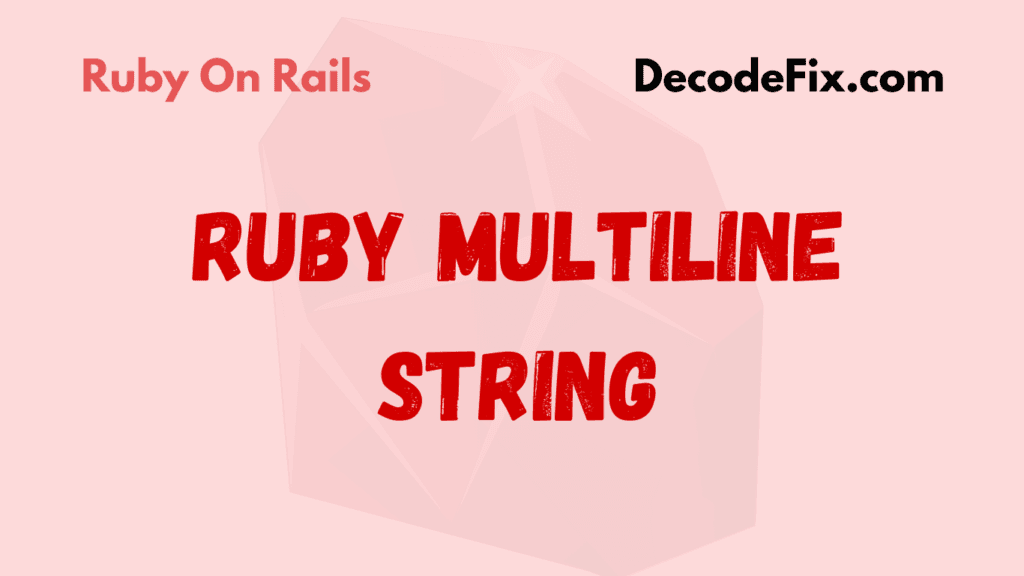
Multiline strings are a core feature of Ruby that allows you to work with large text blocks in a clean and organized way. Whether you’re crafting templates, embedding HTML, or logging data, multiline strings streamline the process. For Rails developers, handling them efficiently can improve readability, maintainability, and debugging. But which technique should you use? Let’s explore the top 5 methods for handling multiline strings effectively in Ruby.
1. HEREDOC: The Clean and Readable Approach
What is HEREDOC in Ruby?
HEREDOC is a syntax that lets you create multiline strings without worrying about escape characters or concatenation. It starts with <<
followed by a delimiter, which ends with the same delimiter on a new line.
html_template = <<HTML
<!DOCTYPE html>
<html>
<head>
<title>My Page</title>
</head>
<body>
<h1>Welcome to Ruby on Rails!</h1>
</body>
</html>
HTML
puts html_template
Indented HEREDOC with <<~
HEREDOC supports indentation with <<~
, which removes leading whitespace.
query = <<~SQL
SELECT * FROM users
WHERE created_at >= '2024-01-01';
SQL
puts query
Pros and Cons of Using HEREDOC
Pros:
- Cleaner syntax for large text blocks.
- Easy to embed code or HTML.
Cons:
- Harder to debug if delimiters are mismatched.
- Not ideal for dynamic content.
2. Concatenation: Combining Strings Line by Line
Understanding String Concatenation
Concatenation joins strings using the +
operator or backslashes for continuation.
paragraph = "Ruby on Rails is a powerful framework " +
"that simplifies web development. " +
"It promotes convention over configuration."
puts paragraph
For multiline strings, backslashes allow continuation:
description = "This is a description " \
"spanning multiple lines " \
"for better readability."
puts description
When to Use Concatenation for Multiline Strings
Concatenation is useful when creating strings dynamically in loops or conditional statements. However, it can reduce readability for larger blocks of text.
Check out our article on filter_map
for optimizing loops and conditions in your code.
3. String Interpolation: Adding Dynamic Content
What is String Interpolation?
String interpolation lets you embed Ruby expressions inside strings using #{}
.
name = "Alice"
greeting = "Hello, #{name}!"
puts greeting
Using Interpolation in Multiline Strings
Combine interpolation with HEREDOC or arrays for dynamic multiline strings.
user_name = "Bob"
message = <<~TEXT
Hi #{user_name},
Welcome to our platform!
Let us know how we can assist you.
Best regards,
The Team
TEXT
puts message
Limitations of Interpolation
- Overusing interpolation can make code harder to read.
- Values must be valid Ruby expressions.
4. Arrays and join: A Modular Alternative
Breaking Down Multiline Strings into Arrays
This technique involves creating an array of strings and using join
to combine them.
lines = [
"Line 1 of the message.",
"Line 2 provides additional details.",
"Line 3 concludes the message."
]
multiline_string = lines.join("\n")
puts multiline_string
Advantages of Using Arrays
- Modular and easy to modify.
- Ideal for dynamically generating content.
Use Case
Useful in scenarios like generating email templates or logs.
5. Inline Block Strings: Using Backslashes and Escape Characters
What Are Inline Block Strings?
Inline block strings use escape characters like \n
for newlines or backslashes (\
) for continuation.
log_message = "User logged in successfully.\n" \
"IP Address: 192.168.0.1\n" \
"Timestamp: 2024-12-01 14:30:00"
puts log_message
Use Cases for Inline Block Strings
- Compact logging.
- Generating formatted CLI outputs.
Tips for Choosing the Right Technique
Factors to Consider
- Readability: HEREDOC or arrays work best.
- Performance: Concatenation is faster for small strings.
- Dynamic Content: Use interpolation or arrays.
Comparing the Techniques
Technique | Readability | Flexibility | Use Case Example |
---|---|---|---|
HEREDOC | High | Moderate | Embedding HTML or templates |
Concatenation | Moderate | Low | Dynamic small strings |
Interpolation | High | High | Personalized messages |
Arrays + join | High | High | Modular text generation |
Inline Block | Low | Moderate | Logging |
Common Pitfalls to Avoid When Working with Multiline Strings
- Mismatched Delimiters in HEREDOC
- Ensure starting and ending delimiters are consistent.
- Improper Escape Characters
- Avoid unintended newlines or spacing issues.
- Overcomplicating Interpolation
- Use it sparingly to maintain readability.
Conclusion
Mastering these techniques empowers you to write cleaner, more maintainable, and efficient Ruby code. HEREDOC stands out for its readability, making it a great choice for embedding large text blocks like templates or SQL queries. Arrays combined with join
offer unparalleled modularity, making dynamic content generation straightforward and organized. String interpolation adds a layer of flexibility for creating personalized and dynamic messages, while concatenation and inline-block strings provide compact solutions for simpler tasks like logging or formatting output. By understanding the strengths and limitations of each method, you can select the approach that best fits your project’s requirements and your coding style, ensuring your Ruby code remains readable, efficient, and easy to manage.