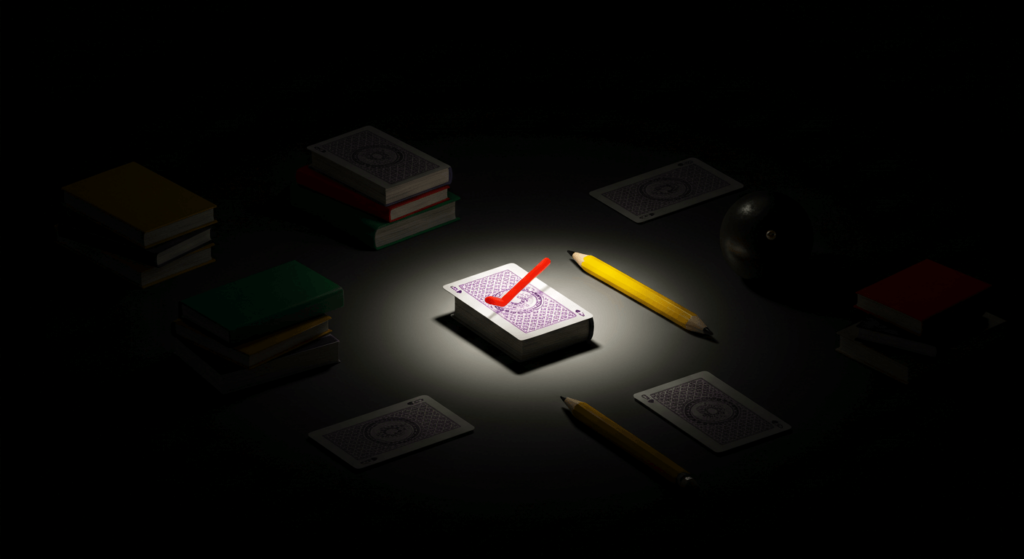
Introduction
Sorting algorithms are a fundamental part of computer science, playing a vital role in organizing data efficiently. From searching databases to optimizing algorithms, sorting is indispensable. Among these algorithms is Selection Sort, a straightforward method that teaches foundational concepts while offering practical applications.
In this guide, we’ll explore Selection Sort, breaking it down into digestible steps, showcasing its key features, and performing a time complexity analysis for best, average, and worst cases. Let’s dive in!
What is the Selection Sort?
Definition
Selection Sort is a simple comparison-based sorting algorithm. It works by repeatedly finding the smallest (or largest, depending on the order) element from the unsorted part of the array and moving it to the sorted part.
Think of arranging a stack of books by height, one at a time—it does something similar!
Steps
Here’s how it operates step by step:
- Start with the first element and consider it the smallest.
- Compare this element with the rest of the array. If a smaller element is found, update the smallest value.
- Swap the smallest element with the current element.
- Move to the next element and repeat the process for the rest of the array.
- Continue until the array is sorted.
Example
Let’s take a small array and sort it:
Array: [29, 10, 14, 37, 13]
- First Pass: Find the smallest element (10) and swap it with the first element (29).
Result: [10, 29, 14, 37, 13] - Second Pass: Find the smallest element in the remaining unsorted array ([29, 14, 37, 13]), which is 13. Swap it with 29.
Result: [10, 13, 14, 37, 29] - Third Pass: The smallest element in [14, 37, 29] is 14. No swap is needed.
Result: [10, 13, 14, 37, 29] - Fourth Pass: The smallest in [37, 29] is 29. Swap with 37.
Final Result: [10, 13, 14, 29, 37]
Key Features of Selection Sort
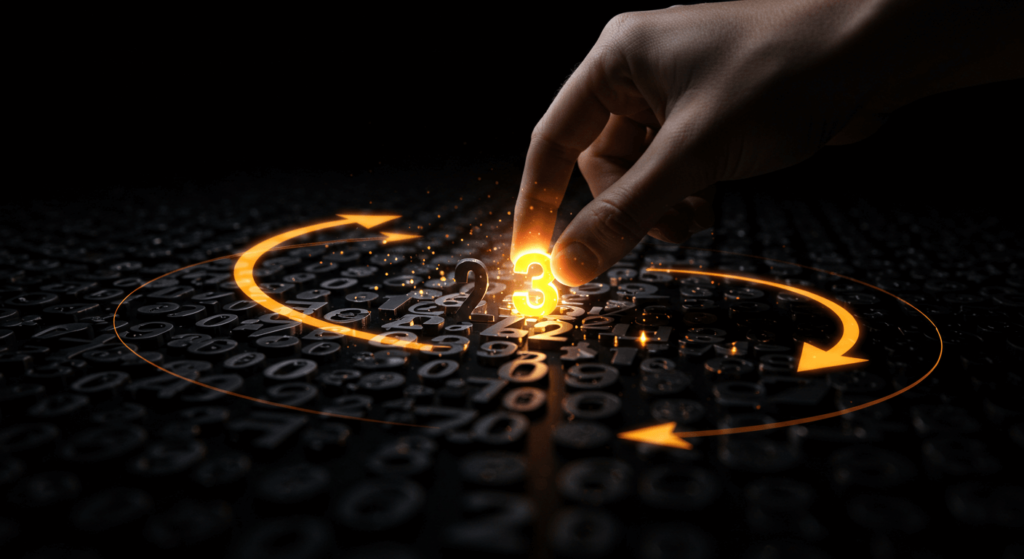
Simplicity
It is incredibly easy to understand and implement. Beginners in computer science often start with this algorithm to grasp sorting fundamentals.
In-Place Algorithm
It doesn’t require extra memory for sorting. The process occurs within the array itself, making it space-efficient.
Best for Small Datasets
It performs well with small datasets where its simplicity outweighs performance concerns. For large datasets, other algorithms like Quick Sort or Merge Sort are better suited.
Pro Tip
While Selection Sort isn’t the fastest algorithm, it’s a great tool for teaching algorithmic thinking. Use it to build a strong foundation in sorting logic before tackling more complex methods.
Time Complexity of Selection Sort
Understanding the time complexity of Selection Sort provides insight into its performance across different scenarios. Regardless of the input arrangement, the algorithm’s complexity remains the same—O(n²). Let’s explore why.
Best Case Time Complexity of Selection Sort: O(n²)
Selection Sort doesn’t optimize for already sorted data. Whether the array is sorted or unsorted, the algorithm always scans the remaining elements to find the smallest one, making its performance identical.
Step-by-Step Example: Best-Case Scenario
Array: [1, 2, 3, 4, 5]
- In the first pass, compare 1 with all other elements. No swaps are needed, but the comparisons still occur.
- In the second pass, compare 2 with the remaining elements. Again, no swaps but comparisons continue.
- Repeat this process until all elements are checked.
Key Insight: Even though no swaps occur, n-1 comparisons are made during the first pass, followed by n-2 comparisons in the second, and so on. This consistent behavior results in a time complexity of O(n²) even for the best-case scenario.
Average Case Time Complexity of Selection Sort: O(n²)
The average case reflects a typical input scenario—neither sorted nor completely reversed. Here, Selection Sort must still compare each element with the rest of the array.
Why Does It Stay O(n²)?
For an array of size n, the algorithm performs:
- n-1 comparisons in the first pass,
- n-2 comparisons in the second pass,
- And so on until just one element remains.
This consistent pattern leads to approximately n²/2 comparisons, simplified to O(n²) in Big-O notation.
Worst Case Time Complexity of Selection Sort: O(n²)
The worst-case scenario occurs when the array is in reverse order. Even here, Selection Sort performs the same number of comparisons as in the best and average cases, making its complexity O(n²).
Step-by-Step Example: Worst-Case Scenario
Array: [5, 4, 3, 2, 1]
- In the first pass, the algorithm identifies 1 as the smallest element and swaps it with 5.
Result: [1, 4, 3, 2, 5] - In the second pass, 2 is identified and swapped with 4.
Result: [1, 2, 3, 4, 5] - The process continues until the array is fully sorted.
Key Insight: Although more swaps occur in the worst case, the number of comparisons remains consistent. Hence, the time complexity does not change.
Space Complexity of Selection Sort
Why is memory efficient?
One of its standout features is its space complexity: O(1). It performs sorting directly on the input array without requiring additional memory for temporary storage.
How Does This Compare to Other Algorithms?
- Merge Sort: Requires additional memory for merging, making its space complexity O(n).
- Quick Sort (in-place version): Requires space for recursive stack calls, which can be as much as O(log n) in the best case.
Practical Implication
The in-place nature makes it ideal for situations where memory is limited, such as embedded systems or small devices. However, its slower speed on large datasets often limits its practical use to smaller arrays.
Pro Tip
If you’re working with large datasets, consider Quick Sort or Merge Sort for faster performance. However, for a quick and memory-efficient sorting task, Selection Sort remains a solid choice.
Comparison with Other Sorting Algorithms
Understanding how it stacks up against other sorting algorithms can help you decide when (or if) to use it. Let’s compare it to Bubble Sort, Insertion Sort, and Merge Sort.
Selection Sort vs. Bubble Sort
Criteria | Selection Sort | Bubble Sort |
---|---|---|
Time Complexity | O(n²) | O(n²) |
Swaps | Minimal (at most n-1) | High |
Memory Usage | In-place (O(1)) | In-place (O(1)) |
Best Use Case | Clear comparisons per pass | Simple but inefficient |
Key Insight: Selection Sort is generally faster than Bubble Sort due to fewer swaps.
Selection Sort vs. Insertion Sort
Criteria | Selection Sort | Insertion Sort |
---|---|---|
Time Complexity | O(n²) | O(n²) (average and worst) |
Best Case | O(n²) | O(n) |
Stability | Not stable | Stable |
Best Use Case | Teaching fundamentals | Nearly sorted arrays |
Key Insight: Insertion Sort is better for nearly sorted data due to its adaptive nature.
Selection Sort vs. Merge Sort
Criteria | Selection Sort | Merge Sort |
---|---|---|
Time Complexity | O(n²) | O(n log n) |
Memory Usage | In-place (O(1)) | Requires extra memory |
Scalability | Poor (slow for large n) | Excellent (fast for large n) |
Key Insight: Merge Sort dominates for large datasets but requires more memory, unlike Selection Sort.
Real-World Use Cases of Selection Sort
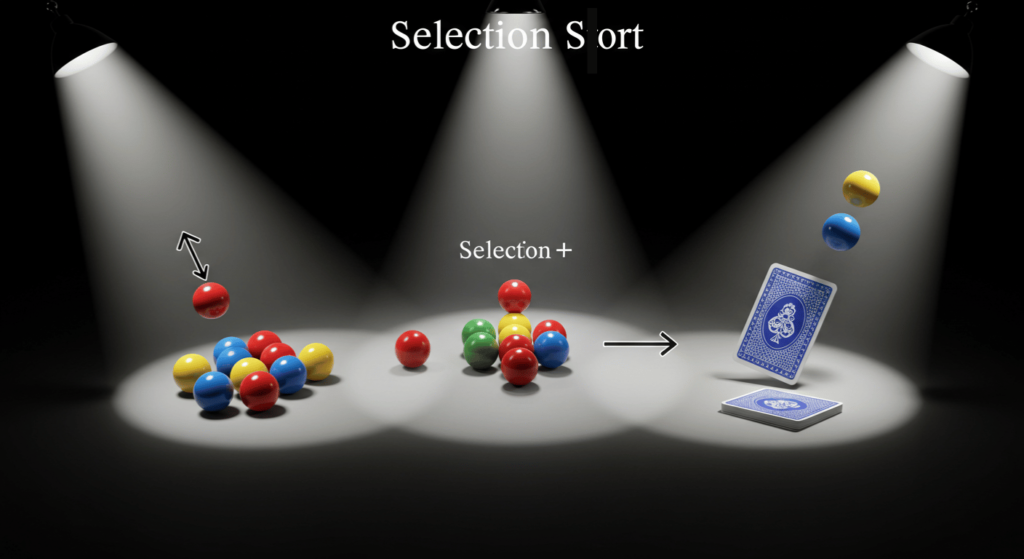
Its simplicity and memory efficiency make it suitable for specific scenarios.
Educational Purposes
It is often used in classrooms to teach fundamental sorting concepts because it’s easy to understand and visualize.
Small Datasets
When working with a small number of elements, its performance is acceptable and its memory efficiency can be a key advantage.
Resource-Constrained Systems
In environments with limited memory, such as embedded systems, the algorithm’s in-place sorting makes it a practical choice.
Code Implementation
Below are code snippets for Selection Sort in C, Python, and JavaScript.
C Program for Selection Sort
#include <stdio.h>
void selectionSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
// Swap the minimum element with the first unsorted element
int temp = arr[minIndex];
arr[minIndex] = arr[i];
arr[i] = temp;
}
}
void printArray(int arr[], int n) {
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
int main() {
int arr[] = {64, 25, 12, 22, 11};
int n = sizeof(arr) / sizeof(arr[0]);
selectionSort(arr, n);
printArray(arr, n);
return 0;
}
Python Program for Selection Sort
def selection_sort(arr):
for i in range(len(arr)):
min_index = i
for j in range(i + 1, len(arr)):
if arr[j] < arr[min_index]:
min_index = j
# Swap the minimum element with the first unsorted element
arr[i], arr[min_index] = arr[min_index], arr[i]
# Example usage
array = [64, 25, 12, 22, 11]
selection_sort(array)
print("Sorted array:", array)
Selection Sort in JavaScript Code
function selectionSort(arr) {
for (let i = 0; i < arr.length - 1; i++) {
let minIndex = i;
for (let j = i + 1; j < arr.length; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
// Swap the minimum element with the first unsorted element
[arr[i], arr[minIndex]] = [arr[minIndex], arr[i]];
}
return arr;
}
// Example usage
const array = [64, 25, 12, 22, 11];
console.log("Sorted array:", selectionSort(array));
These examples highlight how it can be implemented in different programming languages. The consistent logic across languages makes it an excellent starting point for beginners. You can use tools like the Algorithm Visualizer to visualize how Selection Sort compares elements, swaps values, and progresses through the array step by step.
Pseudo Code for Selection Sort
Understanding the pseudo-code of Selection Sort helps clarify its step-by-step logic. This approach is especially useful if you’re transitioning from theory to coding or want to teach the algorithm effectively.
Here’s the pseudo-code:
function selectionSort(array):
for i from 0 to length(array) - 1:
minIndex = i
for j from i + 1 to length(array) - 1:
if array[j] < array[minIndex]:
minIndex = j
swap(array[i], array[minIndex])
return array
Step-by-Step Breakdown
- Outer Loop: Start from the first element and iterate through the entire array.
- Inner Loop: Compare the current element with the remaining unsorted elements.
- Minimum Selection: Identify the smallest element in the unsorted portion.
- Swapping: Swap the smallest element with the first element in the unsorted portion.
- Repeat: Continue until the array is completely sorted.
Example: Sorting [64, 25, 12, 22, 11]
- Initial Array:
[64, 25, 12, 22, 11]
- Step 1: Find the smallest element (
11
) and swap with the first element.
Array after Step 1:[11, 25, 12, 22, 64]
- Step 2: Repeat for the unsorted portion. Find the next smallest (
12
) and swap.
Array after Step 2:[11, 12, 25, 22, 64]
- Continue until the array becomes
[11, 12, 22, 25, 64]
.
Tips to Optimize Selection Sort
While it is a simple algorithm, it is inherently inefficient for larger datasets. However, there are still a few ways you can optimize it, especially when combined with other techniques.
Hybrid Approaches
One approach is to combine it with other algorithms, like Insertion Sort. For example, you could switch to Insertion Sort once the array becomes small enough, where Insertion Sort outperforms Selection Sort. This hybrid approach improves the overall performance of datasets that are small or partially sorted.
Optimizing Swaps
While it minimizes the number of swaps, some variations of it aim to reduce the number of unnecessary swaps by checking whether the current element is already in its correct position before performing a swap. This could slightly improve the algorithm’s performance in specific scenarios.
However, despite these optimizations, it remains inefficient for larger datasets due to its O(n²) time complexity. If you need more speed, consider exploring algorithms like Merge Sort or Quick Sort, which have better performance for large arrays. To understand more about other sorting algorithms, check out our guide on Sorting Algorithms.
Conclusion
We’ve walked through the Selection Sort algorithm, its time complexity, and how it compares to other sorting techniques. We’ve also explored when it makes sense to use it and how it can be optimized in certain cases.
Key Takeaways:
- Selection Sort is simple, easy to understand, and in-place.
- It has O(n²) time complexity for all cases—best, average, and worst.
- It is well-suited for educational purposes and small datasets.
Understanding it helps build a solid foundation in sorting algorithms. By experimenting with it, you gain insight into how algorithms operate under the hood.
If you want to delve deeper into other sorting algorithms and their complexities, make sure to explore our Sorting Algorithms Comparison guide for more advanced insights.
FAQs
The best-case time complexity of the Selection Sort is still O(n²). This is because the algorithm always iterates over the entire array and performs comparisons, even if the array is already sorted. Unlike some other algorithms, such as Insertion Sort, Selection Sort does not take advantage of partially sorted arrays.
Selection Sort is O(n²) for all cases because, regardless of the array’s initial order, the algorithm performs a nested loop: one to iterate through the array and another to find the minimum element in the remaining unsorted section. This results in n(n-1)/2 comparisons, which simplifies to O(n²).
It is suitable when:
– The dataset is small.
– Memory efficiency is a priority (since it’s an in-place algorithm).
– The goal is to teach sorting concepts without needing the fastest solution.
For larger datasets or applications requiring high performance, other algorithms like Quick Sort or Merge Sort are recommended.
Call to Action
We’d love to hear your thoughts. If you have any questions or insights to share, leave them in the comments on Linkedin.
Start experimenting with Selection Sort and share your experiences! We’ll be happy to help if you encounter any challenges.