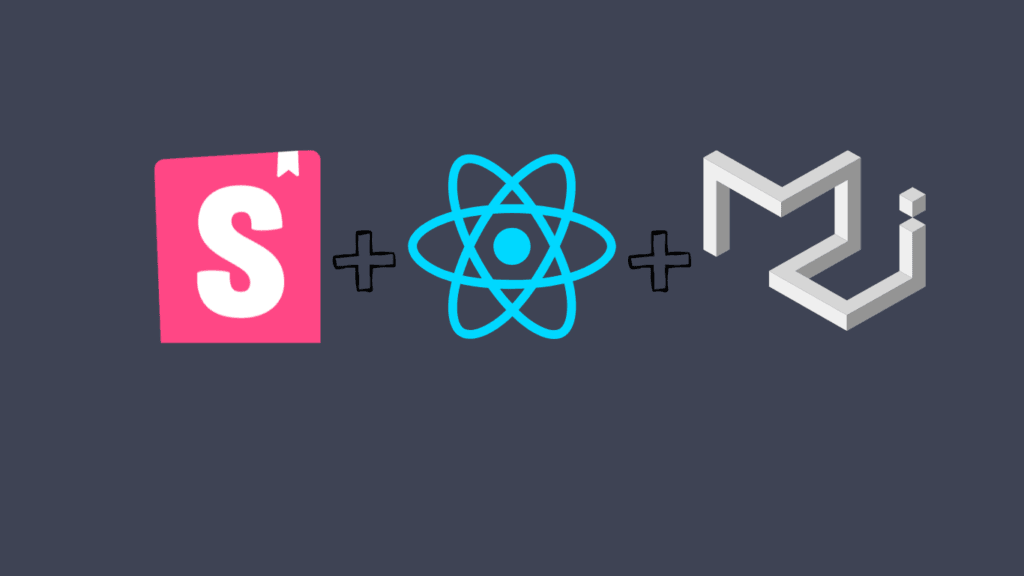
Creating a polished, cohesive UI can be a game-changer, especially in complex apps. Today, many developers are turning to Storybook and Material UI as a way to build, document, and test individual components in isolation before integrating them into a live application. In this guide, I’ll walk you through the core ideas, best practices, and some unexpected insights for building with Storybook and Material UI. Whether you’re an experienced developer or just starting with component libraries, you’ll find a mix of practical advice and in-depth explanations that can help make your work smoother and more scalable.
Introduction: Why Storybook and Material UI?
Storybook has established itself as the go-to tool for creating, viewing, and testing UI components in isolation, making it invaluable for React developers. Paired with Material UI, an adaptable and robust design system based on Google’s Material Design, it creates a streamlined, professional workflow for building visually consistent components.
With Storybook‘s visual documentation and Material UI‘s component-based approach, you’ll be able to:
- Build components independently, which promotes reusability and modularity.
- Ensure a consistent UI across your application.
- Test and refine components in isolation, enhancing efficiency.
In our journey, we’ll explore how to set up a project, use these tools effectively, and build a sample component library that can be scaled up in any large or small project.
Setting Up Storybook with Material UI
1. Project Setup
Start by creating a new React project. We’ll install both Storybook and Material UI.
npx create-react-app storybook-material-ui-example
cd storybook-material-ui-example
npx sb init
Once Storybook is installed, you’ll notice a new .storybook folder that contains the core configuration files.
2. Installing Material UI
Material UI is super easy to set up. Just run:
npm install @mui/material @emotion/react @emotion/styled
And you’re ready to roll! Remember to import the Material UI components you need directly into your component files.
Diving Deeper: Crafting Components in Storybook
What Makes a Good Storybook Component?
A solid component should be versatile and should play nicely with others. Let’s go through a few examples to understand how to structure components for maximum reusability and flexibility.
Example 1: Building a Button
A button is one of the simplest yet most versatile components in any UI library. Here’s how we can create a button that integrates Storybook and Material UI.
In src/components/Button.js:
import React from 'react';
import Button from '@mui/material/Button';
const CustomButton = ({ variant, color, size, label }) => (
<Button variant={variant} color={color} size={size}>
{label}
</Button>
);
export default CustomButton;
In this component, we’re leveraging Material UI’s built-in Button. Notice the props: we’re making the button as flexible as possible by allowing the developer to control aspects like variant, color, and size.
Example 2: Creating a Story for Your Button
Now, let’s create a story file for our button in src/components/Button.stories.js:
import React from 'react';
import CustomButton from './Button';
export default {
title: 'Example/CustomButton',
component: CustomButton,
argTypes: {
color: {
control: { type: 'select', options: ['primary', 'secondary'] },
},
variant: {
control: { type: 'select', options: ['contained', 'outlined'] },
},
size: {
control: { type: 'select', options: ['small', 'medium', 'large'] },
},
},
};
const Template = (args) => <CustomButton {...args} />;
export const Primary = Template.bind({});
Primary.args = {
label: 'Primary Button',
variant: 'contained',
color: 'primary',
};
export const Secondary = Template.bind({});
Secondary.args = {
label: 'Secondary Button',
variant: 'outlined',
color: 'secondary',
};
Storybook makes it easy to add controls that let you switch between different props dynamically. This is a powerful feature when testing the button across multiple states. With controls, you can preview changes in real time, enabling non-developers to explore component behaviors directly within the UI.
Leveraging Material UI Themes in Storybook
One of the standout features of Material UI is theming. By applying a consistent theme, you ensure that all your components look unified and meet branding requirements.
- Setting Up the Theme
In src/theme.js:
import { createTheme } from '@mui/material/styles';
const theme = createTheme({
palette: {
primary: {
main: '#1976d2',
},
secondary: {
main: '#dc004e',
},
},
typography: {
fontFamily: 'Arial, sans-serif',
},
});
export default theme;
export default theme;
- Applying the Theme in Storybook
In .storybook/preview.js:
import { ThemeProvider } from '@mui/material/styles';
import theme from '../src/theme';
export const decorators = [
(Story) => (
<ThemeProvider theme={theme}>
<Story />
</ThemeProvider>
),
];
With this setup, all of your components in Storybook will now follow the theme’s rules. The benefits are significant—you can instantly see how different elements align visually, which saves time when tweaking colors or typography.
Advanced Tips for Building with Storybook and Material UI
Now, let’s dive deeper. Building modular components is one thing, but designing a full-fledged UI library is another. Here are some advanced tips for taking your setup to the next level:
1. Use Storybook Addons for Accessibility and Testing
Storybook has a robust addon ecosystem. For instance, storybook/addon-a11y helps ensure components meet accessibility standards.
npm install @storybook/addon-a11y
Add the addon in .storybook/main.js:
module.exports = {
addons: ['@storybook/addon-a11y'],
};
2. Documenting Components
Good documentation is key. Storybook’s Docs addon can auto-generate documentation from your component and story files.
npm install @storybook/addon-docs
With Docs, you can create a live guide for your components. This is incredibly useful in teams where designers, PMs, and other stakeholders need an up-to-date reference for UI elements.
3. Testing Interactivity with Storybook Controls
Controls are a game-changer because they let you preview component props in real-time. For example, when working with a button component, you might use controls to dynamically alter the text, color, and size, giving you an instant preview of how the button behaves with different configurations.
Real-World Example: Developing a Dashboard with Storybook and Material UI
In a typical development setting, you might be tasked with building an entire dashboard UI. Using Storybook, you can break down the UI into distinct components like:
- Cards for displaying metrics
- Lists for recent activities
- Tables for displaying data
- Forms for user inputs
Building these as isolated components within Storybook allows for easier testing and maintenance. For example, you can use Material UI’s Grid system to layout these components and verify how they align without impacting the larger application.
The Developer’s Perspective: Is Storybook Worth the Hype?
As a developer, I believe that Storybook is an investment in scalability. By focusing on modularity, you create a library of components that you (and your team) can reuse across multiple projects. This approach also helps maintain consistency, an underrated benefit when working with teams or scaling projects.
And, the best part? You don’t need to set up complex environments every time you want to tweak a component; Storybook gives you a sandbox where each component stands alone, making development faster and more fun.
Conclusion: Scaling Up with Storybook and Material UI
Using Storybook with Material UI is more than just a way to streamline development—it’s about creating a design-driven workflow that empowers teams to collaborate more effectively. Once set up, Storybook becomes your UI playground, a place to experiment with new ideas, test edge cases, and maintain your component library with ease.
In the end, Storybook is a game-changer because it shifts your focus to crafting high-quality, reusable components. Material UI provides a robust foundation, and Storybook takes it to the next level by making component development an isolated, interactive, and enjoyable experience.
So, whether you are working on a solo project or in a big team, try incorporating Storybook and Material UI into your workflow. It may take some time to set up initially, but once in place, you’ll likely find that it becomes a core part of your development process. And, with the rapid advancements in both tools, it’s a skill set that’s sure to pay off in the years to come.