Sorting algorithms are the heart of computer science, and Merge Sort is a killer among the most efficient ones. It’s more of an assembly line: breaking up into parts, sorting them partially, and then putting everything together to have one final, and finished product. But let’s get more in-depth. What’s special about Merge Sort? Why is it so widely used? and algorithm for merge sort in Python. Let’s break it down.
What is Merge Sort?
Merge Sort is a divide and conquer algorithm. Instead of solving one problem, this algorithm solves each piece of the data separately, then merges all in the correct order. This an intelligent strategy if dealing with huge datasets in terms of ensuring performance.
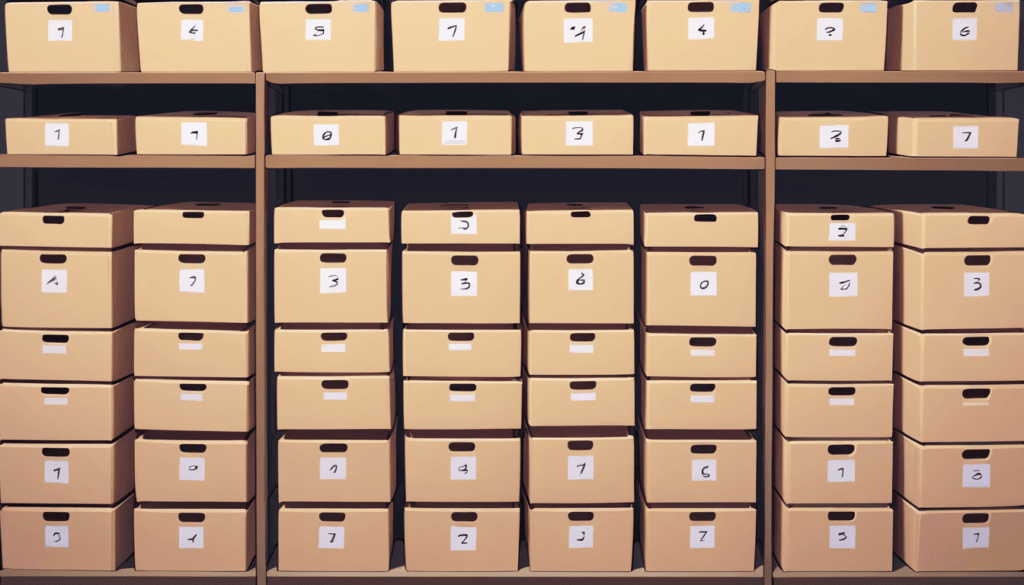
Key Idea:
- Divide the unsorted list into two halves repeatedly until each sublist contains only one element (because a single element is already sorted).
- Conquer each sublist by sorting them.
- Merge the sorted sublists into a single, sorted list.
Where Do We Use Merge Sort?
Now, why should you care? Well, Merge Sort shines in specific use cases:
- Large datasets: When you need to sort huge amounts of data that don’t fit into memory.
- External sorting: It’s commonly used when working with data that must be sorted externally, like in a database or file system.
- Stable sorting: Merge Sort maintains the relative order of records with equal keys. This is important in applications like transaction logs or data streaming.
- Inverted indexes: Many search engines, including Google, utilize algorithms similar to Merge Sort for merging data in inverted indexes.
Frameworks & Libraries Using Merge Sort
Merge Sort isn’t just an academic concept, it’s actually implemented in real-world frameworks and libraries. Some examples include:
- Python’s sorted() function: Under the hood, Python uses an algorithm called Timsort, which is a hybrid of Merge Sort and Insertion Sort. It offers the best of both worlds—Merge Sort’s efficiency for larger datasets, and Insertion Sort’s simplicity for small data chunks.
- NumPy: The popular data science library allows you to specify Merge Sort as the sorting algorithm when using the sort() function. It’s especially useful when dealing with large arrays of data.
The Advantages of Merge Sort
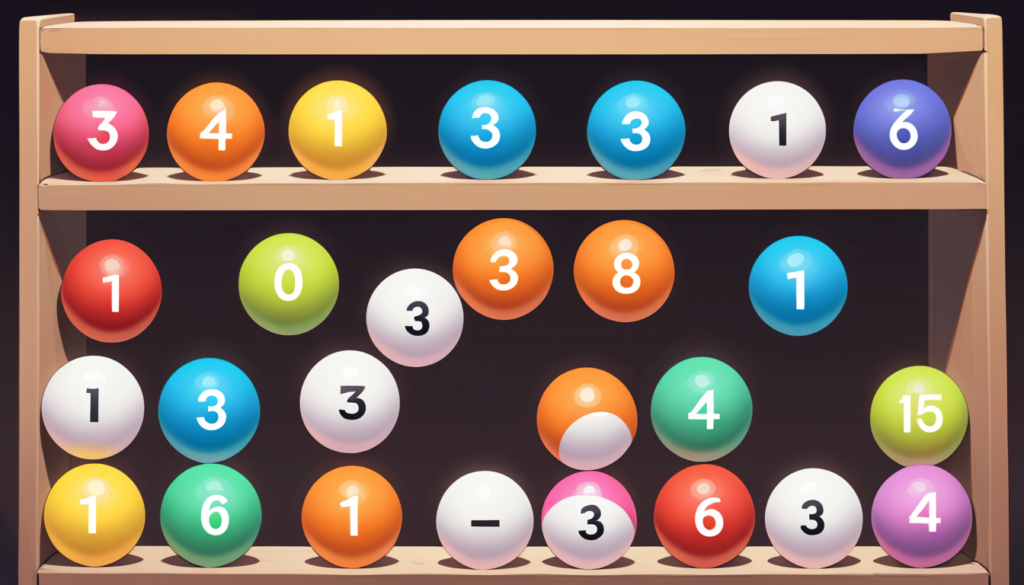
Why use Merge Sort over other algorithms like Quick Sort or Bubble Sort?
- Stable Sorting: This can be essential for preserving the order of equal elements.
- Predictable Time Complexity: Merge Sort consistently runs in O(n log n) time, even in the worst case. This makes it a reliable choice for large datasets.
- External Sorting: When data is too large to fit into memory, Merge Sort is often preferred because it works well with external storage.
The Drawbacks of Merge Sort Algorithm
It’s not all sunshine and rainbows though, Merge Sort has a few limitations:
- Memory Usage: Merge Sort requires O(n) extra space for its merging process, which can be costly for large datasets. That’s why it’s sometimes not the best choice if memory is limited.
- Speed: While Merge Sort has a great worst-case time complexity, algorithms like Quick Sort often outperform Merge Sort in real-world scenarios due to lower constant factors.
Merge Sort Complexity
Let’s get into the details of its performance.
- Time Complexity:
- Best case: O(n log n)
- Average case: O(n log n)
- Worst case: O(n log n)
- No matter what, Merge Sort delivers consistent performance.
- Space Complexity:
Merge Sort requires O(n) auxiliary space due to its recursive splitting and merging process.
Algorithm for Merge Sort in Python
Okay, enough theory. Let’s see what the actual code looks like:
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2 # Finding the mid of the array
L = arr[:mid] # Dividing the elements into 2 halves
R = arr[mid:]
merge_sort(L) # Sorting the first half
merge_sort(R) # Sorting the second half
i = j = k = 0
# Merge the two halves
while i < len(L) and j < len(R):
if L[i] < R[j]:
arr[k] = L[i]
i += 1
else:
arr[k] = R[j]
j += 1
k += 1
# Check if any element was left
while i < len(L):
arr[k] = L[i]
i += 1
k += 1
while j < len(R):
arr[k] = R[j]
j += 1
k += 1
# Example usage
arr = [12, 11, 13, 5, 6, 7]
merge_sort(arr)
print("Sorted array is:", arr)
This Python code splits the input array recursively and merges the sorted halves. The result is a fully sorted array using the merge sort method.
Best Practices for Algorithm of Merge Sort
When implementing Merge Sort, here are a few best practices to keep in mind:
- Avoid unnecessary copying of arrays: In Python, slicing can be memory-intensive. In production systems, you might want to optimize by merging in place or reusing memory where possible.
- Use Timsort if available: Python’s built-in sorting functions (sorted() or list.sort()) use Timsort, which outperforms Merge Sort in real-world scenarios. Unless you’re doing something special, go for Timsort.
- Handle large datasets with care: If memory usage is a concern, consider using an iterative version of Merge Sort or using external sorting techniques.
Divide and Conquer Strategy
At the heart of Merge Sort is the divide and conquer strategy. This powerful approach is not just limited to sorting algorithms; it’s used in various domains such as parallel computing, search algorithms, and problem-solving techniques. It’s a smart way to handle complex problems—break them down into smaller, more manageable sub-problems, solve those, and combine them for the final result.
Conclusion: Merge Sort’s Lasting Impact
In conclusion, Merge Sort remains a valuable algorithm for specific use cases, particularly when dealing with large or external datasets where memory and stability are crucial factors. Although it may not always be the fastest or most space-efficient option in day-to-day programming, its consistency and reliability make it a tool worth having in your algorithmic toolbox.
So, next time you’re dealing with a large dataset that needs sorting—think about Merge Sort. It’s the steady, reliable option that doesn’t get rattled even when the going gets tough.
FAQ
Merge sort is used for sorting large datasets, particularly in external sorting, like handling massive log files or database management.
Merge sort is preferred for its stable sorting and consistent time complexity of O(n log n), making it ideal for large datasets.
Merge sort is best for sorting large, unsorted datasets, especially when stability and consistent performance are required.
Merge sort is faster than selection sort because it uses a more efficient O(n log n) time complexity compared to selection sort’s O(n²).