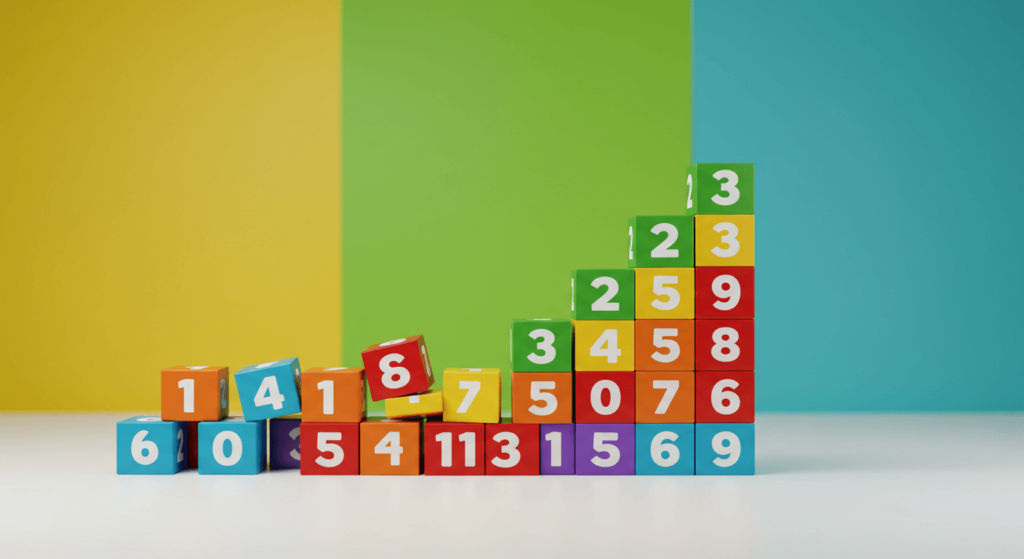
When learning sorting algorithms, Bubble Sort often comes up first. It’s straightforward, easy to understand, and great for teaching the fundamentals of algorithmic thinking. But what about its time complexity? Let’s dive into what makes this algorithm tick (or bubble, in this case).
What Is Bubble Sort?
Bubble Sort is a basic sorting algorithm in which adjacent elements are repeatedly compared and swapped if they are out of order. At the end of each pass, the largest (or smallest) value “bubbles up” to its correct position, making it a simple yet visually intuitive process.
Picture this: when a software engineer explains sorting to a beginner, It is often the first choice due to its ease of implementation. However, its simplicity comes at a cost: inefficiency for larger datasets.
If you’re learning Bubble Sort and want to see it implemented, check out this Algorithm for Bubble Sort in C with practical examples.
Bubble Sort Time Complexity
Time complexity measures the efficiency of an algorithm based on input size. In Bubble Sort:
- Best case (O(n)): The data results are already sorted in one pass, and there are no swaps.
- Average case (O(n²)): The algorithm processes random data with moderate swapping.
- Worst case (O(n²)): Reversed data maximizes the number of swaps and iterations.
In real-world scenarios, such as preparing a dataset for data analysis, this inefficiency can be a bottleneck, making it unsuitable for large-scale sorting tasks. To find alternatives, explore more efficient methods, such as Merge Sort.
Best Case Time Complexity of Bubble Sort
Bubble Sort’s best-case time complexity is O(n). This means that the algorithm requires only a single pass through the dataset to verify that the elements are already sorted. Unlike the average or worst cases, no unnecessary comparisons or swaps occur, making it exceptionally efficient in this scenario.
Take, for instance, a sorted dataset of student grades: [A, B, C, D, F]. It scans the list, comparing adjacent elements, and immediately concludes that no changes are needed. This is where the algorithm’s best-case performance shines.
However, this efficiency is uncommon in practical use cases, as most datasets require some level of reordering. For insights into sorting algorithms optimized for more general cases, you can explore how Insertion Sort handles partially sorted data efficiently.
When Does the Best Case Occur?
The best case occurs when the input array is already sorted. Bubble Sort traverses the array and finds that no elements need swapping. This scenario is ideal and occurs when data preparation, such as cleaning datasets for data analysis, results in an already sorted state.
For instance, consider a scenario where a data scientist runs a report on customer purchase history, and the transactions are pre-ordered by date. Running Bubble Sort in such a scenario requires only one pass, ensuring the algorithm exits early, thanks to an optimization flag that detects no swaps.
Why Is the Best Case Efficient?
The efficiency of Bubble Sort in its best case lies in its early exit mechanism. When no swaps are detected during the first pass, the algorithm terminates immediately, avoiding unnecessary iterations. This feature prevents wasted computational effort and improves performance.
Imagine a real-world analogy: sorting library books that are already arranged alphabetically. A single glance confirms the order, saving you from a time-consuming manual check of each pair.
However, even in the best case, Bubble Sort is rarely the go-to choice for large-scale applications. More efficient algorithms like Merge Sort or Quick Sort outperform Bubble Sort even under optimal conditions. You can dive deeper into alternatives by reading about the Merge Sort Algorithm.
Average Case Time Complexity of Bubble Sort
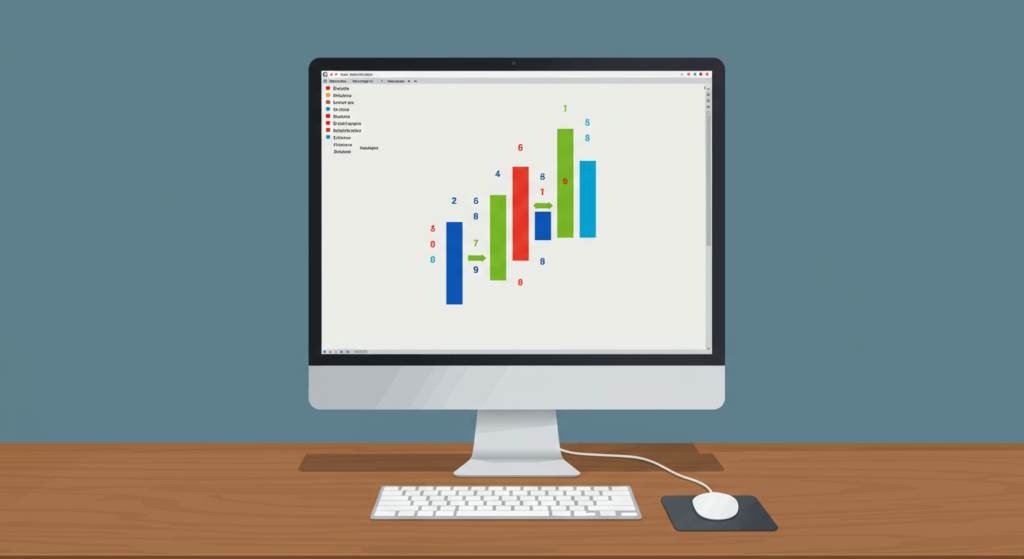
The average case time complexity of Bubble Sort is O(n²). This is the time it takes for the algorithm to complete when the input array is neither sorted nor completely reversed. In these typical scenarios, it must perform several comparisons and swaps, gradually “bubbling” the largest (or smallest) element to its correct position, step by step.
For instance, if you’re sorting a list of user ages in a system where the users’ ages are randomly mixed, Bubble Sort will perform many comparisons and swaps for each element. Each pass ensures the largest unsorted element moves to its correct position, but it takes time proportional to the size of the array—leading to the O(n²) complexity. As a result, it is inefficient for larger datasets, where more advanced algorithms like Quick Sort or Merge Sort might perform better.
What Happens in the Average Case?
In the average case scenario, Bubble Sort works through most of the list, making comparisons and occasionally performing swaps to arrange the elements in their correct order. In other words, it is an everyday situation where the dataset is randomly ordered, and no special optimizations like early exits (as in the best case) can be leveraged.
Think of this as organizing a stack of books by height when they are randomly arranged. For every book, you’d compare it with the next one, swapping them if necessary until the entire stack is ordered. In technical terms, each element must be compared with every other element, leading to O(n²) comparisons and potential swaps.
The behavior is similar to a web application that checks and orders user comments by date in random order. Bubble Sort will compare adjacent comments and continue to swap them until it reach the final, correctly ordered state.
The Role of Randomized Input
Randomized input significantly affects the performance of Bubble Sort. While in the best case, the input is already sorted, and in the worst case the input is in reverse order, in the average case, the input is typically random. This random nature ensures that most of the time, Bubble Sort will need to perform a number of comparisons and swaps, which results in the O(n²) time complexity.
Imagine a case where you’re sorting employee names by age in a database, and the order is randomized. Here, the algorithm will need to go through multiple iterations, repeatedly swapping adjacent employees to bring the younger employees to the front of the list.
Interestingly, randomized inputs contribute to the worst-case scenario (reverse order) for algorithms like Selection Sort, but for Bubble Sort, the randomness keeps the algorithm engaged in multiple iterations, which in turn prolongs the sorting process. For more information, you can explore Selection Sort and how it handles randomized input differently from Bubble Sort.
Worst Case Time Complexity of Bubble Sort
The worst-case time complexity of Bubble Sort is O(n²), occurring when the input is in reverse order. In this scenario, Bubble Sort must compare and swap every adjacent pair of elements until the list is fully sorted. Each pass through the list places the largest unsorted element at the end, but because the array is completely reversed, it will make the maximum number of comparisons and swaps.
To put this in perspective, imagine sorting a deck of cards arranged in the wrong order. You would need to compare every card with its adjacent one and perform swaps for every pair. This results in a significant number of operations, especially as the number of cards increases. The same applies to sorting a large dataset, such as user information in a database, where the worst-case scenario demands a lot of work.
For instance, consider a financial dataset with stock prices. If the data is ordered in reverse, the algorithm will repeatedly compare and swap prices for each pair, leading to a time complexity of O(n²). Therefore, for larger datasets, alternative sorting algorithms like Quick Sort or Merge Sort are often recommended.
When Does the Worst Case Occur?
The worst-case scenario for Bubble Sort occurs when the input list is completely reversed. In this case, every element must be compared with every other element, leading to the highest number of comparisons and swaps. A reverse-ordered dataset forces it to perform the maximum number of operations on each pass, making it inefficient for large datasets.
For a practical example, consider sorting student grade input in descending order by mistake. If the grades are in reverse order, Bubble Sort will compare and swap each adjacent pair until the list is fully sorted. This scenario results in O(n²) comparisons, which is much costlier than algorithms like Merge Sort that can sort this data more efficiently.
As the dataset grows larger, the number of operations increases exponentially, and that’s why Bubble Sort becomes increasingly impractical for real-world applications dealing with large data volumes.
How Does the Number of Comparisons Increase?
The number of comparisons in Bubble Sort increases exponentially as the size of the input grows. In the worst-case scenario, each element needs to be compared with every other element in the list, leading to n(n-1)/2 comparisons, which simplifies O(n²) in terms of time complexity.
For example, if you’re sorting a list of 100 elements, It will need to perform nearly 5,000 comparisons in the worst case. As the input size grows to 1,000 elements, the number of comparisons jumps to nearly 500,000, demonstrating how quickly the number of operations escalates.
This increase in the number of comparisons is one of the primary reasons why Bubble Sort is inefficient for large datasets. For instance, when sorting customer transactions in a large database, Bubble Sort’s number of comparisons can grow unmanageably high, making it unsuitable for production-level systems. In contrast, Merge Sort and Quick Sort optimize the comparison process and are far more suitable for large-scale data handling.
Comparing Bubble Sort to Other Sorting Algorithms
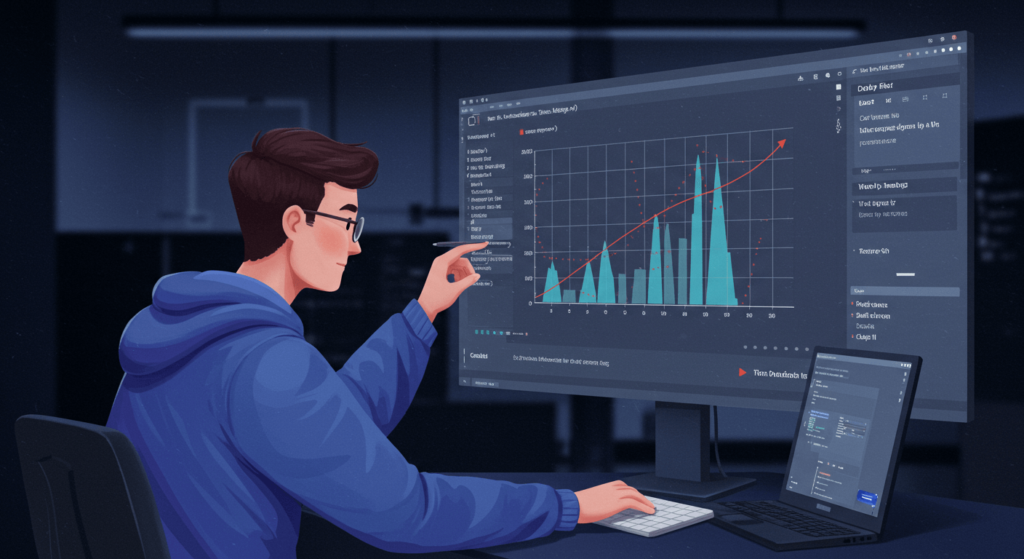
When evaluating Bubble Sort against other sorting algorithms, its simplicity is both its strength and weakness. Unlike Quick Sort or Merge Sort, which have average time complexities of O(n log n), Bubble Sort performs at O(n²) in the worst case, making it inefficient for larger datasets.
Bubble Sort’s simplicity makes it an ideal choice for educational purposes, where the goal is to demonstrate the basic principles of sorting. But in real-world scenarios, especially when handling large datasets, more efficient algorithms like Merge Sort or Quick Sort are generally preferred. For example, when sorting a massive e-commerce product catalog or analyzing big data in fields like healthcare, these algorithms perform much better by reducing the number of operations.
However, It still holds value in small-scale scenarios. For instance, if you are sorting a list of fewer than 10 items in an online contact form or student roster, the overhead of more complex algorithms outweighs their efficiency, making Bubble Sort a reasonable choice.
Ultimately, Bubble Sort is a great starting point for learning algorithms, but when it comes to practical, large-scale applications, you should look toward Quick Sort or Heap Sort for better performance.
Is Bubble Sort Practical?
While Bubble Sort is easy to understand and implement, its practicality becomes questionable when scaling up. The O(n²) time complexity means that as the size of the input grows, the algorithm becomes significantly slower. For instance, imagine you’re working with a customer database containing millions of records. Running Bubble Sort would result in a lot of unnecessary computations, wasting both time and computational resources.
However, it is still useful in specific low-cost systems where computational resources are limited or when sorting data that is already mostly ordered. It can be effective in embedded systems, where the cost of implementing complex algorithms outweighs the performance benefits. For example, when sorting small sensor data arrays in IoT devices, might be more efficient because of its low overhead.
In short, while it is not suitable for high-performance computing, it remains practical in simple applications or when the input size is small enough that O(n²) operations don’t pose a problem. It’s about choosing the right tool for the job.
Scenarios Where Bubble Sort Might Still Be Useful
Though Bubble Sort is not a go-to choice for large datasets, there are certain situations where it might still be useful. These scenarios typically involve small datasets or specific conditions that minimize the inefficiencies of Bubble Sort’s O(n²) time complexity.
One such scenario is when sorting small arrays or lists with a fixed and manageable size. For instance, sorting the top 5 most recent products in an online store’s admin panel requires minimal overhead, making Bubble Sort an ideal option for simplicity and speed in these cases.
Another area where it shines is when the list is nearly sorted. If the data is almost in order, Bubble Sort can often finish faster due to its adaptive behavior. For example, in real-time data feeds where the data is updated and frequently close to sorted, Bubble Sort can complete quickly compared to more complex algorithms that may still analyze the entire list.
Additionally, Bubble Sort could be useful in small embedded systems or legacy software, where upgrading the algorithm may not be necessary or feasible. In cases like these, Bubble Sort is a simple and understandable solution.
While Bubble Sort is outdated in the grand scheme of sorting algorithms, these niche use cases show that, with the right context, it can still be a practical choice.
Space Complexity of Bubble Sort
The space complexity of Bubble Sort is O(1), which means it operates in constant space. This is one of the reasons why it is often considered space-efficient.
Bubble Sort is an in-place sorting algorithm, meaning it doesn’t require any additional storage or memory beyond the input array. It sorts the array by repeatedly swapping adjacent elements, and the only extra memory it uses is for the temporary variable used during the swaps. This temporary variable requires a constant amount of space, regardless of the size of the input array.
In contrast to algorithms like Merge Sort or Quick Sort, which require additional memory for recursion stacks or auxiliary arrays, Bubble Sort does not need to allocate any extra space proportional to the size of the input. Therefore, its space complexity remains constant, O(1).
Imagine you’re sorting a list of items on your phone’s contact list using Bubble Sort. While sorting, it won’t require any extra memory beyond the list of contacts you’re already working with. So, no matter how many contacts you have, the sorting process will still only use a small, constant amount of extra memory.
For more on the performance characteristics of different sorting algorithms, you can check out this Sorting Algorithms Comparison page.
This is particularly beneficial when dealing with small datasets or environments where memory is constrained. However, when it comes to large datasets, Bubble Sort‘s O(n²) time complexity often outweighs its space-efficient nature.
Conclusion
In conclusion, Bubble Sort may not be the most efficient sorting algorithm for large datasets, but its simplicity, ease of implementation, and low resource requirements make it a valuable tool in specific scenarios. Whether used as an educational tool, for small datasets, or in situations where the input is nearly sorted, it still holds a place in the world of sorting algorithms.
However, for high-performance applications or cases where sorting time is crucial, more efficient algorithms like Quick Sort or Merge Sort should be prioritized. As with any algorithm, the key is to understand its strengths and limitations and apply it where it makes the most sense. By recognizing the context in which it is appropriate, you can ensure that your sorting operations are both effective and efficient, no matter the task at hand.
In the end, while Bubble Sort may not be suitable for all scenarios, its simplicity and adaptability ensure that it remains an important concept in the study of algorithms and a useful tool in certain practical applications.