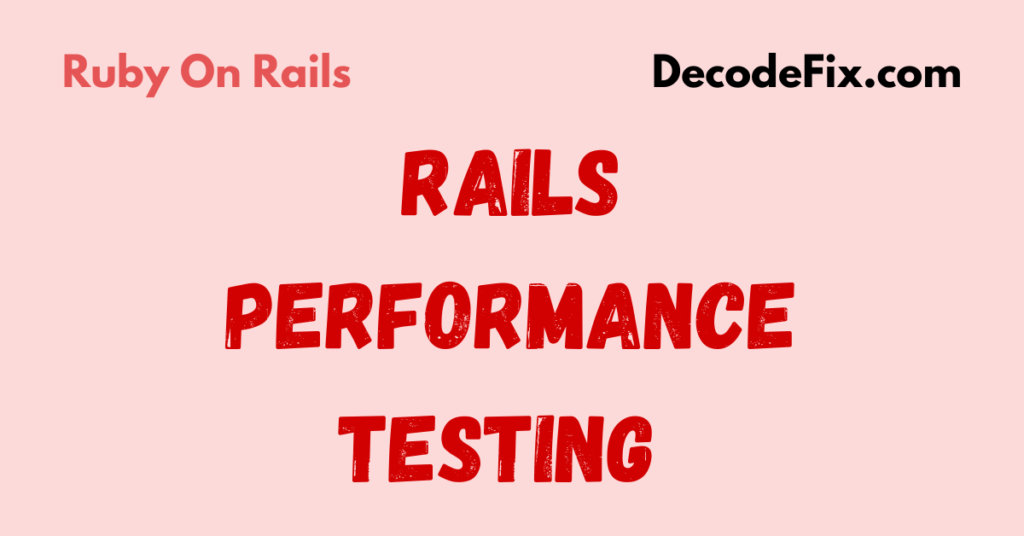
What Is Performance Testing in Rails?
Performance testing evaluates how well your Rails application performs under real-world conditions. Imagine it as testing your car’s speed and endurance before a long road trip—it ensures you won’t break down when it matters most. Similarly, for a Rails app, performance testing helps you gauge its speed, stability, and scalability when handling actual traffic.
If your app takes too long to load or respond, users will get frustrated, leading to a drop in engagement, conversions, or even revenue. This is especially critical for applications dealing with e-commerce, APIs, or high-traffic platforms, where every millisecond counts.
Why Performance Testing Is Vital for Rails Apps
- User Experience: A fast app keeps users happy and engaged.
- Scalability: Ensure your app handles more users as it grows.
- Reliability: Prevent crashes under heavy traffic.
Common Bottlenecks in Rails Apps
- Slow database queries.
- Inefficient API calls.
- Overloaded controllers.
Getting Started with RSpec for Performance Testing
What Is RSpec?
RSpec is a popular testing framework for Ruby that allows developers to write clean, expressive, and readable tests for their applications. It’s widely known for making test-driven development (TDD) accessible and efficient, helping developers ensure their code works as expected.
But here’s the kicker—RSpec isn’t just for functional or unit tests. It’s also a powerful tool for performance testing! By leveraging its flexibility, you can write tests that measure the speed and efficiency of your app’s critical features, such as API endpoints, database queries, and background jobs. This makes RSpec an all-in-one solution for maintaining both the correctness and performance of your Rails applications.
Why Use RSpec for Performance Testing?
- Easy to integrate into Rails projects.
- Flexible syntax for writing custom tests.
- Works well with benchmarking tools.
Setting Up RSpec
- Add RSpec to your Rails project:
bundle add rspec-rails
- Install RSpec:
rails generate rspec:install
- Add performance testing gems like
benchmark-ips
for deeper insights.
Writing Performance Tests in RSpec
Find Critical Performance Areas
Start by identifying slow sections in your app. Look for:
- Long database queries (
N+1
problems). - Slow API responses.
- Heavy controller actions.
Using Tools Like MiniProfiler to Locate Issues Quickly
MiniProfiler is a helpful tool for identifying performance bottlenecks in your Rails app. It provides a detailed breakdown of how long each part of your application takes to process, including database queries, external API calls, and more. By adding MiniProfiler to your app, you can pinpoint areas that need optimization, ensuring your application performs well under heavy usage.
Here’s how you can integrate and use MiniProfiler for performance testing:
- Add the MiniProfiler Gem
First, add themini_profiler
gem to your Gemfile:gem 'rack-mini-profiler'
- Install and Configure
After adding the gem, runbundle install
. Then, add the following to yourconfig/application.rb
to ensure MiniProfiler is enabled in your app:if Rails.env.development? || Rails.env.test?
require 'rack/miniprofiler'
config.middleware.use Rack::MiniProfiler
end
- Using MiniProfiler in Your App
When you run your Rails application, MiniProfiler will automatically track and display performance metrics for each page request. You can click on the profiler to see detailed insights, including time spent on various queries, views, and other processes. This makes it easier to identify performance bottlenecks quickly.
Creating a Basic Performance Test
Once MiniProfiler or similar tools are set up, you can create a basic performance test to check how well your app handles database queries. In Rails, the Benchmark
module helps you measure and display how long specific blocks of code take to execute.
Here’s how you can write a performance test for a database query:
require 'rails_helper'
RSpec.describe 'Performance Test', type: :request do
it 'measures response time for fetching users' do
Benchmark.bm do |bm|
bm.report('Fetch users') do
100.times { User.all.to_a }
end
end
end
end
Explanation:
Benchmark.bm
: This method starts the benchmarking process and prints out how much time each operation takes.bm.report('Fetch users')
: This defines the block of code whose performance you want to measure. In this case, we’re fetching theUser
model 100 times.User.all.to_a
: This query fetches all users from the database. Running it 100 times helps to measure the average performance.
Running this test will display the time taken for fetching users and help you identify if this query is too slow, especially if it’s executed frequently.
Adding Benchmarks for Deeper Insights
While basic performance tests are a good start, deeper insights can help you identify more subtle performance issues. One such approach is using Benchmark.ips
, which measures the number of iterations that can be executed per second. This method is especially useful for measuring how fast individual operations are running, helping you compare different implementations or optimize specific queries.
Here’s an example of how you can use Benchmark.ips
to measure operations per second:
require 'benchmark/ips'
Benchmark.ips do |x|
x.report("Query Users") { User.where(active: true).to_a }
x.compare!
end
Explanation:
Benchmark.ips
: This method measures how many iterations of the block can run per second. It’s ideal for assessing the efficiency of operations that may be run many times in your application.x.report("Query Users")
: This defines the operation you’re testing. In this case, we’re measuring the performance of fetching active users from the database.x.compare!
: After running the test, this will display a comparison of the results, allowing you to see how different operations stack up against each other in terms of speed.
This deeper level of benchmarking helps you measure performance in a more granular way, allowing you to see how different queries or operations impact the overall performance.
Next Steps: Optimizing Based on Results
After running these basic and benchmarked tests, you may identify slow queries or other performance issues. At this point, it’s important to:
- Optimize Database Queries
Look for N+1 query problems or unnecessary database calls and fix them by eager loading associations or rewriting queries to be more efficient. - Optimize Code
Refactor inefficient code that might be causing performance issues. For example, you might identify a complex calculation or an overused loop that can be optimized. - Use Caching
Consider using Rails caching strategies to reduce database load for frequently accessed data. - Simulate Load
Finally, simulate user traffic using tools like JMeter orRack::Attack
to ensure your optimizations hold up under pressure.
By systematically measuring and addressing performance bottlenecks with these tools and techniques, you’ll ensure that your Rails app remains fast and scalable.
Advanced Techniques for Performance Testing
Simulating Load
Want to test under heavy traffic? Simulate multiple users:
RSpec.describe 'Load Test', type: :request do
it 'handles 50 concurrent users' do
threads = []
50.times do
threads << Thread.new { get '/users' }
end
threads.each(&:join)
end
end
Database Performance Testing
Analyze slow queries with tools like pg_stat_statements
for PostgreSQL.
Integrating Tests in CI/CD
Add RSpec performance tests to your CI pipelines. Automate with tools like Jenkins or GitHub Actions to catch performance regressions early.
Real-World Example of Testing a Rails API
When testing an API endpoint in Rails, performance testing becomes crucial to ensure that it responds quickly and handles real-world loads efficiently. Let’s walk through an example of how to test an API endpoint’s response time using RSpec and Benchmark.realtime
.
Step 1: Set Up Your Performance Test
Here’s a simple RSpec test that measures the response time of the /api/v1/users
API endpoint:
RSpec.describe 'API Performance', type: :request do
it 'measures API response time' do
response_time = Benchmark.realtime do
get '/api/v1/users' # Make a GET request to the API endpoint
end
expect(response_time).to be < 0.5 # Ensure response is under 500ms (0.5 seconds)
end
end
Explanation:
Benchmark.realtime
: This method measures how long the block of code inside it takes to execute. In this case, it’s wrapping theget '/api/v1/users'
request, which makes an HTTP GET request to the API.get '/api/v1/users'
: This is the API endpoint you’re testing. It could be any Rails API endpoint, and here we’re assuming that it returns a list of users.expect(response_time).to be < 0.5
: This is the assertion part. It checks if the response time is under 0.5 seconds (500 milliseconds), which is typically a good benchmark for API performance.
Step 2: Analyze the Results
Once you run the test, you’ll receive feedback about how long the request took to process. Here’s how you can interpret the results:
- If the response time is under 500ms, your API is performing well and likely doesn’t need optimization.
- If the response time is over 500ms, you may need to investigate and optimize the API endpoint.
Step 3: Optimize Based on Results
If you find that the API is slow, here are some common optimizations you can consider:
- Optimize Slow Queries:
- Problem: Slow database queries are a common reason for slow API responses.
- Solution: Use indexes on frequently queried columns, rewrite inefficient queries, or optimize ActiveRecord queries to avoid N+1 query problems.
- Example: Adding an index to the
users
table for frequently queried columns likeemail
orcreated_at
can significantly speed up query performance.
add_index :users, :email
- Example: Adding an index to the
- Handle Heavy Payloads:
- Problem: If your API is returning large datasets, the response payload can be too large, leading to long response times.
- Solution: Use pagination to break up the response into smaller chunks, reducing the size of the data being sent at once.
- Cache Frequently Accessed Data:
- Problem: If your API endpoint is requesting the same data repeatedly, it can lead to unnecessary database calls.
- Solution: Use caching strategies like fragment caching or low-level caching in Rails to cache responses that don’t change often.
- Optimize Background Jobs:
- Problem: Some operations in API endpoints may be time-consuming, like sending emails or processing data.
- Solution: Move these operations into background jobs using Sidekiq or ActiveJob so the API can respond more quickly.
- Use HTTP Compression:
- Problem: Large payloads can also be slow to transfer over the network.
- Solution: Enable HTTP compression for API responses to reduce the size of data transferred.
- Example: You can use Rails to enable Gzip compression by adding this to your
config/environments/production.rb
:
config.middleware.use Rack::Deflater
- Example: You can use Rails to enable Gzip compression by adding this to your
Step 4: Rerun the Test
After making optimizations, rerun the performance tests to verify that the changes have improved the response time. For example, if your original response time was over 1 second, after optimizations, you should aim to bring it under 500ms.
Tools to Boost RSpec Performance Testing
Profilers
- MiniProfiler: Visualize bottlenecks in your app.
- rack-mini-profiler: Integrates seamlessly with Rails.
Monitoring Tools
- New Relic: Monitors live app performance.
- Skylight: Pinpoints slow code in production.
Best Practices for Performance Testing
- Write tests for real-world scenarios.
- Focus on critical paths like login and checkout flows.
- Avoid overloading with redundant tests.
Conclusion
Performance testing is crucial for delivering fast and reliable Rails applications. Using RSpec, you can efficiently test your app’s speed, identify bottlenecks, and ensure optimal performance for real-world scenarios. By integrating RSpec into your development process, you not only improve user satisfaction but also prepare your app to scale effectively as your audience grows. Start implementing these strategies today and see measurable improvements in your app’s responsiveness and efficiency.
FAQs on Rails Performance Testing with RSpec
Run them during major updates or before deployment.
RSpec is primarily for backend testing, but it pairs well with tools like Selenium for frontend performance checks.
Unit tests check correctness. Performance tests ensure speed and efficiency.