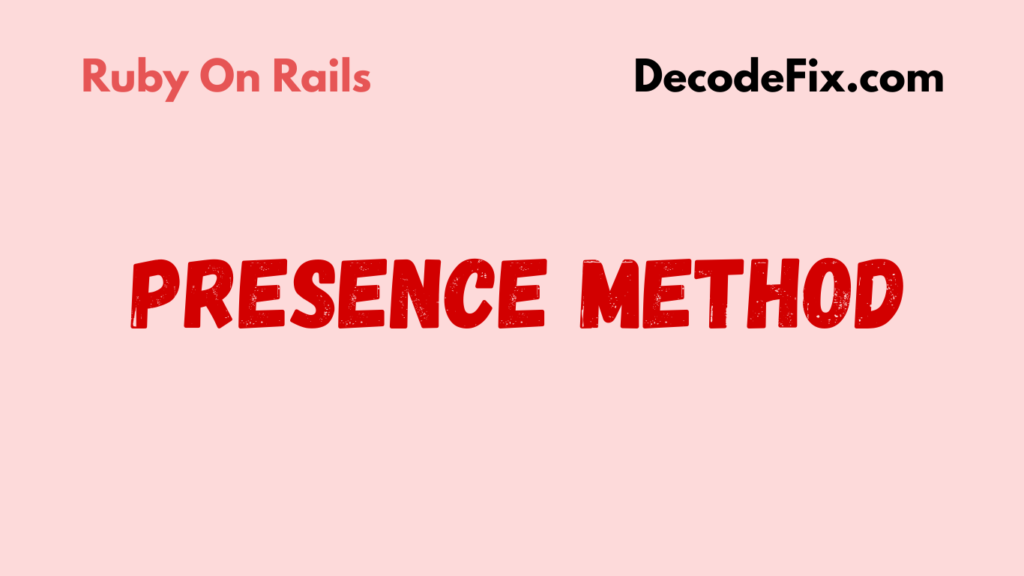
Handling nil
values and empty objects in Ruby on Rails can sometimes lead to clunky, verbose code. Enter the presence method, a gem of Rails that often flies under the radar. It offers an elegant way to manage emptiness in objects, simplify conditional checks, and make your code shine like a well-polished gem.
In this blog, we’ll explore what the presence method is how it works, and—more importantly—when and why you should use it in your Rails applications. Whether you’re a Rails newbie or a seasoned developer looking to clean up your codebase, this guide has you covered.
What Is the Presence Method?
The presence
method is a simple yet powerful addition to Rails. It checks whether an object is present (not nil
, false
, or blank). If the object is present, it returns the object itself; otherwise, it returns nil
.
Here’s the formal definition:
object.presence
If object.present?
is true, it returns the object. If object.blank?
is true, it returns nil
.
Example:
name = ""
default_name = "Guest"
# Using presence for fallback
final_name = name.presence || default_name
puts final_name # Output: "Guest"
Key Takeaway:
Instead of writing verbose conditionals like this:
final_name = name && !name.blank? ? name : default_name
…you can use presence
to achieve the same result with far more readability.
How Does the Presence Method Work Internally?
The presence
method is part of Rails’ ActiveSupport module. Here’s its internal implementation:
def presence
self if present?
end
This one-liner leverages the present?
method, which checks if an object is neither nil
nor blank. The presence
method simply returns the object if it passes this test.
Why Use the Presence Method?
1. Cleaner Code with Fallback Values
A common use case for presence
is to provide default values when a variable is empty.
Without presence:
email = params[:email]
final_email = email.blank? ? "example@example.com" : email
With presence:
final_email = params[:email].presence || "example@example.com"
Why it matters: The second version is shorter, clearer, and more expressive.
2. Chaining Methods Safely
When working with nested objects or chains, you often check for nil
or blank values to avoid exceptions.
Example:
user_city = user.address.city if user.address.present?
This can be simplified:
user_city = user.address.presence&.city
Key Benefit: You avoid multiple if
statements or guards, making the code compact.
3. ActiveRecord Query Parameters
In Rails, ActiveRecord queries often handle optional filters. Instead of manually checking each parameter for emptiness, use presence
.
Example:
# Filtering only when params[:status] is present
scope = Post.where(status: params[:status].presence)
Why it’s awesome: You dynamically include filters without cluttering your query logic.
4. Preprocessing User Input
User-submitted forms often include empty strings for optional fields. Instead of cluttering your controller logic, clean up values with presence
.
Example:
# Before saving the user
params[:nickname] = params[:nickname].presence || "Anonymous"
When Should You Avoid Presence?
While presence
is handy, but it’s not always the best tool for the job. Here are some scenarios where caution is needed:
- Boolean Values:
Sincefalse.presence
returnsnil
, avoid usingpresence
with boolean logic.value = false value.presence || true # Returns true, which might not be intended
- Complex Chains:
If your logic involves deeply nested objects or custom conditions, explicitly checking forblank?
might be clearer for maintainability.
Real-World Example: Dynamic Search Filters
Suppose you’re building a blog platform (like DecodeFix, wink). Users can filter posts by category, status, or author, but the filters are optional.
Here’s how you might handle it with presence
:
Without presence:
scope = Post.all
scope = scope.where(category: params[:category]) unless params[:category].blank?
scope = scope.where(status: params[:status]) unless params[:status].blank?
scope = scope.where(author: params[:author]) unless params[:author].blank?
With presence:
scope = Post.all
scope = scope.where(category: params[:category].presence)
scope = scope.where(status: params[:status].presence)
scope = scope.where(author: params[:author].presence)
Result: The second version is shorter, easier to read, and easier to maintain.
Fun Fact: The Presence Method Outside Rails
Though presence
is a Rails method, it can be useful in plain Ruby too. By adding Rails’ ActiveSupport gem to a standalone Ruby project, you can use it in scripts and tools.
Example in a Ruby CLI Tool:
require 'active_support/core_ext/object/blank'
username = ENV['USERNAME'].presence || "default_user"
puts "Welcome, #{username}!"
Common Pitfalls with Presence
- Confusing Behavior with
nil
andfalse
:
Remember,false.presence
is alwaysnil
. Don’t rely on presence for boolean checks. - Overusing for Readability:
Whilepresence
simplifies code, overusing it in every situation can make logic less explicit. Sometimes, clarity should win over brevity.
Conclusion: When to Reach for Presence
The presence
method is your go-to tool in these situations:
- You need a fallback value for empty strings or objects.
- You’re filtering query parameters dynamically.
- You’re handling user input that might be empty.
- You want cleaner, more readable code.
By incorporating presence
in your Ruby on Rails projects, you’ll not only write better code but also save yourself from future headaches when revisiting old projects.