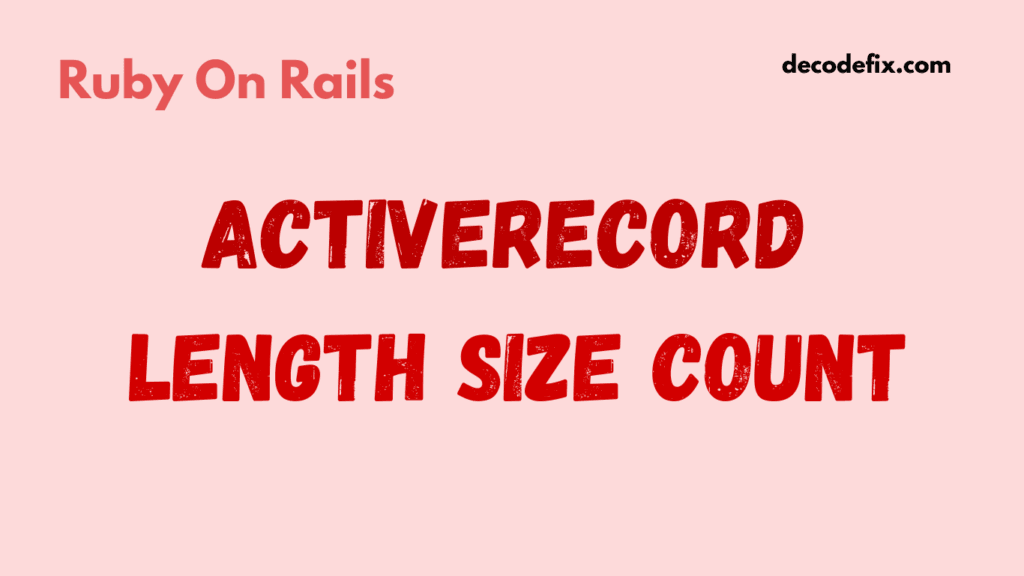
When working with data in Ruby and Rails, developers often need to determine the number of elements within collections, arrays, hashes, strings, and other objects. Ruby offers three primary methods to achieve this: length, size, and count. Though they seem similar, each has unique features and optimal use cases. In this guide, we’ll clarify their distinctions, explore where each is most efficient, and provide practical examples to help you decide which method to use.
Length in Ruby
What is length?
The length
method returns the number of elements in a collection or characters in a string. This method is straightforward and widely used for checking sizes without any conditions.
array = [1, 2, 3, 4, 5]
array.length # Output: 5
string = "Hello, world!"
string.length # Output: 13
When to use Length
The length
method is an excellent choice when you need a quick, simple, and straightforward way to determine the size of an array, string, or other collection without any additional filtering or conditions. Here’s when to opt for length:
- Strings: To get the character count in a string.
- Arrays/Hashes: To determine the total number of elements.
Performance Tip: length
is often a better choice for readability in code, as it’s universally understood and widely used across various coding languages.
Size in Ruby and Rails
What is size?
In Ruby, size behaves similarly to length. You’ll often see size
used interchangeably with length
in Ruby code, especially in arrays, hashes, and strings. However, Rails takes size
a step further by using it with ActiveRecord to optimize database queries.
array = [1, 2, 3, 4, 5]
array.size # Output: 5
string = "Hello, world!"
string.size # Output: 13
Special Use in ActiveRecord
In Rails, size
on an ActiveRecord association checks if data is already loaded in memory. If the data is loaded, it returns the length of the loaded data. If not, it performs an optimized database count query. This makes size
a powerful tool in Rails when working with ActiveRecord associations.
# Assuming User has_many :posts
user = User.find(1)
# Efficient count without loading all posts
user.posts.size # Uses COUNT query if posts are not loaded yet
# Accessing posts after checking size
user.posts.each do |post|
# Work with each post
end
When to Use size:
- Use
size
for ActiveRecord collections to leverage Rails-optimized database querying. - For arrays, hashes, and strings, use
size
as a readable alternative tolength
.
Performance Tip: In Rails, if an association collection is already loaded, size
will avoid additional database calls, making it both faster and more efficient for certain scenarios.
Count in Ruby and Rails
What is the Count?
The count
method counts the elements in an array, hash, or other enumerable collections. With Count, you can specify conditions, allowing it to count only items that meet specific criteria.
array = [1, 2, 3, 4, 5]
# Count all elements
array.count # Output: 5
# Count only elements greater than 3
array.count { |num| num > 3 } # Output: 2
Using count in Rails
In Rails, count
triggers a database COUNT
query if used on ActiveRecord collections that are not loaded into memory. Unlike size
, it always issues a COUNT
query when used with conditions.
# Assuming User has_many :posts
user = User.find(1)
# Triggers a COUNT query on the database
user.posts.count # Efficient COUNT query without loading all posts
# COUNT query with condition
user.posts.count { |post| post.published? }
When to Use Count:
- For Conditions: If you need to count elements based on specific conditions.
- Database Efficiency: To get an accurate count directly from the database, use
count
ActiveRecord associations.
Performance Tip: Using count
with a condition on ActiveRecord without loading the association can save memory and processing time. However, beware that complex conditions may make queries slower depending on the size of your dataset.
Comparing length, size, and count in Rails
Summary Table
Method | Ideal Use Case | Behavior |
---|---|---|
length | Arrays, hashes, strings | Returns total element count |
size | Rails ActiveRecord, general collections | Uses COUNT if unloaded data |
count | Conditional counts, ActiveRecord collections | Uses COUNT with condition |
Key Differences
- Memory Optimization:
size
in Rails avoids loading large datasets by checking if data is already loaded.count
on unloaded ActiveRecord associations directly query the database, keeping memory usage low.
- Flexibility:
count
allows conditional counting, whilelength
andsize
do not.
Practical Examples of length, size, and count in Rails
Here are some common examples where understanding the differences between these methods helps avoid potential performance issues.
Example 1: Counting ActiveRecord Associations
Suppose you have a User
model with a large number of Post
records. If you want the total number of posts, each method performs differently.
user = User.find(1)
# Returns length of posts if already loaded, otherwise triggers a COUNT query
user.posts.size
# Triggers COUNT query on the database directly
user.posts.count
Example 2: Conditional Count on an Array
If you have an array and want to count elements based on a condition, count
is the only option:
array = [1, 2, 3, 4, 5]
# Only counts elements greater than 3
array.count { |num| num > 3 } # Output: 2
Example 3: Using Size with ActiveRecord to Avoid Loading Data
Rails developers frequently encounter large datasets, especially when working with associations. Here’s a scenario where size
shines:
# Let's say we have a huge dataset
user = User.includes(:posts).find(1)
# Efficiently count posts without loading the entire dataset
puts user.posts.size # Triggers COUNT if posts are not already loaded
Conclusion
Choosing the right method depends on your collection type, dataset size, and conditions. Here’s a quick guide:
- Use
length
for simple counts on arrays, strings, and hashes when data is already loaded. - Use
size
in Rails for ActiveRecord collections when data might not be loaded, as it optimizes database queries. - Use
count
for ActiveRecord queries with conditions, as it avoids loading all records into memory.
These three methods can seem interchangeable, but choosing the right one can enhance performance, especially with large datasets in Rails.