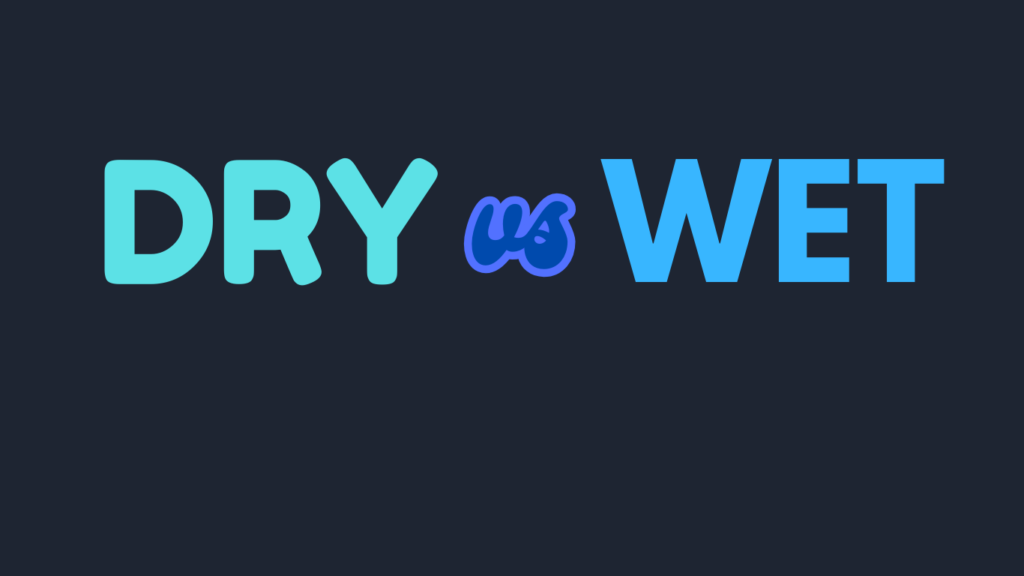
In programming, there is an ongoing discussion about writing DRY (Don’t Repeat Yourself) code versus WET (Write Everything Twice) code. These terms describe two different approaches to software development.
DRY focuses on reducing repetition and making code cleaner and more efficient. WET, on the other hand, emphasizes writing code in a way that may repeat certain elements for clarity or simplicity.
Understanding these principles helps developers write better code. However, applying them is not always straightforward. This guide will explain DRY and WET code, their key features, and the concept of “moist” code. It will also highlight the importance of clean coding practices. Whether you are a beginner or an experienced developer, this information will help you decide which approach works best for your project.
What is a DRY Code?
DRY stands for Don’t Repeat Yourself, a principle coined by Andy Hunt and Dave Thomas in their book The Pragmatic Programmer. The idea behind DRY is simple: minimize duplication in your code. If there’s a piece of code that performs a specific function, you should write it once and reuse it wherever needed. This approach makes code:
- Easier to maintain – Fixing a bug in one place addresses it across all usage.
- More readable – It’s easier to understand the program’s flow with fewer repetitive lines.
- Less error-prone – Reduces the likelihood of inconsistent behaviors caused by different implementations.
Example of DRY Code in C++:
#include <iostream>
double calculate_tax(double price, double tax_rate) {
return price * tax_rate;
}
int main() {
double tax_for_item = calculate_tax(100, 0.2);
std::cout << "Tax for item: " << tax_for_item << std::endl;
return 0;
}
This single function calculate_tax
avoids repeating tax calculation logic multiple times in the code.
What is the WET Code?
On the flip side, WET stands for Write Everything Twice (or in some cases, We Enjoy Typing). WET code does the opposite of DRY by allowing duplication, which can happen due to various reasons:
- Time constraints – Rushing to meet deadlines often means quick fixes and temporary solutions.
- Lack of refactoring – Developers may not revisit older code to consolidate repeated logic.
- Complex requirements – Certain features might need similar but slightly different implementations.
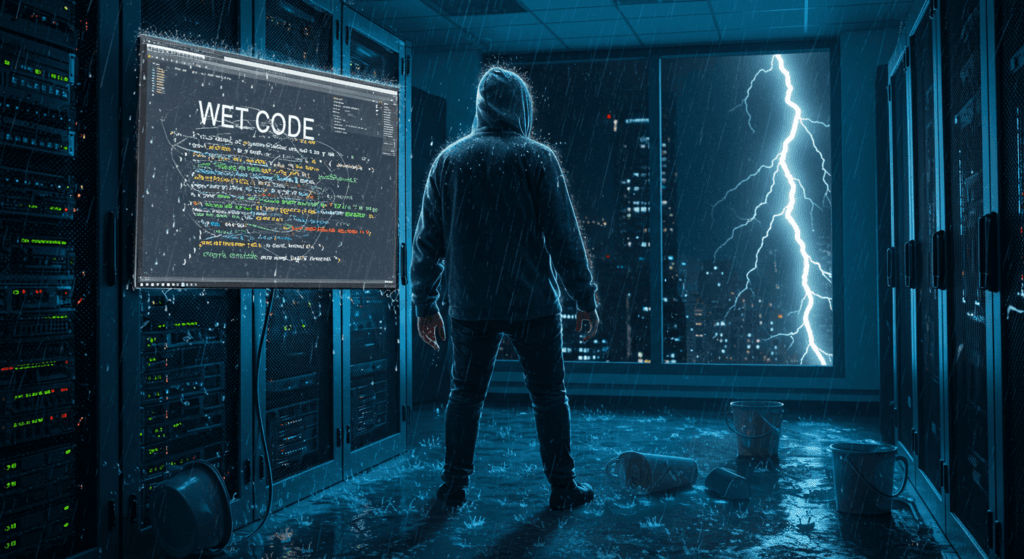
Example of WET Code in C++:
#include <iostream>
double calculate_tax_for_item1(double price) {
return price * 0.2;
}
double calculate_tax_for_item2(double price) {
return price * 0.2;
}
int main() {
double tax_for_item1 = calculate_tax_for_item1(100);
double tax_for_item2 = calculate_tax_for_item2(150);
std::cout << "Tax for item 1: " << tax_for_item1 << std::endl;
std::cout << "Tax for item 2: " << tax_for_item2 << std::endl;
return 0;
}
While these two functions do the same thing, having them as separate methods leads to redundancy and makes the code harder to maintain.
What is the Moist Code?
Moist code is a term used to describe code that lies somewhere between WET and DRY. While it seeks to avoid unnecessary duplication, it’s a bit more flexible than DRY. Moist code may duplicate functionality in cases where strict adherence to DRY would lead to complex abstractions or diminish code readability.
Imagine you have similar code blocks that aren’t identical but share commonalities. Rather than creating highly abstracted code that’s hard to understand, moist code allows for a bit of repetition to keep things straightforward and maintainable.
Key Differences Between DRY and WET Code
DRY and WET code principles are fundamentally different in their approach to coding. Here’s a closer look:
Aspect | DRY Code | WET Code |
---|---|---|
Philosophy | Avoids duplication at all costs | Allows for some level of duplication |
Readability | Can improve readability if done well | Can make code messy and harder to follow |
Maintenance | Easier to maintain | Harder to maintain due to repeated code |
Flexibility | Less flexible, as changes affect all | More flexible in adapting individual parts |
Error Rate | Lower error rate | Higher error rate due to inconsistent logic |
Key Characteristics of DRY Code
DRY code adheres to specific principles that make it more effective for certain types of applications:
- Modular: Code is split into functions or classes, each handling a specific responsibility.
- Reusability: Functions and classes are designed to be reused, improving efficiency.
- Centralization: Common logic is centralized in one location, allowing easy updates.
- Minimal Coupling: DRY code typically has lower dependencies between parts, so each component functions independently.
Pros and Cons of DRY Code
Pros:
- Reduces overall code volume, leading to smaller, faster applications.
- Easy to update, as changes are made in one place.
- Reduces redundancy, making bugs less likely.
Cons:
- DRY code can sometimes lead to over-abstraction.
- Not all problems fit neatly into a DRY structure, so it can make code more confusing if forced.
Key Characteristics of WET Code
WET code, while often criticized, has its own set of characteristics and can be beneficial in specific cases.
- Simplicity: Sometimes, WET code can actually be simpler to read and understand.
- Rapid Prototyping: For quick, one-time projects, it’s often faster to just “get it done” rather than planning reusable functions.
- Flexibility in Changes: Since code sections aren’t tightly bound together, individual parts can be modified without affecting the whole.
Pros and Cons of WET Code
Pros:
- Easier for new developers to understand in simple projects.
- Allows for flexibility and quick adjustments.
Cons:
- Hard to scale due to increased maintenance and potential inconsistencies.
- Can be prone to bugs if similar logic is updated differently across duplicates.
Clean Code Characteristics
Whether you follow DRY, WET, or even moist principles, clean code is always essential. Clean code is defined not just by minimal redundancy, but by clarity, purpose, and readability. Here are some qualities of clean code:
- Readable: Others (and your future self) should be able to understand the code without extensive explanations.
- Organized: Functions, methods, and classes should be well-organized.
- Purposeful: Each line should serve a clear purpose, with no unnecessary elements.
- Consistent Naming: Variable names and function names should be consistent and intuitive.
Frameworks and Libraries That Prefer Each Approach
Some frameworks and libraries are inherently more suited to DRY, while others naturally lead to WET patterns. Let’s explore some of the popular ones and their tendencies.
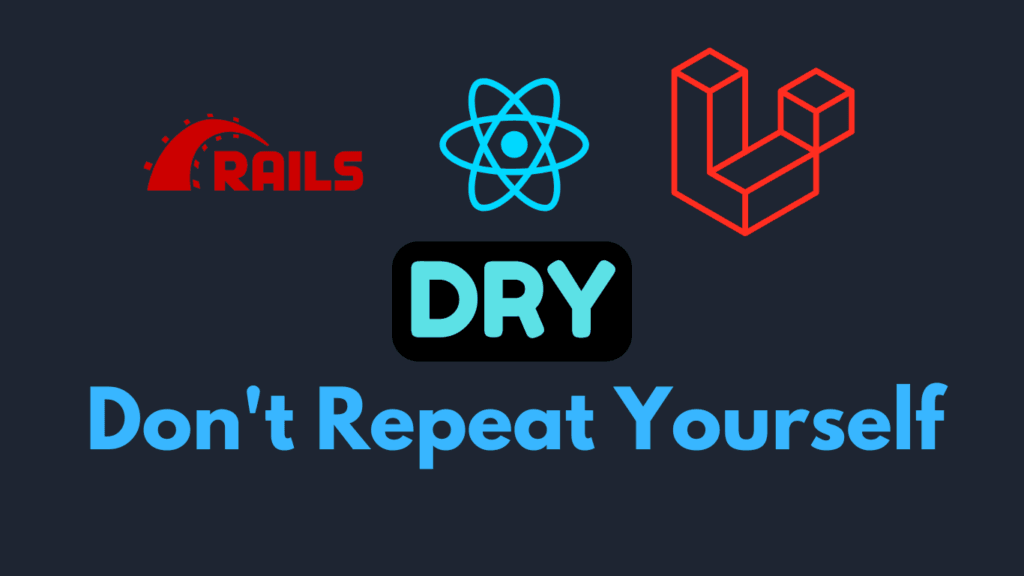
DRY-Friendly Frameworks and Libraries
- Ruby on Rails: Rails encourages DRY with its convention-over-configuration philosophy, offering reusable components like partials, helpers, and shared layouts.
- React with Component Libraries: React favors modular, reusable components that can be placed in different parts of an application.
- Laravel: Laravel’s Eloquent ORM and blade templating offer ways to maintain DRY by leveraging relationships and reusable templates.
These frameworks encourage you to build reusable components and follow modular design, making it easier to adhere to DRY principles.
WET-Prone Frameworks and Libraries
- Vanilla JavaScript Projects: JavaScript can easily become WET in raw or unstructured projects without frameworks to enforce modularity.
- JQuery in Legacy Codebases: Older jQuery-based code tends to be WET, as it often involves repeated code for common DOM manipulations.
- PHP without Frameworks: In PHP without frameworks, you may find yourself duplicating code to handle similar actions due to a lack of built-in structure for reusability.
While these tools don’t necessarily “encourage” WET, the lack of inherent modularity makes it easy for developers to write repetitive code.
When DRY Doesn’t Work
While DRY is usually seen as the gold standard, there are times when sticking strictly to DRY can lead to problems:
- Over-abstraction: Creating reusable code can sometimes lead to overly abstract functions, making the code harder to understand.
- Hidden Dependencies: Overusing DRY can lead to a situation where one piece of code has too many dependencies, creating a “spaghetti code” effect.
- Misplaced Reusability: Not every code block needs to be reused. Sometimes, it’s simpler to write similar code that meets specific needs rather than creating one-size-fits-all functions.
Consider this scenario in a banking application: if you have a function that checks if a user’s account is active, using that same function to verify if a transaction is valid might cause unexpected issues. They seem similar but have different requirements, and enforcing DRY might make things confusing.
The Four Key Concepts of Code Design
1. Modularity: Breaking down a system into smaller parts, each responsible for a specific task. This is closely tied to DRY as it helps avoid repetition.
2. Simplicity: Avoid overly complex structures or logic that can make the code unreadable.
3. Flexibility: Ensuring code can adapt to changes without extensive rewrites.
4. Consistency: Following a coherent pattern across the codebase. Consistency helps other developers understand your approach.
Real-World Examples of WET, DRY, and Moist in Action
Example of DRY: Think of e-commerce platforms like Amazon. With millions of products, DRY principles help reduce redundancy, particularly in code related to product listings, pricing, and discounts.
Example of WET: Consider a small startup developing a prototype. Since the product is likely to change rapidly, developers may use WET code for speed and flexibility.
Example of Moist: A healthcare application might use moist principles for processing patient data, balancing reusability and slight variations for each patient’s unique needs.
Transitioning from WET to DRY Code
Transitioning from WET code to DRY code is a critical step in writing clean, efficient, and maintainable software. It involves a systematic approach to identifying redundancy, consolidating logic, and adopting best practices.
1. Code Review and Refactoring
Importance of Identifying Repetitive Patterns
Code review is essential for identifying areas of redundancy where the same logic or similar code blocks are repeated. These patterns are prime candidates for refactoring to achieve DRY code. Repetition can make code harder to maintain, as updates need to be made in multiple places, increasing the chance of introducing bugs.
Example:
Let’s say you have a program in C++ that calculates areas for rectangles and squares. WET code might look like this:
#include <iostream>
using namespace std;
void calculateRectangleArea(int length, int width) {
cout << "Rectangle Area: " << length * width << endl;
}
void calculateSquareArea(int side) {
cout << "Square Area: " << side * side << endl;
}
int main() {
calculateRectangleArea(5, 10);
calculateSquareArea(4);
return 0;
}
Here, similar logic (multiplying dimensions) is repeated. This can be refactored into a single, reusable function:
#include <iostream>
using namespace std;
void calculateArea(int length, int width = 0) {
int area = (width == 0) ? length * length : length * width;
cout << "Area: " << area << endl;
}
int main() {
calculateArea(5, 10); // Rectangle
calculateArea(4); // Square
return 0;
}
2. Techniques for Consolidating Logic
To transition from WET to DRY, focus on consolidating repetitive logic into reusable components. Here are some effective techniques:
- Use Functions: Abstract repetitive logic into functions with clear inputs and outputs.
- Create Classes: Encapsulate related data and behavior into classes.
- Parameterization: Use parameters to handle variations in repetitive tasks.
- Inheritance and Polymorphism: In object-oriented programming, use inheritance to share common behavior across classes.
Example Using Classes:
#include <iostream>
using namespace std;
class Shape {
public:
virtual void calculateArea() = 0; // Pure virtual function
};
class Rectangle : public Shape {
private:
int length, width;
public:
Rectangle(int l, int w) : length(l), width(w) {}
void calculateArea() override {
cout << "Rectangle Area: " << length * width << endl;
}
};
class Square : public Shape {
private:
int side;
public:
Square(int s) : side(s) {}
void calculateArea() override {
cout << "Square Area: " << side * side << endl;
}
};
int main() {
Shape* rect = new Rectangle(5, 10);
Shape* sq = new Square(4);
rect->calculateArea();
sq->calculateArea();
delete rect;
delete sq;
return 0;
}
When to Choose DRY, WET, or Moist Code
- Large Projects: DRY is typically better for large, long-term projects, as it keeps code manageable.
- Short-term Prototyping: WET might be beneficial if you’re building a quick prototype and don’t expect the code to last.
- Customizable Systems: Moist code can be ideal in applications that require some repetition for readability, especially if customization is a priority.
Conclusion
When it comes to choosing between DRY, WET, and moist principles, the best approach is often to find a balance. Coding is both an art and a science and while best practices are helpful, real-world needs sometimes demand flexibility.
Remember, the goal of any coding principle is to make your code readable, maintainable, and adaptable. So whether you’re aiming for DRY efficiency, WET simplicity, or moist flexibility, focusing on writing clean, clear, and purposeful code will always be the right choice.