If you’re getting ready for a job interview and are new to React, it’s important to get familiar with the React basic interview questions. React, a JavaScript library developed by Facebook has become very popular because of its flexibility and efficiency in creating dynamic user interfaces. When preparing for interviews, the key is to thoroughly understand React basic interview questions. For example, many interviewers may ask about concepts such as JSX, components, and the workings of the virtual DOM. React focuses on building components, managing state, and effectively using hooks. Grasping these foundational concepts will help you succeed in answering most React JS interview questions for freshers.
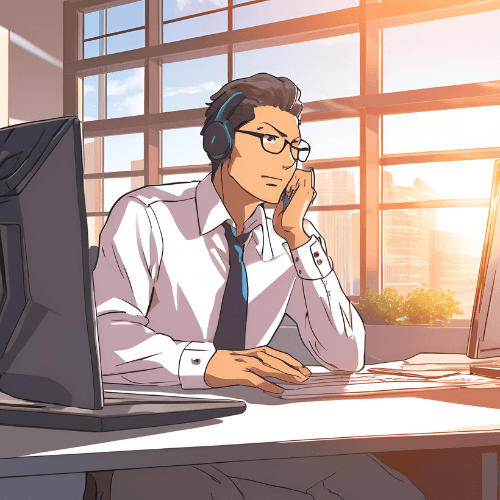
React Concepts for Interviews: What You Need to Know
Interviewers often emphasize specific React concepts for an interview, such as the differences between class and functional components, the workings of useState and useEffect, and the uniqueness of React’s one-way data flow. Mastering these concepts will not only assist you in tackling React JS basic interview questions but also boost your confidence in real-world applications. You can become more confident by giving a react quiz. A common challenge for candidates is explaining the distinction between props and state, so ensure you can articulate this clearly. Some common React interview questions also focus on practical aspects, like optimizing rendering or managing events within React. These topics are particularly important if you’re entering your first job, so consider practicing them in small projects before your interview.
How to Approach React Interview Questions for Freshers
React interviews are not just theoretical; they assess your ability to apply knowledge in real-world situations. When confronted with React JS interview questions for freshers, always try to connect your answers to practical applications. For instance, when discussing lifecycle methods, you can explain how hooks like useEffect
serve as replacements in functional components.
Interview Questions for Freshers
What is React? In what ways is React used?
React is one of the most popular JavaScript libraries for creating dynamic user interfaces. React mostly serves single-page applications. It was created by Facebook and was used to make complex UIs to become easier to handle. With React, a developer can build reusable components, meaning you can break down your application into smaller parts that can be managed. This is one of the greatest benefits of React: it will update and render only the right components whenever your data changes, making it a pretty effective real-time application. Whether you are working on small projects or large applications, this helps to make the scalability and maintenance of your application easier in the case of React’s component-based structure.
What are the main features of React?
React comes with several powerful features that set it apart:
- JSX (JavaScript XML): A syntax that lets you write HTML inside JavaScript.
- Components: Reusable, independent building blocks.
- Virtual DOM: Enhances performance by updating only parts of the actual DOM.
- Unidirectional Data Flow: Data flows in one direction, from parent to child, making debugging simpler.
- Hooks: A way to use state and other React features in functional components.
These features make React a strong choice for building efficient, fast, and scalable applications.
How do you create a component in React?
In React, components can be created either as functional components or class components. A functional component is a JavaScript function that returns JSX:
function MyComponent() {
return <div>Decode Fix!</div>;
}
A class component uses ES6 classes and extends React.Component
:
class MyComponent extends React.Component {
render() {
return <div>Decode Fix!</div>;
}
}
Both types can receive props and manage their own state, though hooks now allow functional components to manage state as well.
What is the difference between state and props in React?
Props are inputs to a component that come from a parent component and are immutable, meaning the receiving component cannot change them. In contrast, state serves as an internal data store for a component, enabling it to keep track of changes over time. Unlike props, state can be modified by the component itself through methods like setState() or hooks such as useState. To put it simply, props are static and managed externally, while state is dynamic and managed internally.
What are lifecycle methods in React?
Lifecycle methods are special methods in class components that allow you to run code at specific points during a component’s life, like when it is created or updated. Key lifecycle methods include:
componentDidMount()
: Runs after the component is mounted.componentDidUpdate()
: Runs after the component has updated.componentWillUnmount()
: Runs right before the component is removed.
These methods are useful for tasks like fetching data, setting up subscriptions, or cleaning up resources.
What is the purpose of keys in React lists?
Keys in React are essential for uniquely identifying elements within a list. When rendering lists of components, React relies on keys to monitor which items have changed, been added, or removed. This mechanism allows React to enhance the rendering process by updating only the components that need it, rather than re-rendering the entire list. It’s important to always use a unique value, like an id or index, as a key when mapping over arrays.
What is the purpose of the useState
hook?
The useState
hook is used in functional components to add and manage state. It returns a state variable and a function to update that state. For example, in a simple counter:
const [count, setCount] = useState(0);
This line creates a count
state variable with an initial value of 0
, and setCount
is used to change that value. Whenever the state is updated, the component re-renders.
How does the useEffect
hook work?
The useEffect
hook allows you to perform side effects in functional components, such as fetching data or interacting with the DOM. It runs after every render by default but can also be configured to run only when certain variables change. For example:
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]);
This effect will only be re-run when count
changes.
What are controlled components in React?
Controlled components are the form input elements that React has control over the values through state. For example, in controlled text input, the value of input is preserved inside the state of that specific component and changes through onChange event. It helps you have full control over the form inputs so it becomes easier to handle validation and dynamic updates.
What are higher-order components (HOCs) in React?
A HOC is a function that accepts a component and returns a new component with added functionality. It is a means to abstract the reuse of component logic. Suppose you want to implement an authentication HOC and share that auth logic across various other components. Simply speaking, an HOC is a way of taking one existing component and enriching it without affecting its underlying structure.
What is the difference between a functional component and a class component?
The function components are lighter and are defined simply as JavaScript functions. They were traditionally stateless, but with hooks like useState and useEffect now they can handle state as well as effects. Class components are ES6 classes that extend React.component and utilize lifecycle methods for handling state. Most applications now use function components because they are lighter weight and use hooks for handling any side effects and states.
What is the use of the create-react-app
command?
create-react-app allows developers to create a new React project from the command line or terminal with an immediately working build configuration, development server, and environment. It avoids having to do many of the configurations that would otherwise be necessary to set up tools like Webpack or Babel so you can code your application as soon as possible.
What is the purpose of React Router in React applications?
React Router is the library through which we control the routing and navigation of an application with a React controller. Using it, developers can define different routes for different parts of the application, creating the possibility of building SPAs in which users navigate from view to view without the page refreshing.
What is conditional rendering in React?
Conditional rendering allows you to render different components or elements according to specific conditions. In React, this can be achieved with the use of conditional operators in JavaScript, if, else, or even the ternary operator. It helps to display the different UI elements based upon the state or props passed to a component.
What is the significance of prop types in React?
Prop types help ensure that props passed to a component will be of the correct type. Using the PropTypes library, you can specify what types of arguments are expected for each prop, helping catch bugs at development time. Example: you can ensure a name prop is a type of string while a count prop should be a number. This kind of validation avoids run-time errors and makes your application more reliable.
If you want to outsource at reasonable prices, feel free to contact us