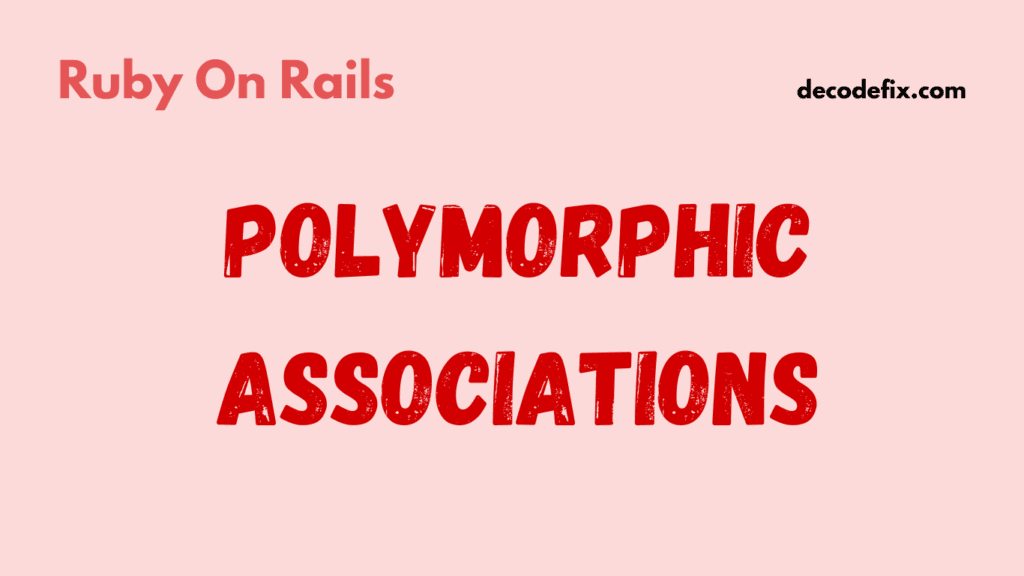
Polymorphic associations in Rails allow a single model to belong to more than one model, offering flexibility in database design. This guide dives into how Rails migrations work with polymorphic associations. We’ll cover basics, practical examples, key methods, and best practices to make working with polymorphic associations in Rails migrations clear, effective, and efficient.
What Are Polymorphic Associations in Rails?
In Rails, a polymorphic association allows a model to belong to multiple other models using a single association. For instance, if you want a Comment
model that can belong to either an Article
or a Photo
, polymorphic associations make that possible.
Here’s how it works in a typical case:
- Polymorphic means “many forms.” The model with the polymorphic association (
Comment
) can be linked to different types of models (Article
orPhoto
) via one association. - Polymorphic relationships require special columns, specifically an ID column and a type column, to identify the model each instance relates to.
Key Benefits of Polymorphic Associations
- Reusability: Allows you to reuse a model across different models without creating multiple tables.
- Flexibility: You can associate a single model with multiple other models, making the design adaptable.
- Simplicity: Reduces the need for extra tables, simplifying the database structure for relationships.
Challenges of Not Using Polymorphic Associations
Data Duplication and Complexity
If you’re not using polymorphic associations, you could end up with a lot of duplicated data. Let’s say you have a Comment
model that belongs to both Post
and Article
. Without polymorphism, you might need two separate foreign key columns in the comments
table: post_id
and article_id
.
But what happens if you need a new model, say Video
? You would have to add yet another foreign key column and modify all of your queries and code to accommodate the new model. This quickly becomes cumbersome as your app scales.
Lack of Flexibility
If your system grows to include many different models that need to be associated with a Comment
model, managing separate relationships for each model can become a headache. You’re essentially re-writing the same logic over and over. This rigid structure stifles flexibility and forces you to repeat the same logic in many places.
Increased Database Schema Complexity
Another issue with not using polymorphic associations is the increasing complexity of your database schema. When you need to store similar data across multiple models, maintaining separate tables for each model’s specific relation can become unwieldy. The complexity grows especially when you want to update or modify these relations.
How Rails Polymorphic Associations Fix Common Issues
Polymorphic associations solve the above issues by allowing a single model to belong to multiple models, using a combination of two fields: a polymorphic_type
and polymorphic_id
. This approach greatly simplifies your database schema and keeps your code DRY (Don’t Repeat Yourself).
Example Setup
Suppose you have a Comment
model that can belong to Post
, Article
, or Image
. Instead of having separate columns for each foreign key, you’d have the following setup in your database:
comments
table:id
(primary key)content
(text of the comment)commentable_type
(string: stores the name of the model this comment belongs to, e.g.,Post
,Article
,Image
)commentable_id
(integer: stores the id of the record this comment belongs to)
Post
,Article
, andImage
models:- All models would use the
has_many :comments, as: :commentable
association.
- All models would use the
With this setup, each comment is linked to a specific model (post, article, or image) via the commentable_type
and commentable_id
fields. This eliminates the need for additional foreign key columns for each model type, making your schema cleaner and more flexible.
Implementation of Polymorphic Association in Rails
Here’s a step-by-step guide to implementing a polymorphic association in Rails.
Step 1: Define the Polymorphic Model
Create a model that will hold the polymorphic association. For example, let’s create a Comment
model that can belong to multiple models, like Post
and Photo
.
rails generate model Comment content:text commentable:references{polymorphic}
This command generates a Comment
model with the following fields:
content
: stores the comment text.commentable_id
andcommentable_type
: store the ID and type of the associated model.
After generating the model, run the migration:
rails db:migrate
Step 2: Set Up the Polymorphic Association in the Comment Model
In the Comment
model, add belongs_to :commentable, polymorphic: true
.
# app/models/comment.rb
class Comment < ApplicationRecord
belongs_to :commentable, polymorphic: true
end
This enables Comment
to associate with multiple models using the commentable
relationship.
Step 3: Add Associations in Related Models
Now, define associations in the models that will have comments. For example, if you want Post
and Photo
to have comments, add has_many :comments, as: :commentable
.
# app/models/post.rb
class Post < ApplicationRecord
has_many :comments, as: :commentable
end
# app/models/photo.rb
class Photo < ApplicationRecord
has_many :comments, as: :commentable
end
This tells Rails that Post
and Photo
models can each have many comments through the commentable
polymorphic association.
Step 4: Create Comments for Different Models
To add comments to a Post
or Photo
, specify the commentable
model directly when creating a comment.
Example usage in Rails Console:
post = Post.create(title: "First Post")
photo = Photo.create(name: "Sunset Photo")
# Creating comments for a Post
post.comments.create(content: "Great post!")
# Creating comments for a Photo
photo.comments.create(content: "Beautiful photo!")
Step 5: Access Comments and Commentable Models
You can access comments from the Post
or Photo
model, or retrieve the commentable
model from a Comment
.
# Get all comments for a specific post
post.comments
# Get the commentable model (e.g., Post or Photo) from a comment
comment = Comment.first
comment.commentable # Returns the associated Post or Photo
Step 6: Query Polymorphic Associations
Rails provides an easy way to query polymorphic associations.
Example: Retrieve all comments for both Post
and Photo
.
Comment.where(commentable_type: "Post")
Comment.where(commentable_type: "Photo")
Polymorphic Associations and Database Table Design
A common question with polymorphic associations is how this impacts the database structure. Polymorphic associations use two key columns:
commentable_type
(string): Stores the name of the associated model.commentable_id
(integer): Stores the ID of the associated model.
These columns are stored in a single table (e.g., comments
) to create a flexible relationship. Because of this, queries that involve polymorphic associations can be more complex than simple foreign key joins, but Rails handles this quite efficiently under the hood.
Testing Polymorphic Associations in the Rails Console
Testing polymorphic associations is pretty straightforward using the Rails console. Here’s how you can test it:
Create Models
Open your Rails console (rails c
), and create a new Post
and Comment
:
post = Post.create(title: "My Post", content: "Some content")
comment = post.comments.create(content: "This is a comment")
Check Associations
Now, you can check the polymorphic association:
comment.commentable # Should return the post object
post.comments # Should return an array with the comment object
Test the Comment for Another Model
Let’s add a comment to an Article
model:
article = Article.create(title: "My Article", content: "Article content")
comment2 = article.comments.create(content: "This is another comment")
Verify the Comment Type
You can check the commentable_type
to ensure it is working as expected:
comment2.commentable_type # Should return 'Article'
Working with Rails Polymorphic Associations
Let’s walk through how you can use polymorphic associations in action:
Creating a Comment on an Article
article = Article.find(1)
comment = article.comments.create(content: "This is a comment on an article.")
Here, article.comments.create
automatically fills in the commentable_id
and commentable_type
fields based on the article
instance.
Creating a Comment on a Photo
photo = Photo.find(1)
comment = photo.comments.create(content: "This is a comment on a photo.")
Similarly, the commentable_id
and commentable_type
fields are set to link the comment with the specific photo
.
Fetching Comments for Any Model
article_comments = article.comments
photo_comments = photo.comments
This way, you can retrieve comments for any model that has a polymorphic association.
Polymorphism Isn’t the Only Option
Polymorphic associations are a powerful tool, but they aren’t the only solution for handling relationships in your app. In some cases, a regular foreign key relationship may make more sense. For example:
- If your models are very distinct and don’t share a lot of behavior, a simple
belongs_to
andhas_many
relationship might be more straightforward. - If you need to ensure strong data integrity (e.g., ensuring that the associated record always exists), non-polymorphic foreign keys might be a better choice.
When to Avoid Using Polymorphic Associations
Despite its advantages, polymorphic associations aren’t always the best solution. Here are some situations where you might avoid using them:
- Performance Concerns: Polymorphic queries can be slower than simple foreign key queries, especially as your database grows. You may find that using simple foreign keys or a join table provides better performance in some cases.
- Complexity in Queries: Polymorphic associations introduce some complexity when writing queries. You may need to use
joins
andincludes
to optimize performance, which could make your code harder to maintain. - Strong Type Safety: If you need to enforce strict data integrity and relationships, polymorphic associations can be tricky. Since the
commentable_type
column is a string, there’s a risk of storing invalid or unexpected values.
Advanced Tips for Rails Polymorphic Migrations
Adding Constraints: Rails doesn’t automatically enforce constraints for polymorphic associations. You can add custom validation in your models to ensure data integrity.
validates :commentable_type, inclusion: { in: %w[Article Photo] }
Creating Indexes: Adding an index to commentable_type
and commentable_id
speeds up queries, especially as your dataset grows.
add_index :comments, [:commentable_type, :commentable_id]
Default Scopes: You can define a default scope in your polymorphic model to filter records based on commentable_type
.
scope :for_articles, -> { where(commentable_type: "Article") }
Using Polymorphic in Queries: Polymorphic associations make querying flexible. For example:
Comment.where(commentable: article).count
The count will give a numeric output of how many comments on articles.
Real-World Example of Using Polymorphic for Notifications
Imagine a Notification
model that could apply to multiple models—Comment
, Like
, or Follow
. Instead of creating a separate notification for each model, you can use a polymorphic association:
# app/models/notification.rb
class Notification < ApplicationRecord
belongs_to :notifiable, polymorphic: true
end
This setup can be beneficial for complex applications, where notifications could relate to various types of interactions.
Migrating from Non-Polymorphic to Polymorphic
If you need to transition an existing non-polymorphic association to a polymorphic one, there are a few extra steps. Here’s a rough outline:
Add New Columns: First, add notifiable_type
and notifiable_id
columns.
add_reference :notifications, :notifiable, polymorphic: true, index: true
Backfill Data: Use a script to populate notifiable_type
and notifiable_id
based on the existing associations.
Notification.update_all(notifiable_type: "Comment", notifiable_id: 1) # Example only
Update Associations: Update your models to use the new polymorphic association.
Clean Up Old Columns: Once confirmed, remove the old foreign keys.
Testing Rails Polymorphic Migrations
To verify that your migrations and polymorphic associations work correctly:
Create Sample Data: Generate test records to ensure they are linked correctly.
Use FactoryBot for Test Setup: If using FactoryBot, you can set up polymorphic factories like this:
factory :comment do
content { "This is a test comment" }
association :commentable, factory: :article
end
Write Tests: Test all CRUD operations for your polymorphic associations. Ensure each linked model functions as expected.
Conclusion: When and Why to Use Polymorphic Associations in Rails
Polymorphic associations in Rails provide a flexible and efficient way to handle relationships between models that share common behavior. By using polymorphism, you can reduce data duplication, simplify your database schema, and make your code more maintainable. However, like any tool, it’s important to know when and where to use it. For situations where you need strong data integrity, performance is a priority, or the relationships are very distinct, it may be better to stick with simpler foreign key associations.
By understanding polymorphism and using it appropriately, you can build more scalable, efficient, and maintainable Rails applications.