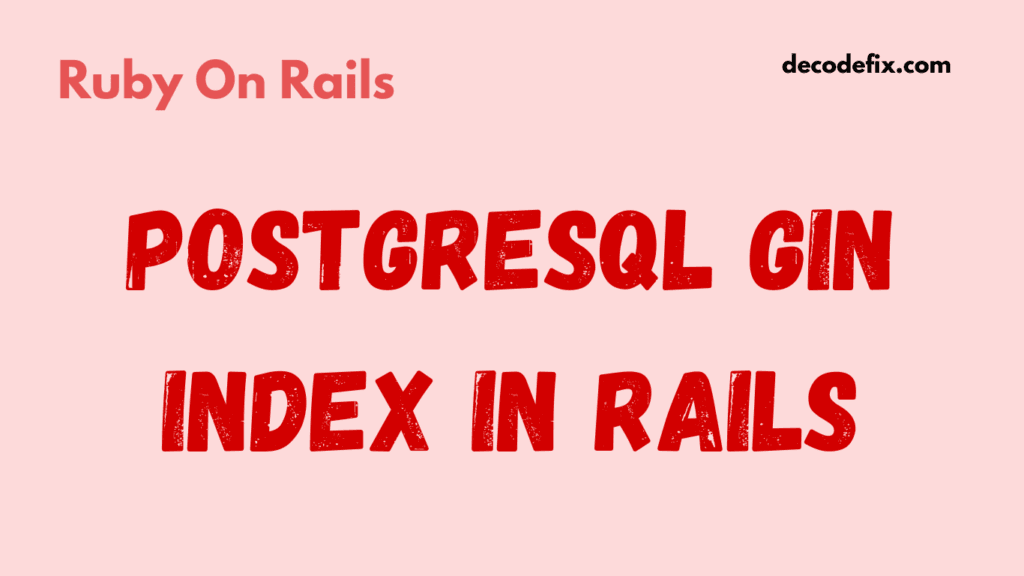
When working with large datasets in Rails, optimizing data retrieval is crucial for performance. Indexes are an essential tool in this process, and GIN (Generalized Inverted Index) is a specialized type that can dramatically speed up searches on certain data types. This blog will cover what a GIN index is, why it’s valuable, how it differs from B-tree indexes, and how to use it effectively in a Rails application.
What is a GIN Index?
A GIN index is a type of index that accelerates searches for complex data types. It is particularly useful for working with arrays, JSONB data, and other multi-valued fields in databases like PostgreSQL. Unlike other index types that work well for single values, GIN indexes efficiently handle data that contains multiple values, making them ideal for searches within arrays or nested JSON fields.
What is an Index in Rails?
In Rails, an index is a database structure that helps optimize data retrieval by creating a separate structure that allows the database to find rows faster without scanning the entire table. By default, Rails will use B-tree indexes for general-purpose indexing.
When you create an index on a column in Rails, you’re effectively telling the database to create a structure that speeds up searches on that column. This is crucial for columns that are frequently queried, like user IDs, email addresses, or other fields used in searches or joins.
Why Use a GIN Index in Rails?
The main advantage of a GIN index is its ability to make queries on large, complex data sets much faster. For instance, if you have a table with a JSONB column that stores nested data (like user preferences or product details), a GIN index can drastically reduce query times. This is useful in real-world scenarios where:
- You need to search within an array, like tags associated with a post.
- You have a JSONB column that stores nested or unstructured data.
- You frequently perform containment queries, such as checking if a JSON object contains specific key-value pairs.
How Does the Gin Index Work in PostgreSQL?
When you create a Gin Index, it doesn’t just store the indexed values directly. Instead, it builds an inverted index where every word or value is mapped to the rows where it appears. This is what makes it so fast, especially when dealing with complex data types.
For example, if you’re storing a list of tags in a column (as an array or JSON), the Gin Index will map each individual tag to the row it belongs to. So, when you query for a specific tag, it can instantly return the relevant rows without needing to scan the entire table.
Here’s an analogy: Imagine a large library. Without an index, you’d need to skim through every book to find a topic. But with an index (a Gin Index), you can look up the topic and immediately find all the books that mention it.
How to Set Up a Gin Index in Rails
Now that you understand the concept of a Gin Index, let’s get to the practical part—how to implement it in your Rails app.
Step 1. Install PostgreSQL with JSONB Support
The Gin Index is mainly used with JSONB or arrays, so you first need to make sure you’re using a PostgreSQL database that supports these features. Rails comes with great built-in support for PostgreSQL, so all you need is to ensure you’re using it.
If you’re starting a new Rails app, simply run:
rails new myapp --database=postgresql
For existing projects, check your database.yml
file to confirm you’re using PostgreSQL.
Step 2. Create a Migration for the Gin Index
In Rails, creating indexes is handled through migrations. Let’s say you’re working with a model Product
that has a tags
column (stored as a JSONB type) and you want to create a Gin Index on that column.
Here’s how you would create the migration:
class AddGinIndexToProductsTags < ActiveRecord::Migration[6.0]
def change
add_index :products, :tags, using: :gin
end
end
In the above example, we’re adding an index to the tags
column in the products
table using the Gin index method.
Step 3. Run the Migration
Once you’ve created the migration, it’s time to run it:
rails db:migrate
This will create the Gin Index in your database. If you ever need to remove it, you can simply create a migration to remove the index.
Step 4. Full-Text Search Example
Suppose your app also requires full-text search across articles or blog posts. You can take advantage of the Gin Index for this use case as well.
Here’s an example of creating a full-text search Gin Index on a content
column:
class AddGinIndexToPostsContent < ActiveRecord::Migration[6.0]
def change
add_index :posts, :content, using: :gin, opclass: :gin_trgm_ops
end
end
In this case, we’re using gin_trgm_ops
for a trigram-based full-text search, which is a more specialized index that helps with fuzzy search queries.
Step 5. Querying with Gin Index
Once the index is in place, you can start benefiting from its speed in your queries. Here’s an example of querying a JSONB column for a specific value:
Product.where("tags @> ?", '{ "color": "blue" }')
In this query, the @>
operator checks if the tags
column contains the specified JSON object. With the Gin Index, this query will run faster as your dataset grows.
For full-text searches, you can do something like this:
Post.where("to_tsvector('english', content) @@ to_tsquery('english', ?)", "rails & performance")
What is the Difference Between the GIN Index and the B-Tree Index?
While B-tree indexes are the default index type in most databases, they aren’t always efficient for complex data types like arrays or JSONB columns. Here’s a comparison of B-tree and GIN indexes to help clarify:
Feature | B-Tree Index | GIN Index |
---|---|---|
Data Type | Optimized for single-value columns (e.g., INT, VARCHAR) | Optimized for multi-valued fields (e.g., ARRAY, JSONB) |
Use Case | Primary keys, foreign keys, single-column searches | Full-text search, array and JSONB containment queries |
Index Size | Generally smaller | Larger than B-tree |
Query Speed | Faster for equality comparisons | Faster for containment queries (e.g., JSONB or array searches) |
Example of GIN vs. B-Tree
If your application often checks if a tags
array contains certain values, a GIN index will perform better. However, if you’re querying by id
or checking a simple condition like name = 'Rails'
, a B-tree index is more appropriate.
How to Decide When to Use GIN Index in Rails?
- Data Type: Use GIN indexes for JSONB, arrays, or full-text fields.
- Query Pattern: If you need to frequently search within multi-valued fields, a GIN index is likely more efficient.
- Performance Requirements: If your application has performance bottlenecks due to complex queries, try adding a GIN index and monitor the improvements.
Real-World Scenarios for GIN Indexes in Rails
- JSONB Columns: Many modern applications store semi-structured data in JSONB fields. For example, storing product details, user preferences, or order metadata in JSONB allows flexibility. A GIN index enables quick searches within these fields.
- Tagging and Categories: For applications that allow users to tag posts, photos, or products, storing tags in an array and indexing them with a GIN index can speed up retrieval when searching by tag.
- E-commerce and Recommendations: If you store user preferences or product attributes in JSONB or arrays, a GIN index can improve the performance of recommendation systems or personalized content.
Pros and Cons of the GIN Index in Rails
Pros:
- High Performance: Optimizes search speed for complex data types.
- Supports Containment Queries: Ideal for
@>
containment operators. - Perfect for JSONB and Array Searches: Speeds up searches within multi-valued fields.
Cons:
- Larger Size: Requires more storage than B-tree indexes.
- Slower Insert and Update Performance: Because of their complexity, GIN indexes can slow down insert and update operations.
When Not to Use Gin Indexes
While Gin Indexes are powerful, they’re not always the right solution. Here are a few scenarios where you might want to reconsider using a Gin Index:
- Small datasets: If your dataset is small and simple, a Gin Index might not offer any significant performance improvement.
- Heavy updates: If the column you’re indexing changes frequently, Gin Indexes can become slow to update, so consider performance trade-offs.
In these cases, you might be better off sticking with a regular B-tree index or another simpler approach.
Best Practices for Using Gin Indexes in Rails
To get the most out of your Gin Index, follow these best practices:
1. Use JSONB or Arrays Wisely
Gin indexes are great for JSONB and arrays. However, keep your JSON structure clean and consistent. Indexing large, nested JSON objects can lead to unnecessary complexity. Use simpler, flatter structures for optimal performance.
2. Combine Gin with Other Indexes
Sometimes, combining a Gin Index with other types of indexes (like a B-tree) can improve performance. For instance, you could create a composite index if your queries filter by multiple columns.
3. Monitor Query Performance
Even with a Gin Index, monitor how your queries perform over time. PostgreSQL’s EXPLAIN command is a great tool to see how your queries are being executed. Use it to verify that the Gin Index is being used as expected.
Here’s an example query to use with EXPLAIN
to check if your Gin Index is being used:
EXPLAIN ANALYZE
SELECT *
FROM products
WHERE tags @> '{"color": "blue"}';
EXPLAIN ANALYZE
: This command tells PostgreSQL to show the query execution plan and actually execute the query to provide real-time performance data.
Sample Output (for a query using the Gin Index):
Seq Scan on products (cost=0.00..431.00 rows=10 width=1000) (actual time=0.043..0.057 rows=10 loops=1)
Filter: (tags @> '{"color": "blue"}'::jsonb)
Rows Removed by Filter: 500
Planning Time: 0.090 ms
Execution Time: 0.093 ms
This output shows how PostgreSQL is executing the query. If the Gin Index is being used properly, you’ll see a reference to the GIN index scan instead of a Seq Scan (sequential scan), indicating that the query is benefiting from the index.
Real-World Example: E-commerce Product Search
Let’s consider an e-commerce platform that has a large inventory of products. Each product has tags, descriptions, and attributes stored as JSONB data. The platform allows users to search products based on multiple filters, including color, size, and category.
By using a Gin Index on the tags
column (which is stored as JSONB), the platform can:
- Quickly filter products by attributes like color or size.
- Speed up the full-text search for product descriptions.
- Handle large amounts of data efficiently as the product catalog grows.
Without a Gin Index, querying through these attributes could take a lot of time, especially when filtering based on multiple values.
Conclusion
In Rails, GIN indexes are an effective tool for improving search performance on complex data types like arrays and JSONB. While they aren’t necessary for every application, they are a valuable option for scenarios involving multi-valued data or containment queries. By understanding when to use GIN indexes versus traditional B-tree indexes, you can make informed choices that keep your Rails app fast and efficient.
Key Takeaways:
- Use GIN indexes for multi-valued data, especially arrays, and JSONB.
- B-tree indexes are better for single-valued columns.
- Optimize your database by carefully selecting indexes based on your query needs.
Whether you’re building an e-commerce platform or a social media app, knowing when and how to apply GIN indexes in Rails can make a substantial difference in performance.