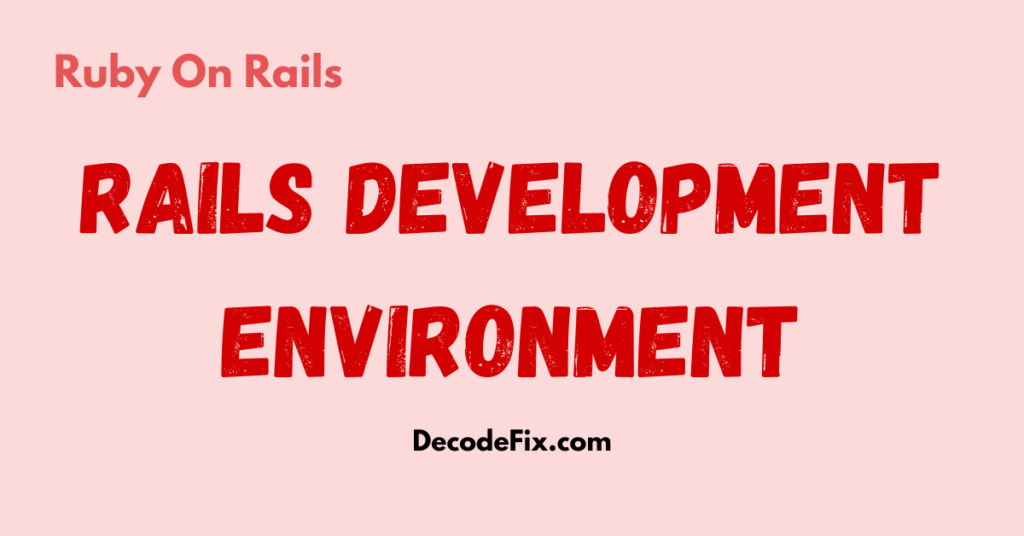
Ruby on Rails, often called Rails, is a powerful web development framework that simplifies coding and speeds up development. Setting up a Rails development environment can seem tricky at first, but with this guide, you’ll get started quickly and confidently. Let’s break down the process step by step.
Why Choose Ruby on Rails?
Rails combines simplicity with power. Its convention-over-configuration approach minimizes repetitive tasks, making it perfect for startups and established projects alike. Companies like GitHub, Shopify, and Airbnb rely on Rails for their applications. If it’s good enough for them, it’s worth your attention!
Prerequisites for Ruby on Rails Development
Before diving in, make sure your system meets these requirements:
- Operating System: macOS, Linux, or Windows (using WSL2)
- Text Editor or IDE: VS Code, RubyMine, or Sublime Text
- Terminal Access: Command Prompt, Terminal, or Git Bash
- Basic Knowledge of Ruby and Command Line
Got that? Awesome. Let’s get started.
Step 1: Install Ruby
Ruby is the heart of Rails, so you’ll need it first.
On macOS
- Open the terminal.
- Install Homebrew by running:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
- Install Ruby:
brew install ruby
On Linux
- Update your package list:
sudo apt update
- Install Ruby:
sudo apt install ruby-full
On Windows
- Install Windows Subsystem for Linux (WSL2).
- Install Ruby via a Linux distribution, like Ubuntu:
sudo apt install ruby-full
To confirm the installation, type:
ruby -v
You should see the installed Ruby version.
Step 2: Install a Ruby Version Manager
Using a Ruby version manager like RVM or rbenv ensures you can manage multiple Ruby versions easily.
Installing rbenv
git clone https://github.com/rbenv/rbenv.git ~/.rbenv
cd ~/.rbenv && src/configure && make -C src
Then install ruby-build:
git clone https://github.com/rbenv/ruby-build.git ~/.rbenv/plugins/ruby-build
Why Use a Version Manager?
It simplifies switching between projects requiring different Ruby versions.
Step 3: Install Rails
Now for the star of the show! Once Ruby is ready, installing Rails is straightforward.
Command to Install Rails
Run:
gem install rails
To verify, check the version:
rails -v
You’re halfway there!
Step 4: Set Up a Database
Rails works best with a database. PostgreSQL is a popular choice, but SQLite is great for beginners.
Installing SQLite (Default)
macOS or Linux:
sudo apt install sqlite3 libsqlite3-dev
For Windows, SQLite is pre-installed with Rails.
Step 5: Install Node.js and Yarn
Rails needs a JavaScript runtime. Node.js and Yarn handle JavaScript assets.
Install Node.js
- Visit Node.js and download the LTS version.
- Install via the installer.
Install Yarn
npm install --global yarn
Step 6: Create Your First Rails App
Let’s build something real!
- Open your terminal.
- Run:
rails new my_first_app
- Navigate to the app folder:
cd my_first_app
- Start the Rails server:
rails server
- Visit
http://localhost:3000
in your browser.
Voilà! Your first Rails app is live.
Common Pitfalls and How to Avoid Them
- Dependency Errors: Ensure you’re using compatible Ruby and Rails versions.
- JavaScript Runtime Missing: Double-check Node.js installation.
- Database Connection Issues: Use
rails db:setup
for configuration.
Optimizing Your Development Environment
Use a Git Version Control System
Version control helps track code changes. Install Git:
sudo apt install git
Set up your name and email:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Choose an Editor with Ruby Plugins
Install plugins for VS Code or RubyMine to enhance productivity.
Debugging Made Easy
Use the byebug
gem for debugging Rails apps.
Frequently Asked Questions
Q: Can I skip installing rbenv or RVM?
Yes, but managing Ruby versions manually is harder.
Q: What’s the best database for Rails?
PostgreSQL is robust for production. SQLite is fine for beginners.
Q: Can I use Rails without a database?
Yes! Rails can work for API-only projects where a database isn’t required.
Q: Which operating system is best for Rails?
Rails works well on all major operating systems. However, many developers prefer macOS or Linux for their developer-friendly tools.
Q: How do I uninstall Rails?
Use the command gem uninstall rails
.
Real-World Example: Rails in Action
Imagine building a to-do app. Within hours, Rails helps you set up routes, controllers, and views. Add a database, and you’re storing tasks!
Pro tip: Use scaffolding for quick app generation. Run:
rails generate scaffold Task title:string completed:boolean
Keeping Your Environment Up-to-Date
Update Gems
Regularly run:
bundle update
Upgrade Rails
Stay current with Rails versions to access new features.
Resources to Learn Rails Faster
- Rails Guides: https://guides.rubyonrails.org/
- Ruby on Rails Podcasts: Perfect for learning on the go.
- Codecademy’s Ruby Course: Ideal for beginners.
Conclusion
Setting up a Ruby on Rails development environment is like learning to ride a bike. Once you grasp the basics, you’ll create apps with ease. Follow this guide step by step, and you’ll have a functional environment in no time.
Rails has stood the test of time for a reason—simplicity, power, and speed. Whether you’re a beginner or an experienced developer, Rails can supercharge your web development journey.
Happy coding!